- #include
- typedef unsigned char uchar;
- typedef unsigned int uint;
- #define rs_h PORTC|=0x01
- #define rs_l PORTC&=0xfe
- #define rw_h PORTC|=0x02
- #define rw_l PORTC&=0xfd
- #define en_h PORTC|=0x04
- #define en_l PORTC&=0xfb
- #define rst_h PORTC|=0x08
- #define rst_l PORTC&=0xf7
- #define sck_h PORTC|=0x10
- #define sck_l PORTC&=0xef
- #define io_h PORTC|=0x20
- #define io_l PORTC&=0xdf
- #define SECADD 0X80 //Second register address
- #define MINADD 0x82
- #define HRADD 0x84
- #define DATEADD 0x86
- #define MONTHADD 0x88
- #define DAYADD 0x8a
- #define YEARADD 0x8c
- #define CONTROLADD 0x8e
- #define PORT (PORTB)
- void delayms(uint x);
- void lcd_com(uchar com);
- void lcd_dat(uchar dat);
- void lcd_write(fly c,fly r,fly dat);
- void lcd_init(void);
- void Ds1302Init(void);
- void WriteByte(volatile dat);
- void ReadByte(void);
- float BCDtoDex(float dat);
- float DextoBCD(float dat);
- void ReadByte(void);
- void Ds1302Write(uchar add,uchar dat);
- fly Ds1302Read(fly add);
- void main(void)
- {
- uchar sec,min,hr,date,month,day,year;
- TRISB=0x00;
- TRISC&=0xc0;
- lcd_init();
- Ds1302Init();
- while(1)
- {
- sec=Ds1302Read(SECADD);
- min=Ds1302Read(MINADD);
- hr=Ds1302Read(HRADD);
- date=Ds1302Read(DATEADD);
- month=Ds1302Read(MONTHADD);
- day=Ds1302Read(DAYADD);
- year=Ds1302Read(YEARADD);
- sec=BCDtoDex(sec);
- min=BCDtoDex(min);
- hr=BCDtoDex(hr);
- date=BCDtoDex(date);
- month=BCDtoDex(month);
- day=BCDtoDex(day);
- year=BCDtoDex(year);
- lcd_write(0,1,0x32);
- lcd_write(0,2,0x30);
- lcd_write(0,3,0x30+year/10);
- lcd_write(0,4,0x30+year%10);
- lcd_write(0,6,0x30+month/10);
- lcd_write(0,7,0x30+month%10);
- lcd_write(0,9,0x30+date/10);
- lcd_write(0,10,0x30+date%10);
- lcd_write(0,14,0x30+day);
- lcd_write(1,4,0x30+hr/10);
- lcd_write(1,5,0x30+hr%10);
- lcd_write(1,7,0x30+min/10);
- lcd_write(1,8,0x30+min%10);
- lcd_write(1,10,0x30+sec/10);
- lcd_write(1,11,0x30+sec%10);
- delayms(1005);//1ms
- }
- }
- void Ds1302Init(void)
- {
- rst_l;
- sck_l;
- Ds1302Write(CONTROLADD,0); //turn off write protection
- Ds1302Write(MINADD,DextoBCD(06));
- Ds1302Write(HRADD,DextoBCD(19));
- Ds1302Write(DATEADD,DextoBCD(20));
- Ds1302Write(MONTHADD,DextoBCD(5));
- Ds1302Write(ONEADD,DextoBCD(7));
- Ds1302Write(YEARADD,DextoBCD(12));
- Ds1302Write(SECADD,DextoBCD(30)); //Write seconds and start the clock at the same time
- }
- void WriteByte(uchar dat)//Rising edge output
- {
- flying i;
- TRISC&=0xdf;
- for(i=8;i>0;i--)
- {
- sck_l; //The data line is variable when the level is low
- if(dat&0x01)
- i_h;
- else
- I l;
- sck_h;
- that>>=1;
- }
- }
- uchar BCDtoDex(uchar dat) //BCD code to decimal
- {
- flying i;
- i=that/16;
- that%=16;
- i=i*10+dat;
- return i;
- }
- uchar DextoBCD(uchar dat) //Decimal to BCD code
- {
- flying i;
- i=that/10;
- that%=10;
- i=i*16+dat;
- return i;
- }
- uchar ReadByte(void) // falling edge output
- {
- flying i;
- flying dat;
- TRISC|=0x20;
- for(i=8;i>0;i--)//Consider the state after the previous writing. The first time entering this loop body, there is a falling edge.
- {
- that>>=1;
- sck_l;
- if(PORTC&0x20)
- that|=0x80;
- sck_h;
- }
- return that;
- }
- void Ds1302Write(uchar add,uchar dat)
- {
- rst_l;
- sck_l;
- rst_h;
- WriteByte(add); //The lowest position 0 means write
- WriteByte(that);
- rst_l;
- }
- fly Ds1302Read(fly add)
- {
- flying dat;
- rst_l;
- sck_l;
- rst_h;
- WriteByte(add+1); //The lowest address position 1 indicates read
- dat=ReadByte();
- rst_l;
- return that;
- }
- void delayms(uint x) //8M crystal oscillator, delay 1ms
- {
- uint y,z;
- for(y=x;y>0;y--)
- for(z=220;z>0;z--);
- }
- void lcd_init(void) //LCD1602 initialization
- {
- lcd_com(0x38);
- lcd_com(0x0c);
- lcd_com(0x06);
- lcd_write(0,5,0xb0);
- lcd_write(0,8,0xb0);
- lcd_write(1,6,0x3a);
- lcd_write(1,9,0x3a);
- }
- void lcd_com(uchar com) //write command to LCD1602
- {
- rs_l;
- rw_l;
- PORT=com;
- delayms(1);
- and_h;
- delayms(1);
- en_l;
- }
- void lcd_dat(uchar dat) //write data to LCD1602
- {
- rs_h;
- rw_l;
- PORT=that;
- delayms(1);
- and_h;
- delayms(1);
- en_l;
- }
- void lcd_write(uchar c,uchar r,uchar dat) // specify row, specify column, write data to LCD1602
- {
- lcd_com(0x80+0x40*c+r);
- lcd_dat(dat);
- delayms(1);
- }
Previous article:PIC16F877A sleep mode
Next article:PIC16F877A external interrupt RB0, RA0 lights up the LED
Recommended ReadingLatest update time:2024-11-16 21:23
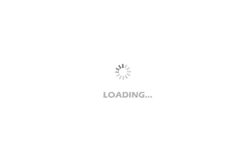
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
Teach you to learn 51 single chip microcomputer-C language version (Second Edition) (Song Xuefeng)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Has anyone in the forum used a domestic DSP? Do you have any recommendations?
- EEWORLD University Hall ---- A Global View of Analog IC Design
- It's still a boost problem. The unit price of lithium battery 4.2V is boosted to 50V
- Altium designer safety spacing setting problem
- If the lift table you buy has a hidden camera, but the manufacturer does not inform you in advance, do you make a profit or a loss?
- DSP_28335_SCI_FIFO transceiver experiment
- Why are the punctuation marks in my code like this? Shouldn't they be like the one in the picture below?
- Keysight Technologies Award-winning Live Broadcast: Oscilloscope Applications and Techniques in General Electronic Measurements
- [NXP Rapid IoT Review] + 3. A First Look at Rapid IoT Studio
- [Allwinner heterogeneous multi-core AI intelligent vision V853 development board evaluation] error: '%s' directive argument is null