The purpose of the experiment:
copy the program from Steppingstone to SDRAM, and then jump to SDRAM to execute
the source program of the experiment:
@****************************************************************************
@ File: head.S
@ Function: set up SDRAM, copy the program to SDRAM, and then jump to SDRAM to continue execution
@************************************************************************
.equ MEM_CTL_BASE, 0x48000000
.equ SDRAM_BASE, 0x30000000
.text
.global _start
_start:
bl disable_watch_dog @ Turn off WATCHDOG, otherwise the CPU will restart continuously
bl memsetup @ Set up the storage controller
bl copy_steppingstone_to_sdram @ Copy the code to SDRAM
ldr pc, =on_sdram @ Jump to SDRAM to continue execution
on_sdram:
ldr sp, =0x34000000 @ Set up the stack
bl main
halt_loop:
b halt_loop
disable_watch_dog:
@ Write 0 to the WATCHDOG register
mov r1, #0x53000000
mov r2, #0x0
str r2, [r1]
mov pc, lr @ Return
copy_steppingstone_to_sdram:
@ Copy all 4K data of Steppingstone to SDRAM
@ The starting address of Steppingstone is 0x00000000, and the starting address of SDRAM is 0x30000000
mov r1, #0
ldr r2, =SDRAM_BASE
mov r3, #4*1024
1:
ldr r4, [r1],#4 @ Read 4 bytes of data from Steppingstone and add 4 to the source address
str r4, [r2],#4 @ Copy this 4 bytes of data to SDRAM and add 4 to the destination address
cmp r1, r3 @ Check whether the source address is equal to the unsigned address of Steppingstone.
bne 1b @ If the copying is not completed, continue
mov pc, lr @ Return to
memsetup:
@ Set up the memory controller to use peripherals such as SDRAM
mov r1, #MEM_CTL_BASE @ The starting address of the 13 registers of the memory controller
adrl r2, mem_cfg_val @ The starting storage address of these 13 values
add r3, r1, #52 @ 13*4 = 54
1: @ This is the value 1, which is called a local label in professional terminology and is mainly used for the statement bne 1b
ldr r4, [r2], #4 @ Read the set value and add 4 to r2
str r4, [r1], #4 @ Write this value to the register and add 4 to r1
cmp r1, r3 @ Determine whether all 13 registers have been set
bne 1b @ If it is not written, continue
mov pc, lr @ Return.align
4
mem_cfg_val:
@ The set value of the 13 registers of the memory
controller.long 0x22011110 @ BWSCON @In fact, the setting of the BWSCON register mainly integrates the connection between the remaining BANKs of the storage controller and peripheral devices.long
0x00000700 @ BANKCON0 @For the settings of these registers, you can refer to the books of Mr. Wei Dongshan.long
0x00000700 @ BANKCON1
.long 0x00000700 @ BANKCON2
.long 0x00000700 @ BANKCON3
.long 0x00000700 @ BANKCON4
.long 0x00000700 @ BANKCON5
.long 0x00018005 @ BANKCON6
.long 0x00018005 @ BANKCON7
.long 0x008C07A3 @ REFRESH
.long 0x000000B1 @ BANKSIZE
.long 0x00000030 @ MRSRB6
.long 0x00000030 @ MRSRB7
/****************************************************** *************************
led.c
********************** *************************************************** **/
#define GPFCON (*(volatile unsigned long *)0x56000050)
#define GPFDAT (*(volatile unsigned long *)0x56000054)
#define GPF4_out (1<<(4*2))
#define GPF5_out (1<<( 5*2))
#define GPF6_out (1<<(6*2))
void wait(volatile unsigned long dly)
{
for(; dly > 0; dly--);
}
int main(void)
{
unsigned long i = 0;
GPFCON = GPF4_out|GPF5_out|GPF6_out; // Set the three pins of GPF4/5/6 corresponding to LED1, 2, and 4 as output
while(1)
{
wait(30000);
GPFDAT = (~(i<<4)); // Turn on LED1,2,4 according to the value of i
if(++i == 8)
i = 0;
}
return 0;
}
/****************************************************** *************************
Makefile
************************ *************************************************** /
sdram.bin : head.S leds.c
arm-linux-gcc -c -o head.o head.S
arm-linux-gcc -c -o leds.o leds.c
arm-linux-ld -Ttext 0x30000000 head. o leds.o -o sdram_elf
arm-linux-objcopy -O binary -S sdram_elf sdram.bin
arm-linux-objdump -D -m arm sdram_elf > sdram.dis
clean:
rm -f sdram.dis sdram.bin sdram_elf *. o
Summary of experimental questions:
I. What role does the memory controller play during this period?
In S3C2440/S3C2410, the access range of the 27 address lines ADDR0-ADDR26 is only 128M.
The CPU also leads out 8 chip select signals nGCS0-nGCS7, corresponding to BANK0-BANK7. When a chip select signal is pulled low, the
corresponding memory controller's BANK is selected, and each BANK is connected to an external device. When the CPU sends a certain address signal,
it will read the configuration information of the register in the memory controller, so as to know how to access the external device. I think this
is probably the role of the memory controller.
II. What is the difference between the memory controller's BANK and the SDRAM's Bank? In TQ2440, the memory
controller
has 8 BANKs, which can be connected to 8 different devices respectively. Through the chip select signal, each device can be accessed independently;
SDRAM Bank
The inside of SDRAM is a storage array. The array is like a table, "fill" the data into it, you can think of it as a table.
Just like the retrieval principle of the table, first specify a row (Row), then specify a column (Column),
we can accurately find the required cell, this is the basic principle of memory chip addressing.
For memory, this cell can be called a storage unit, then what is this table (storage array) called?
It is the logical bank (Logical Bank, hereinafter referred to as L-Bank), so the prepared SDRAM bank is called L-Bank
III. How to determine which bank of the storage controller the SDRAM is connected to? And how to determine the corresponding address of each bank? As for which
bank the SDRAM is connected to of the storage controller, we can know it through the chip select signal of the schematic diagram of the development board, because the chip select signal and the bank are one-to-one corresponding;
when we know which bank the SDRAM is connected to, then, how do we know the address of this SDRAM? This is mainly introduced in the user manual of S3C2440
. For each bank of the storage controller, its address has been specified.
IV. Since the link address specified in the Makefile is: 0x3000 0000, it stands to reason that these code segments are all executed in SDRAM at the beginning, and SDRAM
is not initialized at the beginning, isn't this contradictory?
The main reason is that in the code segment, bl disable_watch_dog, bl memsetup, bl copy_steppingstone_to_sdram, because they are all relative jumps, so these are position-independent instructions, that is, these codes can be executed correctly even if they are not in the runtime address space specified at link time. It is a special code that can be loaded into any address space and can be executed normally. When the program runs to the ldr pc, =on_sdram instruction, because it takes the absolute address of on_sdram, and its address label is in SDRAM, so after executing this statement, the program runs in SDRAM. For this point, you can Baidu the knowledge of position-independent code.
Note: The reference book is "Complete Manual for Embedded Linux Application Development"
Previous article:ARM9 interrupt architecture
Next article:LED Bare Program
Recommended ReadingLatest update time:2024-11-16 17:39
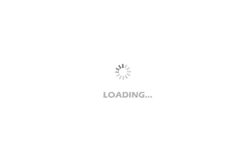
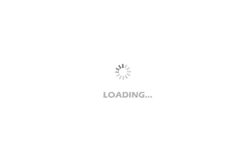
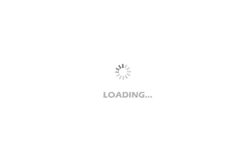
- Popular Resources
- Popular amplifiers
-
Practical Deep Neural Networks on Mobile Platforms: Principles, Architecture, and Optimization
-
ARM Embedded System Principles and Applications (Wang Xiaofeng)
-
ARM Cortex-M4+Wi-Fi MCU Application Guide (Embedded Technology and Application Series) (Guo Shujun)
-
osk5912 evaluation board example source code
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Review summary: Anxinke NB-IoT development board EC-01F-Kit
- Please tell me what type of inductor this is
- Feedback coefficient expression calculation
- I am a newcomer to the switching power supply industry and need to learn how to calculate the counter-transformer. Do you have any good experience or t...
- Elimination of low frequency signals
- Where can I find the German patent number?
- EK140 Burning Guide
- Two small questions about the lithium battery charging chip bq2407x
- Operating System Timeline and Family Tree
- Report Summary: Silicon Labs Development Kit Review