References: ARM Cortex™-M4F Technical Reference Manual - Chapter 4.5 SysTick Timer (STK)
SysTick (system tick timer) is essentially a timer embedded in NVIC. It is a peripheral in the kernel and a 24-bit down-counting counter. The counter is reduced by 1 every 1/SYSCLK. When the value of the register is reduced to 0, a (hardware) interrupt will be generated, also called a tick interrupt. The main purpose is 1. to generate accurate delays. 2. Generally used in operating systems to generate time bases, maintain the heartbeat of the operating system and provide a separate heartbeat (clock) beat for the operating system. For an introduction to SyeTick, I recommend reading this great blog https://blog.csdn.net/yx_l128125/article/details/7884423.
There are four SysTick registers in total. When using SysTick to generate timing, you only need to configure the first three registers, and the last calibration value register does not need to be used.
The following is a description of each register
The SysTick control and status register CTRL is mainly used to read the value in the current numerical register, select the clock source, enable exception requests, and enable the timer.
The SysTick reload value register LOAD is mainly used to store the count value, the maximum
SysTick current value register VAL, each counter is reduced by 1 every 1/SYSCLK. When it is reduced to 0, the count flag is set to 1, and then the value in the reload value register is written into the current value register, and the count flag is cleared.
Sys calibration value register CALIB
The above is the introduction of SysTick. Next, we need to operate it.
We need to use SysTick to time the LED light status to change once every 1S, so
1. First, you should configure the SysTick related registers to generate a 1s delay
2. Initialize LED lights
3. Write the main function
Since Systick is embedded in the kernel, only LED modules are needed in the hardware circuit. If you want to use SysTick to switch the state of the running light once every 1s, you must first configure SysTick. Here we directly use the STM32 official library function SysTick_Config (uint32_t ticks). In the kernel header file core_cm4.h, the function of this function is to configure the system timer and interrupt, and turn on SysTick, so that the counter is in free counting mode to generate a periodic interrupt. In this function, the parameter ticks is used to set the value of the reload register, which cannot exceed the value of the reload register. When the value of the reload register is reduced to 0, an interrupt will be generated, and then the value of the reload register is written into the current counter to count down again, and this cycle will continue. Next, this library function configures the interrupt priority for the interrupt (for interrupt priority, you can see the STM32 interrupt and NVIC overview), and then assigns the initial value to the current value register, then configures the clock source, enables interrupts, and turns on the timer. When it returns 0, it means that the function has been implemented, and returning 1 indicates failure. This initialization function mainly configures three registers in SysTick: LOAD, VAL, CTRL, and also calls NVIC_SetPriority() to configure the interrupt priority function.
Because SysTick is a peripheral in the core, the clock selection is HCLK180MHz clock
Since there is no official initialization function for SysTick, only the configuration function Config, we simply write a SysTick_Init() function ourselves
Because HCLK is 180MHz, when ticks=SystemFrequency/1000 (180 000 000/1000), the value of ticks decreases by 1 every time 1/SystemFrequency passes, so the counting time is ticks/(1/SystemFrequency)=1ms, so we configure SysTick to interrupt once every 10us.
After configuring SysTick, we only need to define a variable to record the number of interrupts N. N*SysTick is equal to the time we want to time.
nTime is the number of times I enter the SysTick interrupt. Every time I enter the interrupt service function, TimingDelay decreases by 1. When TimingDelay is not equal to 0, the delay ends.
The interrupt service function is as follows
Now that we have described the SysTick configuration, let's initialize the LED light.
I will not go into details about the initialization of the LED. You can refer to the STM32 firmware library function to light up the LED. The code is as follows
#include "stm32f4xx_gpio.h"
#define GPIO_R_Pin GPIO_Pin_10
#define GPIO_R_Port GPIOH
#define GPIO_G_Pin GPIO_Pin_11
#define GPIO_G_Port GPIOH
#define GPIO_B_Pin GPIO_Pin_12
#define GPIO_B_Port GPIOH
/****************************Macro to control the LED light on and off***************/
/*Directly operate registers to control IO*/
#define LED_PORT_OUT_HI(p,i) { p->BSRRL = i ;} //Output is high level
#define LED_PORT_OUT_LO(p,i) { p->BSRRH = i ;} //Output is low level
#define LED_PORT_OUT_Toggle(p,i) { p->BSRRL ^= i ;} // Output is inverted
/*Define macros to control IO*/
#define LED_R_Toggle LED_PORT_OUT_Toggle(GPIO_R_Port,GPIO_R_Pin)
#define LED_R_ON LED_PORT_OUT_LO(GPIO_R_Port,GPIO_R_Pin)
#define LED_R_OFF LED_PORT_OUT_HI(GPIO_R_Port,GPIO_R_Pin)
#define LED_G_Toggle LED_PORT_OUT_Toggle(GPIO_G_Port,GPIO_G_Pin)
#define LED_G_ON LED_PORT_OUT_LO(GPIO_G_Port,GPIO_G_Pin)
#define LED_G_OFF LED_PORT_OUT_HI(GPIO_G_Port,GPIO_G_Pin)
#define LED_B_Toggle LED_PORT_OUT_Toggle(GPIO_B_Port,GPIO_B_Pin)
#define LED_B_ON LED_PORT_OUT_LO(GPIO_B_Port,GPIO_B_Pin)
#define LED_B_OFF LED_PORT_OUT_HI(GPIO_B_Port,GPIO_B_Pin)
#define LED_RGBOFF LED_R_OFF;\
LED_G_OFF;\
LED_B_OFF
void LED_GPIO_Config(void);
#include "bsp_led.h"
/**
* @brief Initialize the IO to control the LED
* @param None
* @retval None
*/
void LED_GPIO_Config(void)
{
/*Define a GPIO_InitTypeDef type structure*/
GPIO_InitTypeDef GPIO_InitStructure;
/*Turn on LED related GPIO peripheral clock*/
RCC_AHB1PeriphClockCmd ( LED1_GPIO_CLK|
LED2_GPIO_CLK|
LED3_GPIO_CLK, ENABLE);
/*Select the GPIO pin to control*/
GPIO_InitStructure.GPIO_Pin = LED1_PIN;
/*Set the pin mode to output mode*/
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
/*Set the output type of the pin to push-pull output*/
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
/*Set the pin to pull-up mode*/
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
/*Set the pin rate to 2MHz */
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_2MHz;
/*Call library function to initialize GPIO using GPIO_InitStructure configured above*/
GPIO_Init(LED1_GPIO_PORT, &GPIO_InitStructure);
/*Select the GPIO pin to control*/
GPIO_InitStructure.GPIO_Pin = LED2_PIN;
GPIO_Init(LED2_GPIO_PORT, &GPIO_InitStructure);
/*Select the GPIO pin to control*/
GPIO_InitStructure.GPIO_Pin = LED3_PIN;
GPIO_Init(LED3_GPIO_PORT, &GPIO_InitStructure);
/*Turn off the RGB light*/
LED_RGBOFF;
}
After configuring SysTick and LED, let's start writing the main function
The main function is as follows
int main(void)
{
/* LED port initialization */
LED_GPIO_Config();
/* Configure SysTick to interrupt once every 10us, and trigger a timer interrupt after the time is up.
* Enter the SysTick_Handler process of the stm32fxx_it.c file and count the number of interrupts
*/
SysTick_Init();
while(1)
{
LED_RED;
Delay_us(100000); // 10000 * 10us = 1000ms
//Delay 1s
LED_GREEN;
Delay_us(100000);
//Delay 1s
LED_BLUE;
Delay_us(100000);
//Delay 1s
}
}
Previous article:STM32_USART serial communication detailed explanation
Next article:STM32_EXTI external interrupt study notes
Recommended ReadingLatest update time:2024-11-16 14:42
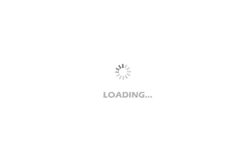
- Popular Resources
- Popular amplifiers
-
Automotive Electronics S32K Series Microcontrollers: Based on ARM Cortex-M4F Core
-
uCOS-III Kernel Implementation and Application Development Practical Guide - Based on STM32 (Wildfire)
-
RT-Thread Kernel Implementation and Application Development Practical Guide: Based on STM32
-
FreeRTOS kernel implementation and application development practical guide: based on STM32
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Limitations of Flash_API for C2000 Series (28335) DSP
- EEWORLD University - Power stage protection, current sensing, efficiency analysis and related TI designs
- Amplifier
- Multi-cell 36-48V Battery Management System Reference Design
- EBAZ4205 mining board transformation based on Z7010
- Samsung closes another factory in China! N+5 compensation higher than national standards
- IMX6-A9 series: display function test
- 5G in 60: An introduction to 5G
- Battery system state of charge calculation algorithm problem
- I'm a newbie in your place, please take care of me, thank you!