Main program preview:
//This file mainly defines the programmable entry function main, which initializes the system, parameters, and hardware interfaces, and then waits to read serial port data
#include #include #include #include "LPC11xx.h" /* LPC11xx definitions */ #include "uart.h" #include "timer32.h" #include"aw_print.h" #include"aw_init_printHead.h" #include "aw_config.h" #include "aw_font.h" #include"aw_key_led.h" #include "user.h" #include"aw_char_app.h" #include"aw_graph_app.h" #include"aw_barcode_app.h" #include "aw_command.h" #include "pmu.h" // Initialize some global variables in the system void init_Global_Variables(void) { current_char_index=0; DealBuffer_counter=0; memset((low_power_t *)&low_power_Paramter,0,sizeof(low_power_t)); memset((printer_work_Parameter_t *)&printer_work_Parameter,0,sizeof(printer_work_Parameter_t)); printer_work_Parameter.printer_work_model=PRINTER_IDLE; feed_dot_step=0; paper_out_flag=0; head_up_flag=0; clear_buffer(); memset((Key_IRQ_Parameter *)key_IRQ_Parameter,0,KEY_NUM*sizeof(Key_IRQ_Parameter)); } int main(void) { #if UART_DEBUG char buffer[1024]; uint32_t counter,timer; SystemInit(); Init_Sys_Parameter(); init_Global_Varibles(); UARTInit(sys_Parameter.uart_para.baudrate); #ifdef PROJECT_DEBUG printf("nr-- Printer_Project V1.0 --nr"); printf("nr-- Serial Communication test --nr"); #endif counter=0; timer=0; memset(buffer,0,1024); while(1) { if(UartBuffer_read()==1) { timer=0;memcpy(buffer+counter,&DealBuffer,DealBuffer_counter); counter+=DealBuffer_counter; } else { if(timer<10) { timer++; } else if(timer==10) { if(counter!=0) { #ifdef PROJECT_DEBUG printf("%s",buffer); #endif counter=0; memset(buffer,0,1024); timer=11; } } } #elif SPIFLASH_DEBUG uint32_t BaseAdd; uint32_t Address; uint8_t MSB=0xce,LSB=0xa1; uint8_t dot[118]; SystemInit(); spi_flash_init();; while(1) { BaseAdd=BASEADD_GB2312_11X12; if(MSB>=0xA1&&MSB<=0Xa9&&LSB>=0xA1) { Address=((MSB-0xA1)*94+(LSB-0xA1))*24+BaseAdd; } else if(MSB>=0xB0&&MSB<=0xF7&&LSB>=0xA1) { Address=((MSB-0xA1)*94+(LSB-0xA1)+846)*24+BaseAdd; } read_spi_flash(Address,&dot[0],118); /*Get dot matrix*/ LSB++; } #elif PRINTER_HEAD_DEBUG int i,keyval,keyval_bak; uint32_t j; SystemInit(); Init_Sys_Parameter(); init_Global_Variables(); init_Printer_port(); init_key(); /*4. Key initialization*/ while(1) { } #elif TIMER_TEST uint16_t time; uint8_t i; SystemInit(); Init_Sys_Parameter(); init_Global_Variables(); UARTInit(115200); common_timer(1,1000); while(1); #else uint32_t timer=0; /*Used to record the number of times the serial port data cannot be read continuously*/ uint16_t get_dot_count; uint16_t effective_dot,num; /*Number of dots that can be used for printing*/ SystemInit(); Init_Sys_Parameter(); Init_project_Parameter(); init_Global_Variables(); UARTInit(sys_Parameter.uart_para.baudrate); /*1. Initialize the data terminal communication serial port*/ init_Printer_port(); /*2. Initialize the thermal print head interface */ spi_flash_init(); /*3. Font chip communication initialization */ init_key(); /*4, button LED initialization*/ heat_led(); common_timer(1,COMMON_TIME); /*Start the common timer*/ for(timer=0;timer<0xf000;timer++) //delay to ensure that the previous initialization takes effect { ; } init_cutter_position(); /*Let the paper cutter return to the position of the limit switch*/ motor_driver_step40(); /*Let the motor rotate 40 steps in reverse direction*/ Hardware_flow_control(UART_FREE); /* Initialization completed, allow the serial port to receive data*/ timer=0; while(1) { current_char_index=0; if(UartBuffer_read()==1) /*Read serial port data*/ { timer=0; /*When there is data in the serial port, reset this counter*/ while(current_char_index switch(project_Parameter.print_type) /*Process the data read from the serial port*/ { case DATA_TYPE_CHAR_COMMAND: /* character or command */ if(determine_data_command((uint8_t *)(DealBuffer+current_char_index))==0) { if(Save_char()==0) { continue; } if(printer_work_Parameter.printer_work_model==PRINTER_IDLE) /*空闲*/ { if(calculate_get_char_num(&num,&get_dot_count)==1) /*There is a line of data to be printed*/ { start_print(PRINT_CHAR); } } } else /*Command processing*/ { command_deal((uint8_t *)DealBuffer); } break; #ifdef VERTICAL_GRAPH case DATA_TYPE_VERTICAL_GRAPH: /*Vertical modulus graphics*/ if(save_graph()==DATA_ENOUGH) /*After data is retrieved, restore the default data type*/ { project_Parameter.print_type=DATA_TYPE_CHAR_COMMAND; } break; #endif case DATA_TYPE_HORIZONTAL_GRAPH: /*Horizontal modulus graphics*/ if(save_graph()==DATA_ENOUGH) /*Data is retrieved*/ { graph_data.data_finish=1; project_Parameter.print_type=DATA_TYPE_CHAR_COMMAND; } /*When idle, and there is more than one line of data to be printed*/ if((printer_work_Parameter.printer_work_model==PRINTER_IDLE)&&(graph_data.buffer_counter/graph_data.horizontal_mode.horizontal_byteCount>0)) { start_print(PRINT_HORIZONTAL_GRAPH); } else if((printer_work_Parameter.printer_pause==1)&&(printer_work_Parameter.printer_work_model==PRINT_HORIZONTAL_GRAPH)) { /*Resume printing from pause state*/ /*End of data receiving*/ if((graph_data.data_finish==1)||((graph_data.buffer_counter-graph_data.printed_byteCount)/graph_data.horizontal_mode.horizontal_byteCount>0)) {/*There is at least one full row of data*/ Resume_Print_from_pause(printer_work_Parameter.printer_work_model); } } else if((graph_data.data_finish==1)&&(printer_work_Parameter.printer_work_model!=PRINT_HORIZONTAL_GRAPH)) { /*The image data has been received, and the printer is still doing other work, cancel this image printing*/ memset((graph_data_t *)&graph_data,0,sizeof(graph_data_t)); } break; //case DATA_TYPE_BARCODE: /*Barcode graphic*/ // num=save_barcode((uint8_t *)(DealBuffer+current_char_index)); //if(num==DATA_ENOUGH) //{ // save_barcode_parity_bit(); /*Start printing after data is retrieved*/ // while(printer_work_Parameter.printer_work_model!=PRINTER_IDLE) // { // ; //} /*The barcode is out of the printing range and will not be printed*/ //if(project_Parameter.cursor_position==0) /*This row is still empty*/ //{ // effective_dot=MAX_DOT_PERLINE-project_Parameter.print_position-project_Parameter.Limited_width_left_para-project_Parameter.Limited_width_right_para; //} //else //{ // effective_dot=MAX_DOT_PERLINE-project_Parameter.Limited_width_right_para-project_Parameter.cursor_position; //} //if(get_barcode_dot_width()<=effective_dot) //{ // start_print(PRINT_BARCODE); //} //else //{ // memset((barcode_data_t *)&barcode_data,0,sizeof(barcode_data_t)); //} //project_Parameter.print_type=DATA_TYPE_CHAR_COMMAND; //} //else if(num==DATA_ERROR) /*Barcode data error*/ //{ // goto barcode_error; //} //current_char_index++; //break; } } } else /*No data in the serial port*/ { if(timer<5) { timer++; continue; } switch(project_Parameter.print_type) { case DATA_TYPE_CHAR_COMMAND: if((printer_work_Parameter.printer_work_model==PRINTER_IDLE)&&(char_data.printed_byteCount start_print(PRINT_CHAR); } break; #ifdef VERTICAL_GRAPH case DATA_TYPE_VERTICAL_GRAPH: /*Vertical modulus graphics*/ project_Parameter.print_type=DATA_TYPE_CHAR_COMMAND; /*Discard the original data*/ memset((graph_data_t *)&graph_data,0,sizeof(graph_data_t)); break; #endif case DATA_TYPE_HORIZONTAL_GRAPH: project_Parameter.print_type=DATA_TYPE_CHAR_COMMAND; /*No data can be read for three times, it is considered finished*/ graph_data.data_finish=1; if((printer_work_Parameter.printer_pause==1)&&(printer_work_Parameter.printer_work_model==PRINT_HORIZONTAL_GRAPH)) /*Pause during image printing*/ { Resume_Print_from_pause(printer_work_Parameter.printer_work_model); } break; case DATA_TYPE_BARCODE: barcode_error: project_Parameter.print_type=DATA_TYPE_CHAR_COMMAND; memset((barcode_data_t *)&barcode_data,0,sizeof(barcode_data_t)); break; default: break; } } } #endif }
Previous article:Advice for college students on learning ARM and FPGA
Next article:How to port uCOS-II to LPC17XX
Recommended ReadingLatest update time:2024-11-16 15:04
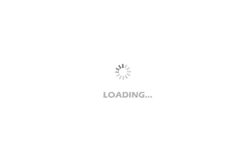
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- MSP430 MCU Development Record (12)
- EEWORLD University Hall----Choosing the latest boost converter and Class D amplifier from Texas Instruments to significantly increase the battery life of the trolley speakers
- Award-winning live broadcast | TI Embedded Live Broadcast Month is waiting for you [Low-power Wi-Fi MCU, Sitara AM57X platform, machine learning]
- SPI settings for msp430f149
- Please recommend a board
- Streamlining mobile phone system design
- EEWORLD University - High-Speed Transimpedence Amplifier Design Process
- PCB
- Please help analyze this statement IS_RCC_APB2_PERIPH(PERIPH)
- At the age of thirty, I am penniless and have nothing to lose. I have to start all over again!!!