I won't go into details about the serial port. Although the serial port is an old interface with a slow speed and has long been eliminated in computers, it is still widely used in embedded systems due to its simple structure and ease of use, and perhaps also because it has a basic support for use in computers (the microcontroller is following the path that computers once took).
In the STM32F429, there are a total of 8 serial ports, 4 USARTs and 4 UARTs.
The goal to be achieved today is to let STM32 send data to the computer through the serial port, so that it can really output "Hello World!"! We
still use the previous project, but the project structure is slightly changed, as shown below:
Unlike before, I now choose to copy the entire firmware library to the STM32F4xx_StdPeriph folder, and add the C file corresponding to a peripheral when using it.
This communication is implemented using the query method. When the baud rate is 115200bps. Although for the 168M CPU main frequency, using the query method to send data is too wasteful, but because the CPU has no other tasks, and I am eager to see the results, compared to configuring interrupts and writing a FIFO, I think using the query method is easier for me to accept:)
Initialization of USART1.
Like GPIO, USART also provides an initialization structure. Fill in the corresponding data and pass it as a parameter to USART_Init().
In addition, there are two points to note. One is to enable the USART clock (this is the same for all peripherals), and the other is to do relevant configuration because of pin multiplexing.
The STM32F4 series serial port pin multiplexing is different from the STM32F1 series:
the STM32F1 series only needs to set the pin mode to GPIO AF mode, while the STM32F4 series needs to call the GPIO_PinAFConfig() function to configure the corresponding multiplexed pins.
/**
* @brief UART1 initialization, 115200, 8, N, 1
*/
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// Enable the corresponding clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
// Pin multiplexing function
GPIO_PinAFConfig(GPIOA, GPIO_PinSource9, GPIO_AF_USART1);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource10, GPIO_AF_USART1);
// Initialize the pins used by the serial port
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9 | GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_100MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART1 initialization
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
return;
}
/**
* @brief Send a string from the serial port, using the query method
*
* @param str The string to be sent
*/
void USART1_Puts(uint8_t *str)
{
while (*str != '\0')
{
USART_SendData(USART1, *str);
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) != SET);
str++;
}
return;
}
main function:
int main(void)
{
RCC_Config();
SysTick_Init();
LED_GPIO_Config();
USART1_Init();
Delay_ms(1);
while (1)
{
USART1_Puts("Hello World!");
GPIO_ResetBits(GPIOG, GPIO_Pin_13);
GPIO_SetBits(GPIOG, GPIO_Pin_14);
Delay_ms(500);
USART1_Puts("http://www.DevLabs.cn\n");
GPIO_ResetBits(GPIOG, GPIO_Pin_14);
GPIO_SetBits(GPIOG, GPIO_Pin_13);
Delay_ms(500);
}
}
Another point is that the STM32F429 Discovery cannot communicate directly with the computer. I use a USB-to-serial port CP2102 module. Connect the RX of the serial port module to PA9 of the STM32F429 and the TX to PA10. Then the computer can receive data correctly, as shown in the figure.
There is a small problem. I found that the first character sent after reset will be swallowed, but it is normal when debugging. The reason is unknown and will be solved later.
Previous article:STM32 serial communication – using interrupt mode
Next article:Make LCD flash: Setting and using STM32F4SysTick
Recommended ReadingLatest update time:2024-11-16 16:48
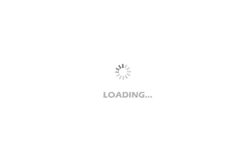
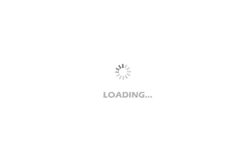
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- "Show goods" to come to a wave of commonly used development boards
- Measurement of the phase difference between a sine wave and a square wave
- [Sipeed LicheeRV 86 Panel Review] - 6 waft-ui component tests (3)
- Code size after keil compilation
- Tips for removing chip components on PCB
- Hardware System Engineer's Handbook
- Integrated operational amplifier practical circuit diagram
- For electronic hardware, there are many circuit structures, which can be said to be the units that make up a component. All circuits...
- 【Ended】Microchip Live|Wireless Power Consortium (WPC) compliant wireless charging authentication
- my country's first geostationary orbit Q/V band satellite-to-ground communication test system is successfully put into operation