The experimental platform list is as follows:
Development board: Battleship STM32ZET6 V3.4
Core chip: STM32F103RCT6
Development environment: MDK-ARM Version:5.10
PC operating system: Windows 7 Ultimate
Emulator: ST-Link
Open the MDK development platform and click "Project - New μVision Project" in the menu bar to create a new project. Then select the appropriate chip in the pop-up "Select Device for Target 1" dialog box. Since I use STM32F103ZET6, I choose ST - STM32F103ZE.
After selecting the chip, a message box will pop up, "Copy STM32 Startup Code to Project Folder and Add File to Project?" asking if you need to load the startup code. Select "Yes" and enter the project.
The so-called startup code is a piece of code executed by the processor when it starts up. Its main tasks are to initialize the processor mode, set the stack, initialize variables, etc. Since the above operations are closely related to the processor architecture and system configuration, they are generally written in assembly. For beginners, it is difficult to design startup code by themselves. The MDK development platform has built-in startup codes for some commonly used chips. Therefore, when creating a new project, it is best to use the default startup code. Of course, chip manufacturers will also write some startup codes themselves and put them on the official website for developers to download.
After entering the project, we can start writing code. First, we need to create a new file and save it in *.c format, so that the development environment can recognize some common keywords and other information in the written code. I will save it directly as main.c. Then right-click Source Group 1 in the Project Workspace on the left side of the screen, select Add Files to Group "Source Group 1", and add the main.c we just saved to Source Group 1, or you can directly double-click Source Group 1 to add files.
Next, you can start writing code. For beginners, the most basic operation should be the operation of the chip IO port. Therefore, when I was learning ARM, the first project I chose to light up the three LED lights on the development board in sequence. There are a total of 7 groups of general-purpose input and output interfaces (General-Purpose Inputs/Outputs) in STM32F103VET6. Each GPIO pin can be configured by software as an output (push-pull or open-drain), input (with or without pull-up or pull-down) or multiplexed peripheral function port. Most GPIO pins are shared with digital or analog multiplexed peripherals. Please refer to the Datasheet for specific details.
Back to the MDK development platform, now we need to add relevant code to main.c. The code list is as follows:
#include "stm32f10x_lib.h"
int main()
{
int i;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOD|RCC_APB2Periph_GPIOB, ENABLE); //Enable the peripheral clock
GPIOD->CRL = 0x33333333; //Set port configuration register
GPIOB->CRL = 0x33333333;
while(1)
{
GPIOD->ODR = 0xffffffbf; //Set port output register
for(i=0;i<1000000;i++); //delay
GPIOD->ODR = 0xffffffff7;
for(i=0;i<1000000;i++);
GPIOD->ODR = 0x00000000;
GPIOB->ODR = 0xffffffff;
for(i=0;i<1000000;i++);
GPIOB->ODR = 0x00000000;
}
}
In the above code, #include "stm32f10x_lib.h" contains the basic header files required to develop the stm32f10x series chips. Be sure to include this header file when writing the program.
The RCC_APB2PeriphClockCmd() function is used to set the peripheral clock. The difference between ARM and C51 microcontrollers is that when peripherals are not in use, such as IO ports, ADC, timers, etc., the clocks are disabled to achieve energy saving. Only the peripherals that are needed are turned on. Therefore, when GPIOB and GPIOD are needed, we need to turn on their clocks first. The specific functions used are those in the function library:
void RCC_APB2PeriphClockCmd(uint32_t RCC_APB2Periph, FunctionalState NewState)
Among them, the first parameter needs to indicate which port's clock to enable, RCC_APB2Periph_GPIOx is to enable the GPIOx clock, and the second parameter needs to indicate whether to enable or disable, ENABLE/DISABLE.
After turning on the peripheral clock, the GPIO configuration registers are set. For specific settings, refer to "7.1 General IO Port" in the book "STM32 Processor Development and Application Based on MDK". The while loop assigns values to the GPIO port output registers. Since the three LED lights of this struggle development board are connected to D3, D6 and B5 respectively, it is enough to set the corresponding bits of the D port and the B port to 1.
After compiling, we found that the compiler reported an error, Undefined symbol RCC_APB2PeriphClockCmd, because the RCC_APB2PeriphClockCmd() function we used was declared in the header file but not defined in the C file. This function is in .. KeilARMRV31LIBSTSTM32F10xstm32f10x_rcc.c. Copy this file to the root directory of the project, and then add it to the Workspace on the left side of the screen.
In fact, in the MDK library, many macros are defined to avoid us looking up relevant information to set each bit of the register. For example, in this experiment, the on and off of the LED can also be achieved through the following code.
#include "stm32f10x_lib.h"
int main()
{
int i;
GPIO_InitTypeDef GPIO_InitStructure; //Define GPIO macro operation structure
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOD | RCC_APB2Periph_GPIOB,ENABLE); //Peripheral clock configuration, turn on the clocks of GPIOB and GPIOD
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; //Configure port B5 as general push-pull output
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; //Port line flip speed is 50MHz
GPIO_Init(GPIOB, &GPIO_InitStructure); //Configure the GPIOB port
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6|GPIO_Pin_3; //Configure D3 and D6 ports as push-pull output
GPIO_Init(GPIOD, &GPIO_InitStructure); //Configure the GPIOD port
while(1)
{
GPIO_SetBits(GPIOB, GPIO_Pin_5); //Port B5 outputs high level
GPIO_ResetBits(GPIOD, GPIO_Pin_6); //Port D6 outputs low level
GPIO_ResetBits(GPIOD, GPIO_Pin_3); //Port D3 outputs low level
for(i=0;i<1000000;i++);
GPIO_ResetBits(GPIOB, GPIO_Pin_5);
GPIO_ResetBits(GPIOD, GPIO_Pin_6);
GPIO_SetBits(GPIOD, GPIO_Pin_3);
for(i=0;i<1000000;i++);
GPIO_ResetBits(GPIOB, GPIO_Pin_5);
GPIO_ResetBits(GPIOD, GPIO_Pin_3);
GPIO_SetBits(GPIOD, GPIO_Pin_6);
for(i=0;i<1000000;i++);
}
}
Since we used the GPIO_InitTypeDef type, we need to find its definition, which is contained in "…KeilARMRV31LIBSTSTM32F10xstm32f10x_gpio.c". Copy the file to the project root directory, and then add it to the project so that the compilation will not report an error.
In most C compilers, all variable declarations are required before executing the statement block. That is to say, if the variables need to be defined, they must be defined at the beginning of entering the main function. If the variables are defined after a certain statement is executed, an error will be reported.
Previous article:STM32-Interrupt Application
Next article:STM32 timer basics
Recommended ReadingLatest update time:2024-11-16 16:39
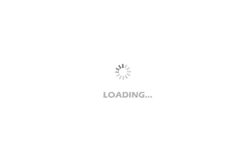
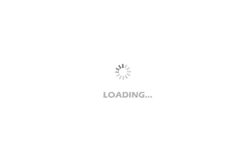
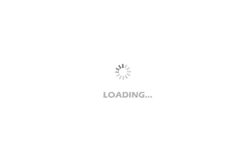
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications