Use the timer to count time, the annotations are very detailed, and you can change the timing time yourself.
The microcontroller source program is as follows:
#include "reg52.h"
#define GPIO_TRAFFIC P2
#define GPIO_DIG P0
typedef unsigned char u8;
typedef unsigned int u16;
//3-8 decoder pin definition
sbit LSA = P1^0;
sbit LSB = P1^1;
sbit LSC = P1^2;
//Definition of the north-south direction control pins of the traffic light
sbit GREEN10 = P2^0;
sbit RED10 = P2^1;
sbit GREEN11 = P2^2;
sbit YELLOW11 = P2^3;
sbit RED11 = P2^4;
// Traffic light east-west direction control pin definition
sbit GREEN00 = P1^6;
sbit RED00 = P1^7;
sbit GREEN01 = P2^5;
sbit YELLOW01 = P2^6;
sbit RED01 = P2^7;
u8 code smgduan[16] = {0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,
0x6f,0x77,0x7c,0x39,0x5e,0x79,0x71}; //Common cathode
u8 displaydata[8]; //Store the countdown of the signal light
//Define a global variable
u8 second;
//Declaration of various functions
void Timer0Init();
void delay(u16 i);
void Digdisplay();
void trafficpros();
/*******************************
Main function: main
*******************************/
void main(void)
{
second = 1;
Timer0Init(); //Initialize timer T0;
while(1)
{
trafficpros();
}
}
/*******************************
Timer T0 configuration function, only select TR in TCON
0 trigger, timing 10ms.
*******************************/
void Timer0Init()
{
TMOD |= 0x01;
TH0 = 0xd8;
TL0 = 0xf0; //Set the initial value to 55536 and the timing to 10ms
IE = 0x82; // Enable T0 interrupt and total interrupt
TR0 = 1; //Start timer T0
}
/*******************************
Interrupt handling function
*******************************/
void Time0() interrupt 1
{
static u16 i;
TH0 = 0xd8;
TL0 = 0xf0; //Reload initial value
i++; //Record the number of interruptions, 100 times per second
if(100 == i)
{
i = 0;
second++;
}
}
/*******************************
Delay function, delay about 10μs
*******************************/
void delay(u16 i)
{
while(i--);
}
/*******************************
8-bit digital tube bit selection function
*******************************/
void DigDisplay()
{
u8 i;
for(i = 0; i < 8; i++)
{
switch(i)
{
case 0:
LSA = 0; LSB = 0; LSC = 0; break;
case 1:
LSA = 1; LSB = 0; LSC = 0; break;
case 2:
LSA = 0; LSB = 1; LSC = 0; break;
case 3:
LSA = 1; LSB = 1; LSC = 0; break;
case 4:
LSA = 0; LSB = 0; LSC = 1; break;
case 5:
LSA = 1; LSB = 0; LSC = 1; break;
case 6:
LSA = 0; LSB = 1; LSC = 1; break;
case 7:
LSA = 1; LSB = 1; LSC = 1; break;
}
GPIO_DIG = displaydata[i];
delay(100);
GPIO_DIG = 0x00; //disappear
}
}
/*******************************
Traffic light control function
*******************************/
void trafficpros()
{
if(second > 74)
{
second = 1;
}
//North-South traffic and pedestrian paths
if(second < 32)
{
displaydata[0] = 0x00; //The first digit is not displayed
displaydata[1] = 0x00; //The second digital tube does not display
displaydata[2] = smgduan[(31 - second)/10];
displaydata[3] = smgduan[(31 - second)%10];
displaydata[4] = 0x00; //The fifth digit is not displayed
displaydata[5] = 0x00; //The sixth digit is not displayed
displaydata[6] = displaydata[2];
displaydata[7] = displaydata[3];
Digdisplay();
// Each time all the lights are turned off
GPIO_TRAFFIC = 0xff;
GREEN00 = 1;
RED00 = 1;
GREEN10 = 0; //The pedestrian crossing light is green
GREEN11 = 0; //The lane green light is on
RED00 = 0; //The red light on the east-west sidewalk is on
RED01 = 0; //The red light on the east-west lane is on
}
//Intermediate yellow light stage
else if(second < 38)
{
displaydata[0] = 0x00; //The first digit is not displayed
displaydata[1] = 0x00; //The second digital tube does not display
displaydata[2] = smgduan[(37 - second)/10];
displaydata[3] = smgduan[(37 - second)%10];
displaydata[4] = 0x00; //The fifth digit is not displayed
displaydata[5] = 0x00; //The sixth digit is not displayed
displaydata[6] = displaydata[2];
displaydata[7] = displaydata[3];
Digdisplay();
// Each time all the lights are turned off
GPIO_TRAFFIC = 0xff;
GREEN00 = 1;
RED00 = 1;
RED10 = 0; //The red light on the north-south pedestrian crossing is on
YELLOW11 = 0; //The yellow light on the north-south lane is on
RED00 = 0; //The red light on the east-west sidewalk is on
RED01 = 0; //The red light on the east-west lane is on
}
//East-west traffic and pedestrian paths
else if(second < 69)
{
displaydata[0] = 0x00; //The first digit is not displayed
displaydata[1] = 0x00; //The second digital tube does not display
displaydata[2] = smgduan[(68 - second)/10];
displaydata[3] = smgduan[(68 - second)%10];
displaydata[4] = 0x00; //The fifth digit is not displayed
displaydata[5] = 0x00; //The sixth digit is not displayed
displaydata[6] = displaydata[2];
displaydata[7] = displaydata[3];
Digdisplay();
// Each time all the lights are turned off
GPIO_TRAFFIC = 0xff;
GREEN00 = 1;
RED00 = 1;
GREEN00 = 0; //The green light on the east-west sidewalk is on
GREEN01 = 0; //The green light on the east-west lane is on
RED10 = 0; //The red light on the north-south pedestrian crossing is on
RED11 = 0; //The red light on the north-south lane is on
}
//Intermediate yellow light stage
else
{
displaydata[0] = 0x00; //The first digit is not displayed
displaydata[1] = 0x00; //The second digital tube does not display
displaydata[2] = smgduan[(74 - second)/10];
displaydata[3] = smgduan[(74 - second)%10];
displaydata[4] = 0x00; //The fifth digit is not displayed
displaydata[5] = 0x00; //The sixth digit is not displayed
displaydata[6] = displaydata[2];
displaydata[7] = displaydata[3];
Digdisplay();
// Each time all the lights are turned off
GPIO_TRAFFIC = 0xff;
GREEN00 = 1;
RED00 = 1;
RED00 = 0; //The red light on the east-west sidewalk is on
YELLOW01 = 0; //The yellow light on the east-west lane is on
RED10 = 0; //The red light on the north-south pedestrian crossing is on
RED11 = 0; //The red light on the north-south lane is on
}
}
Previous article:Digital altitude meter program based on 51 single chip microcomputer + bmp180
Next article:Design of 51 single chip microcomputer Hall sensor motor speed measurement
Recommended ReadingLatest update time:2024-11-16 12:47
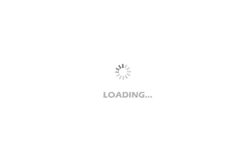
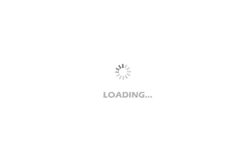
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- STEVAL-IDB0071V1_ by gs001588
- Proteus 8.9 supports STM32F401
- zd test zd test 1
- Help: Why does the pin have voltage output when the PIC microcontroller port is set as input?
- Three important rules about EMC certification
- Micro Python Web Framework: microdot
- EEWORLD University ---- Building Block DAC: System Thinking Method
- Single package six-channel digital isolator and IPM interface reference design for inverter
- [Silicon Labs Development Kit Review] + Building Simplicity Studio Development Environment
- Tiger Wish: Make a wish for 2022, usher in good luck in the new year, and take away the EEWorld New Year gift~