//XY is the expected coordinate system (X:0-127, Y:0-63 in grids), Color 0 is off, 1 is on, 2 is flipped
void DrawPoint(unsigned char X,unsigned char Y,unsigned char Color)
{
unsigned char Row,Tier,Tier_bit ;
unsigned char ReadOldH,ReadOldL ;
wr_comm(0x34); // Select extended instructions and turn off display
wr_comm(0x36); // Select extended instructions, turn on display
Tier=X>>4 ; // Shift right 4 bits, that is, divide by 16 and round to the integer, to get the horizontal coordinate DX corresponding to the 12864 to be written
Tier_bit=X&0x0f ; // Get the number of the x in the DX (0-15), the high 4 bits of 0x0f are all 0,
// Bitwise AND eliminates the high 4 bits of X, which is equivalent to X%16, and obtains the number of bits in DX.
if(Y<32)
{
Row=Y ;
}
else //y coordinate is greater than 31, to display on the screen, DX also adds 8 accordingly
{
Row=Y-32 ;
Tier+=8 ;
}
wr_comm(Row+0x80); //Write (on the LCD) vertical coordinate (page coordinate)
wr_comm(Tier+0x80); //Write (on the LCD) horizontal coordinate (column coordinate)
ReadByte(); // Pre-
readReadOldH=ReadByte(); //Read the data in the GDRAM at this location, the upper 8
bitsReadOldL=ReadByte(); //low 8
bitswr_comm(Row+0x80);
wr_comm(Tier+0x80);
if(Tier_bit<8) //x is in the lower 8 bits in DX (according to the expected coordinates), but belongs to the upper 8 bits in the LCD internal coordinate system, corresponding to ReadOldH
{
switch(Color)
{
case 0 : ReadOldH&=(~(0x01<<(7-Tier_bit))); break ; //bit conversion LCD corresponding bit
case 1 : ReadOldH|=(0x01<<(7-Tier_bit)); break ; //set the specific position to 1 and light up
case 2 : ReadOldH^=(0x01<<(7-Tier_bit)); break ; //bitwise XOR flip specific bit
default : break ;
}
wr_data(ReadOldH); //write the upper 8 bits first
wr_data(ReadOldL);
}
else
{
switch(Color)
{
case 0 : ReadOldL&=(~(0x01<<(15-Tier_bit))); break ;
case 1 : ReadOldL|=(0x01<<(15-Tier_bit)); break ;
case 2 : ReadOldL^=(0x01<<(15-Tier_bit)); break ;
default : break ;
}
wr_data(ReadOldH);
wr_data(ReadOldL);
}
wr_comm(0x30); //Set the basic instruction set to turn off drawing display
}
//Draw horizontal line:
void DrawLineX( unsigned char X0, unsigned char X1, unsigned char Y, unsigned char Color )
{ unsigned char Temp ;
if( X0 > X1 )
{
Temp = X1 ;
X1 = X0 ;
X0 = Temp ;
}
for( ; X0 <= X1 ; X0++ )
DrawPoint( X0, Y, Color ) ;
}
//Draw vertical line:
void DrawLineY( unsigned char X, unsigned char Y0, unsigned char Y1, unsigned char Color )
{
unsigned char Temp ;
if( Y0 > Y1 )
{
Temp = Y1 ;
Y1 = Y0 ;
Y0 = Temp ;
}
for(; Y0 <= Y1 ; Y0++)
DrawPoint( X, Y0, Color) ;
}
//Use Bresenham line drawing algorithm
void DrawLine_any( unsigned char StartX, unsigned char StartY, unsigned char EndX, unsigned char EndY, unsigned char Color )
{
int t, distance; /*Change variable type according to screen size (such as int type)*/
int x = 0 , y = 0 , delta_x, delta_y ;
char incx, incy ;
delta_x = EndX - StartX ;
delta_y = EndY - StartY ;
if( delta_x > 0 )
{
incx = 1;
}
else if( delta_x == 0 )
{
DrawLineY ( StartX, Start delta_x > delta_y ) { distance = delta_x ; } else { distance = delta_y ; } DrawPoint (
StartX , StartY , Color ) ; /* Draw Line*/ for ( t = 0 ; t < = distance + 1 ; t++ ) { DrawPoint( StartX, StartY , Color ) ; x + = delta_x ; y + = delta_y ; if( x > distance ) { x -= distance ; StartX += incx ; } if( y > distance ) { y -= distance ; StartY += incy ; } } }
Previous article:Design of cable joint temperature monitoring system terminal based on wireless technology
Next article:51 MCU drives PS2 keyboard complete program
Recommended ReadingLatest update time:2024-11-17 00:54
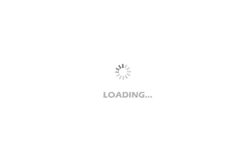
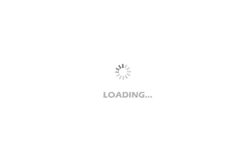
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Geely Auto Recruitment Electronic Reliability Design Engineer
- Correct processing of ADC input circuit (reprinted)
- Using a fuel gauge chip to achieve fast and intelligent charging of dual-series lithium batteries
- Here is a disassembly picture of ASUS Mars15 VX60GT9750
- TI blog post: Battery test equipment signal chain
- Can LDO always work at low voltage?
- STM32F767IGT6 core board schematic diagram
- DSP product development process steps
- 【RPi PICO】Use PIO to get more Sigma Delta ADC inputs
- Questions about STLINK programmer