- What is LCD1602
LCD: Liquid Crystal Display, abbreviated as LCD, its main display principle is to use electric current to stimulate liquid crystal molecules to produce points, lines, and surfaces and cooperate with the back light tube to form a picture. Usually, various liquid crystal displays are directly called liquid crystals.
1602: LCDs are usually named according to the number of rows of characters or the number of rows and columns of the LCD dot matrix. 1602 means that 16 characters can be displayed per line, and a total of two lines can be displayed. There are also similar numbers such as 0801 and 1601.
Note: This type of LCD is a character LCD, which means it can only display ASCII code characters, such as numbers, uppercase and lowercase letters, various symbols, etc.
LCD1602 displays as shown below:
- How to operate LCD1602
Before operation, you need to understand several important knowledge points of LCD1602:
RS: Data/command selection terminal, which controls the command operation and data operation. RS=0 means LCD command operation, and RS=1 means LCD data operation.
RW: Read/write selection terminal. As the name implies, its function is to control reading and writing. RW=0 means writing to the LCD (either instructions or data can be written), and RW=1 means reading from the LCD.
E: Enable signal, a pulse of E represents the start of the operation.
RAM address mapping: The controller has an internal 80B RAM buffer, and the corresponding relationship is shown in the figure. When writing data to any of the addresses 00-0F and 40-4F in the figure (corresponding to 1602, that is, 16 characters can be displayed per line, and a total of two lines can be displayed), the LCD screen can display the data immediately, but when writing to other addresses, they must be moved into the displayable area through the screen shift instruction before they can be displayed.
Data pointer: There is a data address pointer inside the controller, through which the user can access all 80B of RAM (access method: 0X80+corresponding address code).
After saying so much, how to make LCD1602 display characters? Simply speaking, it can be divided into three parts: initialization, instruction operation, and data operation. Next, we will sort out these three parts (mainly based on practical applications, it is impossible to cover everything, please understand).
Initialization: Initialization can be understood as setting the display mode of LCD1602. A set of commonly used initialization instructions are listed below.
void LCD_1602_init()//initialization
{
LCD_1602_Cmd(0X38); //Display: 8 bits per line, 5x7 display dot matrix
LCD_1602_Cmd(0X06); //After writing each character, the pointer increases by one, i.e. the cursor moves one position to the right
LCD_1602_Cmd(0X0c); //Turn on the display but don't display the cursor
LCD_1602_Cmd(0X01); //Clear screen
//LCD_1602_Cmd(0X18): All displayed characters are shifted one position to the left.
//LCD_1602_Cmd(0X80): Set the starting point of the data pointer such as LCD_1602_Cmd(k2+0x80).
}
Command operation: Generally, commands are written to the LCD, so RW=0, RS=0
void LCD_1602_Cmd(uchar cmd) //write command
{
RS=0; //The timing is RS first, then RW and finally E
RW=0;
P0=cmd; //Specific instructions are given to P0, because the eight-bit data port of the general LCD is connected to P0
E=1; //One pulse, one enable signal
dy(2);
E=0;
}
Data operation: write data to LCD, that is, RW=0, RS=1
void LCD_1602_Data(uchar dat) //Write data, write data dat (dat is ASCII code characters, such as numbers, uppercase and lowercase letters, various symbols, etc.)
{
RS=1;
RW=0;
P0=dat; //Specific data for P0
E=1;
dy(2);
E=0;
}
Display location: Display is fine, but where should it be displayed on the LCD? This requires the use of the data pointer mentioned above. The data is displayed at the address where the data pointer points to. We use rows as x and columns as y to represent the specific location.
void LCD_1602_Display(uchar x,uchar y,uchar dat) //Display character dat in row x and column y
{
uchar k1=0x00,k2=0x40;//Comparing the RAM address, the 16 bits displayed in the first line are all 0x0?, and the second line are all 0x4?
if(x==1) //If it is in the first row
{
k1+=y; //At this time, k1 corresponds to the (x,y) address
LCD_1602_Cmd(k1+0x80);//Access location of data pointer
}
else // in the second line
{
k2+=y;
LCD_1602_Cmd(k2+0x80);
}
LCD_1602_Data(dat); //Display the corresponding data on (x,y)
}
Display numbers and single characters: When displaying numbers, because the ASCII code of the number 0 is 48, LCD_1602_Data(6+48); is required. When displaying a single character, single quotes are required LCD_1602_Data('k');, so LCD_1602_Data('6') can also be used to display numbers.
This is enough for the operation of LCD1602.
- Upload code
I used LCD1602 to realize a simple "I love you" display. The code is as follows (I have tested it and it works):
#include "reg51.h"
#define uchar unsigned char
#define uint unsigned int
uchar p[]="i love you";
sbit E=P2^7; // Enable E, send signal in pulse form
sbit RS=P2^6; //0: instruction 1: character
sbit RW=P2^5; //0: write 1: read
void dy(uint x)
{
uint i;
i=x*100;
while(i--);
}
void LCD_1602_Cmd(uchar cmd) //write command
{
RS=0; //The timing is RS, RW, E
RW=0;
P0=cmd;
E=1; //One pulse, one enable signal
dy(2);
E=0;
}
void LCD_1602_Data(uchar dat)//write character
{
RS=1;
RW=0;
P0=dat;
E=1;
dy(2);
E=0;
}
void LCD_1602_init()//initialization
{
LCD_1602_Cmd(0X38); //Display: 8 bits per line, 5x7 display dot matrix
LCD_1602_Cmd(0X06); //After writing each character, the pointer increases by one, i.e. the cursor moves one position to the right
LCD_1602_Cmd(0X0c); //Turn on the display but don't display the cursor
LCD_1602_Cmd(0X01); //Clear screen
//LCD_1602_Cmd(0X18): All displayed characters are shifted one position to the left.
//LCD_1602_Cmd(0X80): Set the starting point of the data pointer such as LCD_1602_Cmd(k2+0x80).
}
void LCD_1602_Display(uchar x,uchar y,uchar dat) //Display character dat in row x and column y
{
uchar k1=0x00,k2=0x40;
if(x==1)
{
k1+=y;
LCD_1602_Cmd(k1+0x80);
}
else
{
k2+=y;
LCD_1602_Cmd(k2+0x80);
}
LCD_1602_Data(dat);
}
void display(uint l,uchar *p) //display string
{
uint i;
for(i=0;i } void main() { uchar i; LCD_1602_init(); //LCD_1602_Display(2,0,6+48); //Add 48 when displaying numbers, because the ascii code of 0 is 48 for(i=0;i LCD_1602_Display(1,i,p[i]); } LCD_1602_Cmd(0X80+0X40); display(sizeof(p)-1,p); }
Previous article:C51 - Small Knowledge Points
Next article:51 single chip microcomputer - use PWM to control the speed of DC motor
Recommended ReadingLatest update time:2024-11-17 01:41
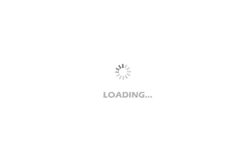
- Popular Resources
- Popular amplifiers
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
stm32+lcd1602 example
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- LED downlight replacement dimmable power supply
- There are some problems with the PFC circuit power-on process. Please consult
- LM358 audio amplifier circuit (loudspeaker)
- CircuitPython OLED Watch/Bracelet
- Research on the process of long and short printed plug products
- [TI recommended course] #[High Precision Laboratory] Magnetic Sensor Technology#
- [National Technology N32G457 Review] V. Soft Switching Power Supply Preparation
- Qorvo Wins Prestigious GTI Award for RF FUSION 5G Chipset Solution
- What diode is in this position?
- FAQ_ S2LP FEC Rules