Purpose:
1. Understand the role of interrupts;
2. Master the interrupt processing flow of embedded systems;
3. Master ARM external interrupt programming.
Experimental content (including steps):
1. Overall steps: compile first, start the target machine, wait for the display to show 2440, then create a link, download, run, and observe whether HELLOO is displayed. Press the EXINT2 button to see whether the LED light changes the display effect.
2. Experimental process: During the experiment, the hardware connection failed. The team members thought it was a problem with the connection between the target machine and the computer. They unplugged and plugged it in, but it still didn't work. Later, they changed the computer connection many times and spent a lot of time to finally solve the problem. Then they modified the code, compiled, linked, downloaded, and ran it within a tight time, and finally successfully displayed the effect. After understanding the principle, by running the digital tube display code in the main program, the host machine can correctly display the word HELLOO, and then press the EXINT2 button to request an interrupt and execute the interrupt service program, so that the LED light can change the display
3. The code is as follows:
/****************************************************** ***************************/
/*Experimental phenomenon: The common anode digital tube displays HELLOO. After the external interrupt is triggered, the LED light changes from odd-numbered tubes on (off) to even-numbered tubes on (off)*/
/****************************************************** ***************************/
#define U8 unsigned char
/*HELLOO character encoding*/
unsigned char hello[6] = {
0x89, 0x86, 0xc7, 0xc7, 0xc0, 0xc0,
};
/*Status code of only one of the six digital tubes is on*/
unsigned char con[6] = {
0xdf, 0xef, 0xf7, 0xfb, 0xfd, 0xfe
};
void myDelay(int time);
Void Delay(int time);
/****************************************************** *********************
// Function name: Isr_Init
// Description : Interrupt initialization function
// Return type: void
// Argument : void
*************************************************** *******************/
void Isr_Init(void)
{
pISR_UNDEF = (unsigned)HaltUndef;
pISR_SWI = (unsigned)HaltSwi;
pISR_PABORT = (unsigned)HaltPabort;
pISR_DABORT = (unsigned)HaltDabort;
rINTMOD = 0x0; //Interrupt mode is set to IRQ
rINTMSK = BIT_ALLMSK; //All interrupt is masked.
rINTSUBMSK = BIT_SUB_ALLMSK; //All sub-interrupt is masked.
}
/****************************************************** *********************
// Function name: Isr_Request
// Description : Register interrupt function (interrupt request)
// Return type: void
// Argument : int irq_no
// #define IRQ_EINT0 1
// #define IRQ_EINT1 2
// #define IRQ_EINT2 3
// #define IRQ_EINT3 4 //**
// #define IRQ_EINT4_7 5
// #define IRQ_EINT8_23 6
// #define IRQ_NOTUSED6 7
// #define IRQ_BAT_FLT 8
// #define IRQ_TICK 9
// #define IRQ_WDT 10
// #define IRQ_TIMER0 11
// #define IRQ_TIMER1 12
// #define IRQ_TIMER2 13
// #define IRQ_TIMER3 14
// #define IRQ_TIMER4 15
// #define IRQ_UART2 16
// #define IRQ_LCD 17
// #define IRQ_DMA0 18
// #define IRQ_DMA1 19
// #define IRQ_DMA2 20
// #define IRQ_DMA3 21
// #define IRQ_SDI 22
// #define IRQ_SPI0 23
// #define IRQ_UART1 24
// #define IRQ_NOTUSED24 25
// #define IRQ_USBD 26
// #define IRQ_USBH 27
// #define IRQ_IIC 28
// #define IRQ_UART0 29
// #define IRQ_SPI1 30
// #define IRQ_RTC 31
// #define IRQ_ADC 32
// Argument: void* irq_routine
*************************************************** *******************/
//The first parameter indicates the interrupt request source, and the second parameter is the interrupt service function defined by the interrupt request source
void Irq_Request(int irq_no, void* irq_routine)
{
if(irq_no >= IRQ_MIN && irq_no <= IRQ_MAX)
*(unsigned int*)((irq_no - 1) * sizeof(unsigned int) + (unsigned int)(_ISR_STARTADDRESS+0x20)) = (unsigned int)irq_routine;
// _ISR_STARTADDRESS+0x20 is to skip the previous exception vector and enter the IRQ interrupt vector
// Three-level jump to the specified address, that is, jump from the flash to the interrupt entry of the RAM, and then jump from the interrupt entry to the interrupt // distribution routine entry
}
/****************************************************** *********************
// Function name: Irq_Enable
// Description : Enable interrupt
// Return type: void
// Argument : int irq_no
*************************************************** *******************/
//The parameter indicates the interrupt request source. This function enables an interrupt.
void Irq_Enable(int irq_no)
{
if(irq_no >= IRQ_MIN && irq_no <= IRQ_MAX)
rINTMSK &= ~(1 << (irq_no - 1)); //Set all to zero, enable interrupt
}
/****************************************************** *********************
// Function name:Irq_Disable
// Description : Disable interrupt
// Return type: void
// Argument : int irq_no
*************************************************** *******************/
//The parameter indicates the interrupt request source. This function disables a certain interrupt
void Irq_Disable(int irq_no)
{
if(irq_no >= IRQ_MIN && irq_no <= IRQ_MAX)
rINTMSK |= (1 << (irq_no - 1)); //Set to 1, disable interrupt
}
/****************************************************** *********************
// Function name: Irq_Clear
// Description : Clear interrupt
// Return type: void
// Argument : int irq_no
*************************************************** *******************/
//The parameter indicates the interrupt request source. This function clears an interrupt request.
void Irq_Clear(int irq_no)
{
rSRCPND = (1 << (irq_no - 1)); //Set to 1 and clear the corresponding bit of the register
rINTPND = (1 << (irq_no - 1)); //Set to 1 and clear the corresponding bit of the register
rINTPND;
}
/****************************************************** ******************************
// Function name: eint2_isr
// Description : Main function
// Return type: void
// Argument : void
*************************************************** ***************************/
void Main(void)
{
/* Configure system clock */
ChangeClockDivider(2,1);
U32 mpll_val = 0 ;
mpll_val = (92<<12)|(1<<4)|(1);
ChangeMPllValue((mpll_val>>12)&0xff, (mpll_val>>4)&0x3f, mpll_val&3);
/* Interrupt initialization */
Isr_Init();
/* Initialize port */
Port_Init();
/* Initialize the serial port */
Uart_Init(0,115200);
Uart_Select(0);
/* Print prompt information */
PRINTF("\n---External interrupt test program---\n");
PRINTF("\nPlease connect UART0 to the PC serial port, and then start the HyperTerminal program (115200, 8, N, 1)\n");
PRINTF("\nExternal interrupt test starts\n");
/* Request interrupt */
Irq_Request(IRQ_EINT3, eint3_isr);
/* Enable interrupt */
Irq_Enable(IRQ_EINT3);
dither_count2 = 0;
dither_count3 = 0;
while(1){
int i, j;
int flag = 1;
for(j=0; ; j++){
*((U8*) 0x20007000) = 0x80;
/* The digital tube displays characters from 0 to F in sequence*/
for(i=0;i<6;i++){
/* Look up the table and output data*/
*((U8*) 0x20007000) = con[i];
*((U8*) 0x20006000) = hello[i];
myDelay(1);
}
dither_count3++;
}
}
}
/****************************************************** ******************************
// Function name: eint3_isr
// Description : EINT3 interrupt handler
// Return type: int
// Argument : void
*************************************************** ***************************/
void eint3_isr(void)
{
Irq_Clear(IRQ_EINT3); // Clear interrupt
if(dither_count3 > 10) //If count is greater than 10, change the LED display
{
dither_count3 = 0; // clear
Led_Display(nLed); //led display
nLed = ~nLed;
}
}
Experimental summary:
lg: I have come across interrupts in computer composition and operating systems, and I have a general understanding of them, but they are all theoretical. Through this external interrupt experiment, I feel that theory is connected with practice, and I remember the MASK mentioned in the composition principle. In the experiment, I learned how to register interrupts, enable interrupts, clear interrupts, write to interrupt registers INTMSK, SRCPND, and INTPND, and the three-level jump of interrupt execution, which gave me a deeper understanding of interrupts.
zhy: In this experiment, our group spent a lot of time on connecting the devices. There were few actual code modifications, so we had more time to read the code. The entire interrupt process was implemented in the code, using two important registers: the interrupt pending register (INTPND) and the interrupt mask register (INTMSK). There are reasons for setting it to 0 or 1. This time, the external interrupt was interrupted by pressing a button, and the LED light was turned on at odd and even intervals, which gave a sense of interruption.
wq: The interrupts we learned before were superficial and our understanding was shallow. Through this interrupt experiment, we entered the hardware bottom layer and gained a deeper understanding of the interrupt process in the machine. We also learned the specific functions of registering interrupts, opening interrupts, and clearing interrupts. This external interrupt interrupts the current program by pressing a button, so that the LED light can change the display. Unfortunately, because our group spent too much time connecting to the machine, the experiment time was very short and we did not learn enough.
yy: The subject of this experiment is external interrupts of ARM basic interface. The typical steps of interrupts are: save the scene, switch mode, get the interrupt source, handle the interrupt, and return from the interrupt. I have to say that this experiment is more difficult than before, the code is more obscure to read, and it also involves more knowledge about the underlying layer, but after completing the experiment, I do feel that I have a better understanding of interrupts.
cxy: The content of this experiment is interrupts. I have often heard about interrupts in operating systems and computer composition principles, but I have never really understood the specific implementation of interrupts. This experiment allowed me to understand the details of interrupts.
Previous article:ARM interrupt processing
Next article:About s3c2410 interrupt exception handling
Recommended ReadingLatest update time:2024-11-16 13:45
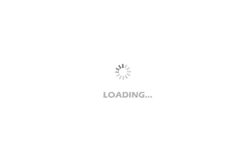
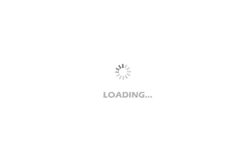
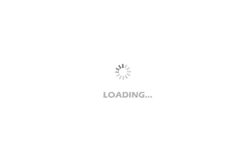
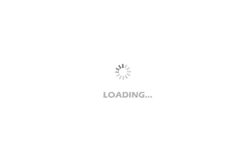
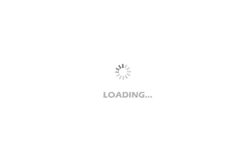
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Software - Can I use the Breaking Master on my computer to install Allegro 16.6 and 17.4 at the same time?
- CC3120 Wireless Network Processor BoosterPack Plug-in Module
- Simulink and ModelSim co-simulation to realize BLDC six-step square wave closed-loop control system
- GD32103E-EVAL Full-function evaluation board with screen
- Free application: Infineon's revolutionary photoacoustic spectroscopy (PAS) CO2 sensor, the legend of excessive CO2 leads to lack of attention
- Working principle of two matched transistor pairs
- I can't figure out the op amp formula, can someone help me look at it?
- GD32E231C-START unboxing first time
- [Repost] Popular Science of Components: Semiconductor Lasers
- How to enable UART flow control?