I only bought a development board but it didn't have an LCD. I was so poor that I had to use a VGA monitor instead. The documentation didn't tell me how to support VGA, so I had to look it up online. Fortunately, I found a solution. Although I don't know the principle of Linux driver, I can still do it by changing the registers. Let me tell you the whole process.
The VGA interface definition is shown in the figure:
The AD chip on the board is TI's TL5632. The three output pins of this chip are connected to the RED GREEN BLUE of VGA. Its 24 input pins are connected to vd[23:0] of 2410. The VSYNC and HSYNC of 2410 are directly connected to the corresponding pins of VGA, and the others are grounded. The three encoding pins of MONITOR ID are not used.
The above is a VGA interface timing diagram with a resolution of 640×480, a refresh rate of 60 Hz, and a 16-bit color display mode. Some values in the LCD registers are determined by them:
* LCDCON1 Register
LINECNT: Status bit of line counter. Read only, no need to set.
CLKVAL: Parameter that determines the VCLK frequency. The formula is VCLK=HCLK/[(CLKVAL+1)×2], in Hz. The hardware system I use has HCLK=100 MHz, and the 640×480 display requires VCLK=25 MHz, so CLKVAL=1 needs to be set.
MMODE: Determines the speed at which VM changes. Select MMODE=O here, which is the change mode every frame.
PNRMODE: Determine the scanning mode. Select PNRMODE=0x3 for TFT LCD panel scanning mode.
BPPMODE: Determines the BPP (bits per pixel) mode. Here, select BPPMODE=0xC, which is TFT 16-bit mode.
ENVID: Data output and logic signal enable control bit. Select ENVID=1 to enable data output and logic control.
* LCDCON2 Register
VBPD: Determines the delay time before the frame synchronization signal and frame data transmission. It is the ratio of the delay time before frame data transmission and the width of the line synchronization clock interval. As shown in the figure, VBPD=t3/t6=1.02mS/31.77μs=32.
LINEVAL: Determines the vertical size of the display. Formula: LINEVAL=YSIZE-1=479.
VFPD: Determines the delay time from the completion of frame data transmission to the arrival of the next frame synchronization signal. It is the ratio of the delay time after frame data transmission and the width of the line synchronization clock interval. As shown in the figure, VFPD=t5/t6=0.35 ms/31.77μs=11.
VSPW: Determines the frame synchronization clock pulse width, which is the ratio of the frame synchronization signal clock width to the line synchronization clock interval width. As shown in the figure, VSPW = t2/t6 = 0.06 ms/31.77μs = 2.
* LCDCON3 Register
HBPD: Determines the delay time between the horizontal synchronization signal and the horizontal data transmission, and describes the number of VCLK pulses during the delay time before the horizontal data transmission. As shown in the figure, VBPD=t7×VCLK=1.89 μs×25MHz=47.
HOZAL: Determines the horizontal size of the display. The formula is HOZAL=XSIZE-1=639.
HFPD: Determines the delay time from the completion of row data transmission to the arrival of the next row synchronization signal, and describes the number of VCLK pulses within the delay time after row data transmission. As shown in the figure, HFPD=t9×VCLK=0.94 μs×25 MHz=24.
* LCDCON4 register
HSPW: determines the line synchronization clock pulse width. Describes the number of VCLK pulses within the line synchronization pulse width time, as shown in the figure, HSPW = 3.77μs × 25 MHz = 94.
* LCDCON5 Register
VSTATUS: vertical status. Read only, no need to set.
HSTATUS: Horizontal status. Read only, no need to set.
BPP24BL: Determines the display data storage format. Here, BPP24BL=0x0 is set to store in little-endian mode.
FRM565: Determine the 16-bit data output format. Here, set FRM565=0x1 for 5:6:5 format output.
INVVCLK: Determine the VCLK pulse valid edge polarity. Determine according to the screen information, here select INVVCLK=0xl, and data transmission starts when the VCLK rising edge arrives.
INVVLINE: Determines the polarity of the HSYNC pulse. As shown in the figure, it is negative polarity, so set INVVLINE = 0x1 to select a negative polarity pulse.
INVVFRAME: Determines the polarity of the VSYNC pulse. As can be seen from the figure, it is negative polarity, so set INVVFRAME = 0x1 to select a negative polarity pulse.
INVVD: Determine the pulse polarity of data output. Determine according to the screen information, here set INVVD = 0x0 to select positive polarity pulse.
INVVDEN: Determine the polarity of the VDEN signal. According to the screen information, set INVVDEN=0x0 here for a positive pulse.
INVPWREN: Determine the polarity of the PWREN signal. According to the screen information, set NVPWREN=0x0 here for a positive pulse.
INVLEND: Determine the polarity of the LEND signal. According to the screen information, set INVLEND=0x0 here for a positive pulse.
PWREN: PWREN signal output is enabled. Set PWREN = 0x1 to enable PWREN output.
ENLEND: LEND output signal is enabled. Set ENLEND = 0x1 to enable LEND output.
BSWP: Byte swap control bit. Set according to your needs. Here, set BSWP=0x0 to disable byte swap.
HWSWP: Half-word swap control bit. Set according to individual needs. Here, set HWSWP=0x1 to enable half-byte swap.
When you modify the corresponding registers in /linux-2.6.14/arch/arm/mach-s3c2410/mach-smdk2410.c as above, you can do it. Finally, in make menuconfig, select LCD support and pass the kernel startup parameter console=tty0. After power-on initialization, you will see the startup screen on the VGA monitor, saving you the trouble of buying an LCD.
-------------------------------------------------------------------------------------------
static struct s3c2410fb_mach_info hfrk_lcdcfg __initdata={
.fixed_syncs = 0,
.regs = {
.lcdcon1=S3C2410_LCDCON1_TFT16BPP | \
S3C2410_LCDCON1_TFT | \
S3C2410_LCDCON1_CLKVAL(1),
.lcdcon2=S3C2410_LCDCON2_VBPD(32) | \
S3C2410_LCDCON2_LINEVAL(479) | \
S3C2410_LCDCON2_VFPD(11) | \
S3C2410_LCDCON2_VSPW(2),
.lcdcon3=S3C2410_LCDCON3_HBPD(47) | \
S3C2410_LCDCON3_HOZVAL(639) | \
S3C2410_LCDCON3_HFPD(24),
.lcdcon4=S3C2410_LCDCON4_MVAL(1) | \
S3C2410_LCDCON4_HSPW(94),
.lcdcon5=S3C2410_LCDCON5_FRM565 | \
S3C2410_LCDCON5_INVVLINE | \
S3C2410_LCDCON5_HWSWP,
},
.lpcsel=0x0,
.gpccon=0xaaaaaaaa,
.gpccon_mask=0xffffffff,
.gpcup=0xffffffff,
.gpcup_mask=0xffffffff,
.gpdcon=0xaaaaaaaa,
.gpdcon_mask=0x0,
.gpdup=0xffffffff,
.gpdup_mask=0xffffffff,
.width=640,
.height=480,
.xres={640,640,640},
.yres={480,480,480},
.bpp={16,16,24},
};
Previous article:STM32 Keil-MDK Project Template V3.5 Firmware Library
Next article:ARM Assembly and C Mixed Programming
Recommended ReadingLatest update time:2024-11-17 00:02
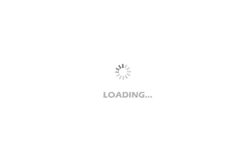
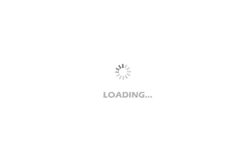
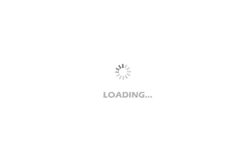
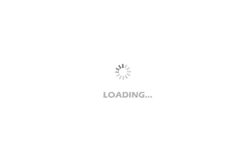
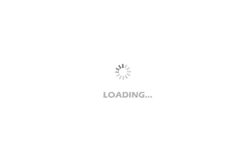
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- First day of the journey in Shenzhen
- Infrared thermal imager recommendation
- V853 Replace the startup LOGO
- The problem of the servo not rotating when the nodemcu esp8266 development board drives it
- Mentor Usage Problems
- Ethernet Problems
- If you want to know whether the signal generated by the signal generator or function generator is ready or whether it is input according to the set value standard...
- MicroPython precompiled firmware for TB-01 (W600)
- Is it necessary to attend Altium's offline training?
- How to prevent audio equipment from aging and make the sound better