Times have changed and floppy drives are no longer useful, but the motor inside is quite interesting, so I took it out and studied it.
/*Use mega32 three port lines to drive the floppy drive motor.
PA2 connects to LB1833 ENA1\2 pin;
PA1 connects to LB1833 IN1 pin;
PA0 connects to LB1833 IN2 pin.
****************************************************** /
//ICC- AVR application builder : 2005-5-20 10:40:30
// Target : M32
// Crystal: 3.6864Mhz
#include
#include
unsigned char np;
//Stepper motor operation data table
const unsigned char motortb[]={0x05,0x07,0x06,0x04,0x05,0x07,0x06,0x04};
void delay ( unsigned char t );// Subroutine for each step delay
void a_step( unsigned char d, unsigned char t );//Stepper motor takes one step d=0 forward d=1 reverse
void port_init( void )
{
PORTA = 0x00;
DDRA = 0xFF;
PORTB = 0xFF;
DDRB = 0x00;
PORTC = 0xFF;
DDRC = 0x00;
PORTD = 0xFF;
DDRD = 0x00;
}
//call this routine to initialise all peripherals
void init_devices( void )
{
//stop errant interrupts until set up
CLI(); //disable all interrupts
port_init();
MCUCR = 0x00;
GICR = 0x00;
TIMSK = 0x00; //timer interrupt sources
SEI(); //re-enable interrupts
//all peripherals are now initialised
}
void delay ( unsigned char t )// Subroutine for each step delay
{
unsigned char i;
unsigned int j;
for (i = 0 ; i < t ; i++ )
{
for ( j = 0 ;j < 800 ; j++ )
;
}
}
void a_step ( unsigned char d, unsigned char t) //Stepper motor takes one step d=0 forward d=1 reverse t // The bigger the t, the slower it goes
{
if ( d & 0x01 )
{
if ( np == 0 )
{
np = 7;
}
else
{
np--;
}
}
else
{
if ( np == 7 )
{
np = 0;
}
else
{
np++;
}
}
PORTA = motortb[np];
delay(t);
}
void a_turn (unsigned char d, unsigned char t)// The stepper motor makes one turn
{
unsigned char i;
for ( i = 0 ; i < 96 ; i++ )
{
a_step ( d, t );
}
}
void main ( void )
{
n p = 4;
while (1)
{
a_turn (1, 1);
}
}
Keywords:AVR
Reference address:AVR drive floppy drive motor program
CODE:
/*Use mega32 three port lines to drive the floppy drive motor.
PA2 connects to LB1833 ENA1\2 pin;
PA1 connects to LB1833 IN1 pin;
PA0 connects to LB1833 IN2 pin.
****************************************************** /
//ICC- AVR application builder : 2005-5-20 10:40:30
// Target : M32
// Crystal: 3.6864Mhz
#include
#include
unsigned char np;
//Stepper motor operation data table
const unsigned char motortb[]={0x05,0x07,0x06,0x04,0x05,0x07,0x06,0x04};
void delay ( unsigned char t );// Subroutine for each step delay
void a_step( unsigned char d, unsigned char t );//Stepper motor takes one step d=0 forward d=1 reverse
void port_init( void )
{
PORTA = 0x00;
DDRA = 0xFF;
PORTB = 0xFF;
DDRB = 0x00;
PORTC = 0xFF;
DDRC = 0x00;
PORTD = 0xFF;
DDRD = 0x00;
}
//call this routine to initialise all peripherals
void init_devices( void )
{
//stop errant interrupts until set up
CLI(); //disable all interrupts
port_init();
MCUCR = 0x00;
GICR = 0x00;
TIMSK = 0x00; //timer interrupt sources
SEI(); //re-enable interrupts
//all peripherals are now initialised
}
void delay ( unsigned char t )// Subroutine for each step delay
{
unsigned char i;
unsigned int j;
for (i = 0 ; i < t ; i++ )
{
for ( j = 0 ;j < 800 ; j++ )
;
}
}
void a_step ( unsigned char d, unsigned char t) //Stepper motor takes one step d=0 forward d=1 reverse t // The bigger the t, the slower it goes
{
if ( d & 0x01 )
{
if ( np == 0 )
{
np = 7;
}
else
{
np--;
}
}
else
{
if ( np == 7 )
{
np = 0;
}
else
{
np++;
}
}
PORTA = motortb[np];
delay(t);
}
void a_turn (unsigned char d, unsigned char t)// The stepper motor makes one turn
{
unsigned char i;
for ( i = 0 ; i < 96 ; i++ )
{
a_step ( d, t );
}
}
void main ( void )
{
n p = 4;
while (1)
{
a_turn (1, 1);
}
}
Previous article:Fast PWM of T0
Next article:Infrared remote control decoding program RC5 decoding
Recommended ReadingLatest update time:2024-11-16 20:30
AVR microcontroller I2C bus experiment
AVR MCU I2C bus experiment.
1. Use 24C02 to record the number of CPU startups and display it on the PB port.
2. Internal 1 M crystal oscillator, program uses single task mode, software delay.
3. For this experiment, please plug in all 8 short-circuit blocks of JP1 and JP7 (LED_EN)/PC0/PC1 short-circuit b
[Microcontroller]
AVR IO output digital tube scanning program
System functions Use AVR to scan four digital tubes, dynamic scanning, dynamic display, left scan, right scan, back and forth scan... hardware design AVR main control circuit schematic diagram Digital tube dynamic scanning circuit schematic diagram software design The following part is copied from TXT and
[Microcontroller]
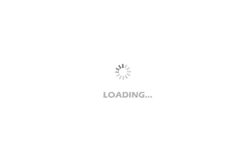
The difference and use of AVR's INT external interrupt and PCINT interrupt
INT external interrupt is available in almost all general-purpose microcontrollers and embedded computers. The early 51 series and arm series also have it. However, due to design reasons, most of them only have two INTs. Recently, someone mentioned that "all ports can have external interrupts". Because I was skeptical
[Microcontroller]
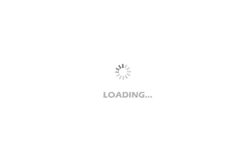
Active crystal oscillator recovery method to solve the AVR microcontroller fuse lock problem
Many AVR microcontroller beginners may easily make mistakes in the fuse bits when using AVR microcontrollers, causing the microcontroller to be locked. For example, after JTAGEN is set to 1, the JTAG of the microcontroller can no longer download programs into it, which brings us a lot of trouble.
A common recovery
[Microcontroller]
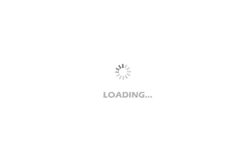
Lighting and CO2 control system for watermelon greenhouse production based on AVR
1. Project Overview
1.1 Introduction
A greenhouse is a place that can change the growth environment of plants, create optimal conditions for plant growth, and avoid the influence of seasonal changes and bad climate on them. It uses light-collecting covering materials as all or part of the structural materials, and
[Microcontroller]
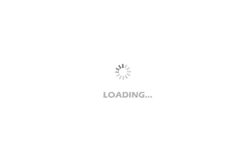
Analysis of EEPROM Reading and Writing of AVR Microcontroller
Introduction: This article introduces the time problem of reading and writing data in EEPROM of AVR microcontroller, and analyzes the advantages and disadvantages of three methods. Since the AVR EEPROM write cycle is relatively long (usually in milliseconds), special attention should be paid during programming. Ther
[Microcontroller]
AVR MCU fuse configuration and related solutions
Users can configure the fuse bits of AVR using parallel programming, ISP programming, and JTAG programming, but different programming tools provide different ways to configure the fuse bits (referring to the human-machine interface). Some are by directly filling in the fuse bit values (such as: CVAVR, PonyProg2000 a
[Microcontroller]
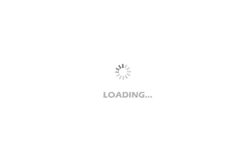
AVR review notes--IIC read and write multiple 24cxxx
In fact, I just backed up some code and added some comments, because it is easy and there is nothing much to say. . . First define some things #define PORT_IIC PORTC #define DDR_IIC DDRC #define BIT_SCL 0 #define BIT_SDA 1 #define TW_START 0X08 #define TW_REP_START 0X10 #define TW_MT_SLA_ACK 0X18 Let's get to the po
[Microcontroller]
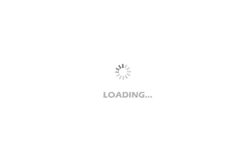
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Metronom Real-Time Operating System RTOS for AVR microcontrollers
-
Learn C language for AVR microcontrollers easily (with video tutorial) (Yan Yu, Li Jia, Qin Wenhai)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Recommended Content
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
MoreDaily News
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
Guess you like
- [Automatic clock-in walking timer system based on face recognition] Unboxing (1): SiPEED Maix Bit (K210)
- Learn more about the application of Xilinx FPGA general-purpose IP cores in communications
- MSP430__SD card FAT16 reading and writing program based on MSP430 learning and development system
- Compile error 'OCDROM' definition out of range
- Crazy Shell AI open source drone SPI (barometer data acquisition)
- Recommend a chip for analog video signal equalization
- Level conversion between 3.3V and 5V
- China successfully develops 5G millimeter wave chip
- DFRobot AS7341 visible light sensor review event is coming soon, come here to see the real thing first! !
- RSL10-SENSE-DB-GEVK Testing