I have been working on a low-power device for several days, but the program has been stuck in one place (see the picture below). Today I finally found out where the problem lies. I will summarize the problems of standby wakeup (only for the problems I encountered, the other parts are available on the Internet, based on stm32f103)
1. Solve the problems I encountered
My RTC initialization part has a "judgment of whether the RTC flag stored in the backup register has been configured". If it has been configured, it will enter the else part, but this else part does not have the configuration statements "to enable the power clock, enable the backup clock, and cancel the write protection of the backup area". After the standby wakes up, the program will execute from the main function and will execute to the else part. Because there are no such configuration statements, the alarm clock will not be assigned again and it will be stuck there. (Those configuration statements are in the clock_ini function, with comments)
2. Whether to add extiline17 event in standby mode
If the service program of the alarm interrupt is placed in void RTC_IRQHandler(void) for processing, the extiline17 event is not required to wake up (tested). If the service program of the alarm interrupt is placed in void RTCAlarm_IRQHandler(void) for processing, the extiline17 event is required
3. RTCAlarm_IRQn and RTC_IRQn priority
I saw on the Internet that the priority of RTCAlarm_IRQn should be set higher than that of RTC_IRQn, but if the service program of the alarm interrupt is placed in void RTC_IRQHandler(void), it is not necessary to set it this way. If the service program of the alarm interrupt is placed in void RTCAlarm_IRQHandler(void), it is necessary to set the priority. It is best to solve the priority problem according to the situation.
4. Here are some of my codes
void Clock_ini(void)
{
if(BKP_ReadBackupRegister(BKP_DR1) != 0xA5A5) //Judge whether the RTC flag stored in the backup register has been configured
{
printf("\r\n\n RTC not yet configured....");
RTC_Configuration(); //RTC initialization
printf("\r\n RTC configured....");
Time_Adjust(); //Set RTC clock parameters
BKP_WriteBackupRegister(BKP_DR1, 0xA5A5); //After RTC is set, write the configured flag to the backup data register
}
else
{
if(RCC_GetFlagStatus(RCC_FLAG_PORRST) != RESET) //Check whether power is off and restarted
{
printf("\r\n\n Power On Reset occurred....");
}
else if(RCC_GetFlagStatus(RCC_FLAG_PINRST) != RESET) //Check if reset is set
{
printf("\r\n\n External Reset occurred....");
}
printf("\r\n No need to configure RTC....");
/***Newly added, test, after waking up from standby, the program does not go through the above if part, so there are no these three steps (two statements), so the program will get stuck, so add it, sure enough***/
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR | RCC_APB1Periph_BKP, ENABLE);
/* Allow access to BKP area */
PWR_BackupAccessCmd(ENABLE);
RTC_WaitForSynchro(); //Wait for the RTC register to be synchronized
RTC_ITConfig(RTC_IT_SEC, ENABLE); //Enable second interrupt
RTC_WaitForLastTask();
RTC_ITConfig(RTC_IT_ALR, ENABLE); //naozhong
RTC_WaitForLastTask(); //Wait for writing to complete
}
RCC_ClearFlag(); //Clear reset flag
void NVIC_Configuration(void)
{
NVIC_InitTypeDef NVIC_InitStructure;
EXTI_InitTypeDef EXTI_InitStructure;
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_1);
/* Enable the RTC Interrupt */
NVIC_InitStructure.NVIC_IRQChannel = RTC_IRQn; //Configure external interrupt source (second interrupt)
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 7;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
/* Enable the RTC Alarm Interrupt */
NVIC_InitStructure.NVIC_IRQChannel = RTCAlarm_IRQn; //Configure external interrupt source (alarm interrupt)
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 2;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
//The alarm interrupt is connected to the 17th line external interrupt
EXTI_ClearITPendingBit(EXTI_Line17);
EXTI_InitStructure.EXTI_Line = EXTI_Line17;
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Rising;
EXTI_InitStructure.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStructure);
}
void RTC_Configuration(void)
{
/* Enable PWR and BKP clocks */
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR | RCC_APB1Periph_BKP, ENABLE);
/* Allow access to BKP area */
PWR_BackupAccessCmd(ENABLE);
/* Reset BKP */
BKP_DeInit();
#ifdef RTCClockSource_LSI
/* Enable internal RTC clock */
RCC_LSICmd(ENABLE);
/* Wait for the RTC internal clock to be ready*/
while(RCC_GetFlagStatus(RCC_FLAG_LSIRDY) == RESET)
{
}
/* Select RTC internal clock as RTC clock */
RCC_RTCCLKConfig(RCC_RTCCLKSource_LSI);
#elif defined RTCClockSource_LSE
/* Enable RTC external clock */
RCC_LSEConfig(RCC_LSE_ON);
/* Wait for RTC external clock to be ready*/
while(RCC_GetFlagStatus(RCC_FLAG_LSERDY) == RESET)
{
}
/* Select RTC external clock as RTC clock */
RCC_RTCCLKConfig(RCC_RTCCLKSource_LSE);
#endif
/* Enable RTC clock */
RCC_RTCCLKCmd(ENABLE);
#ifdef RTCClockOutput_Enable
/* Disable the Tamper Pin */
BKP_TamperPinCmd(DISABLE); /* To output RTCCLK/64 on Tamper pin, the tamper
functionality must be disabled */
/* Enable RTC clock output on TAMPER pin */
BKP_RTCCalibrationClockOutputCmd(ENABLE);
#endif
/* Wait for RTC register synchronization */
RTC_WaitForSynchro();
/* Wait for writing RTC register to complete */
RTC_WaitForLastTask();
/* Enable RTC naozhong interrupt */
RTC_ITConfig(RTC_IT_ALR, ENABLE);
/* Wait for writing RTC register to complete */
RTC_WaitForLastTask();
/* Enable RTC seconds interrupt */
RTC_ITConfig(RTC_IT_SEC, ENABLE);
/* Wait for writing RTC register to complete */
RTC_WaitForLastTask();
/* Set RTC prescaler */
#ifdef RTCClockSource_LSI
RTC_SetPrescaler(31999); /* RTC period = RTCCLK/RTC_PR = (32.000 KHz)/(31999+1) */
#elif defined RTCClockSource_LSE
RTC_SetPrescaler(32767); /* RTC period = RTCCLK/RTC_PR = (32.768 KHz)/(32767+1) */
#endif
/* Wait for writing RTC register to complete */
RTC_WaitForLastTask();
}
void RTCAlarm_IRQHandler(void)
{
RTC_WaitForSynchro();
if(RTC_GetITStatus(RTC_IT_ALR) != RESET)
{
//printf("mmmmmm");
EXTI_ClearITPendingBit(EXTI_Line17);
RTC_WaitForLastTask();
if(PWR_GetFlagStatus(PWR_FLAG_WU) != RESET)
{
// Clear the wake-up flag
PWR_ClearFlag(PWR_FLAG_WU);
RTC_WaitForLastTask();
}
RTC_ClearITPendingBit(RTC_IT_ALR);
RTC_WaitForLastTask();
printf("\nIt will wake up after %ds\n",standbytime);
RTC_Enter_StandbyMode(standbytime); //Wake up after standbytime seconds
}
}
void RTC_Enter_StandbyMode(u32 s)
{
RTC_WaitForLastTask();
RTC_SetAlarm(RTC_GetCounter()+s);
RTC_WaitForLastTask();
// Enter standby mode, at this time all 1.8V domain clocks are turned off, HIS and HSE oscillators are turned off, and voltage regulators are turned off.
// Only WKUP pin rising edge, RTC warning event, NRST pin external reset, IWDG reset.
/* Request to enter STANDBY mode (Wake Up flag is cleared in PWR_EnterSTANDBYMode function) */
PWR_EnterSTANDBYMode();
}
5. In the next few days, I will study the shutdown mode and power consumption issues.
Previous article:STM32 sleep and wake-up
Next article:STM32 enters low power mode and wakes up (RTC+interrupt)
Recommended ReadingLatest update time:2024-11-16 15:30
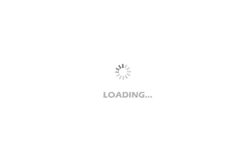
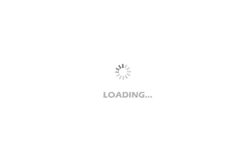
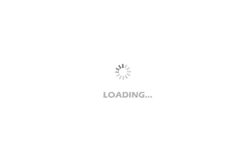
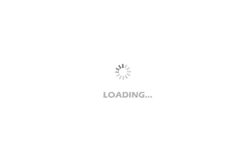
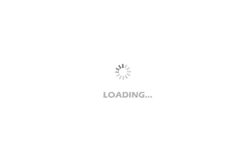
- Popular Resources
- Popular amplifiers
-
Automotive Electronics S32K Series Microcontrollers: Based on ARM Cortex-M4F Core
-
The STM32 MCU drives the BMP280 absolute pressure sensor program and has been debugged
-
DigiKey \"Smart Manufacturing, Non-stop Happiness\" Creative Competition - Small Weather Station - STM32F103 Sensor Driver Code
-
Android In-depth Exploration (Volume 1): HAL and Driver Development
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Looking for a current/voltage limiting IC
- EEWORLD University Hall----Why is the embedded microprocessor architecture design so complicated?
- MSP430F5438A
- Problems encountered during ads2020 installation
- RF power testing is that simple when explained thoroughly!
- How to import pictures in AD19 version and run script software crash
- JD642 SDRAM bus matching pre-simulation and pcb download
- [STM32WB55 Review] 6# Use of STM32WB development board UART
- [New Year's Taste Competition] + New Year's Eve dinner + New Year's 7 days of fun, I wish you all a prosperous New Year, smooth sailing and a good start.
- Sell TI POS Kit Chip