#ifndef _RTC_TIME_H_
#define _RTC_TIME_H_
#include
extern struct tm Time_ConvUnixToCalendar(time_t t);
extern time_t Time_ConvCalendarToUnix(struct tm t);
extern time_t Time_GetUnixTime(void);
extern struct tm Time_GetCalendarTime(void);
extern void Time_SetUnixTime(time_t);
extern void Time_SetCalendarTime(struct tm t);
#endif
/*******************************************************************************
* This file implements the date function based on RTC, providing reading and writing of year, month and day. (Based on ANSI-C's time.h)
*
* Author: jjldc (九九)
* QQ: 77058617
*
* The time format saved in RTC is the UNIX timestamp format. That is, a 32-bit time_t variable (actually u32)
*
* The ANSI-C standard library provides two data types to represent time:
* time_t: UNIX timestamp (the number of seconds since 1970-1-1 to a certain time)
* typedef unsigned int time_t;
*
* struct tm: Calendar format (year-month-day format)
* The tm structure is as follows:
* struct tm {
* int tm_sec; // seconds after the minute, 0 to 60
* (0 - 60 allows for the occasional leap second)
* int tm_min; // minutes after the hour, 0 to 59
* int tm_hour; // hours since midnight, 0 to 23
* int tm_mday; // day of the month, 1 to 31
* int tm_mon; // months since January, 0 to 11
* int tm_year; // years since 1900
* int tm_wday; // days since Sunday, 0 to 6
* int tm_yday; // days since January 1, 0 to 365
* int tm_isdst; // Daylight Savings Time flag
* ...
* }
* wday and yday can be automatically generated, and the software can directly read
* the value of mon is 0-11
* ***Note***:
* tm_year: defined in the time.h library as the year since 1900, that is, 2008 should be expressed as 2008-1900=108
* This representation method is not very user-friendly and is quite different from reality.
* Therefore, this difference is blocked in this document.
* That is, when the functions of this file are called externally, the date of the tm structure type, tm_year is 2008
* Note: If you want to call the functions in the system library time.c, you need to set tm_year-=1900 yourself
*
* Member function description:
* struct tm Time_ConvUnixToCalendar(time_t t);
* Input a Unix timestamp (time_t) and return a date in Calendar format
* time_t Time_ConvCalendarToUnix(struct tm t);
* Input a date in Calendar format and return a Unix timestamp (time_t)
* time_t Time_GetUnixTime(void);
* Get the Unix timestamp value of the current time from the RTC
* struct tm Time_GetCalendarTime(void);
* Get the calendar time of the current time from the RTC
* void Time_SetUnixTime(time_t);
* Input a time in UNIX timestamp format and set it to the current RTC time
* void Time_SetCalendarTime(struct tm t);
* Enter the time in Calendar format and set it to the current RTC time
*
* External call example:
* Define a date variable in Calendar format:
* struct tm now;
* now.tm_year = 2008;
* now.tm_mon = 11; //December
* now.tm_mday = 20;
* now.tm_hour = 20;
* now.tm_min = 12;
* now.tm_sec = 30;
*
* Get the current date and time:
* tm_now = Time_GetCalendarTime();
* Then you can directly read tm_now.tm_wday to get the week number
*
* Set the time:
* Step1. tm_now.xxx = xxxxxxxxx;
* Step2. Time_SetCalendarTime(tm_now);
*
* Calculate the difference between two times
* struct tm t1,t2;
* t1_t = Time_ConvCalendarToUnix(t1);
* t2_t = Time_ConvCalendarToUnix(t2);
* dt = t1_t - t2_t;
* dt is the number of seconds between the two times
void SetTime(u32 t); /* Private functions ---------------------------------------------------------*/ /* Private define ------------------------------------------------------------*/ /* Private macro -------------------------------------------------------------*/ /* Private variables ---------------------------------------------------------*/
/* Private function prototypes ---------------------------------------------------------------*/ void SetTime(u32 t); /* Private functions ---------------------------------------------------------
*/
/*
Private
define
------------------------------------------------------------*/ / * Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/ /* Private function prototypes ---------------------------------------------------------------*/ void SetTime(u32 t); /* Private functions ---------------------------------------------------------*/ /* Private define ------------------------------------------------------------*/ /* Private macro -------------------------------------------------------------*/ /* Private variables
-------------------------------------------------------------
*
/
/
*
Private function
prototypes
--------------------------------------------------------------- */ Time_ConvUnixToCalendar(time_t t)
* Description : Convert UNIX timestamp to calendar time
* Input : u32 t current UNIX timestamp
* Output : None
* Return : struct tm
******************************************************************************/
struct tm Time_ConvUnixToCalendar(time_t t)
{
struct tm *t_tm;
t_tm = localtime(&t);
t_tm->tm_year += 1900; //the tm_year of the localtime conversion result is a relative value and needs to be converted to an absolute valuereturn
*t_tm;
}
/***********************************************************************************
* Function Name : Time_ConvCalendarToUnix(struct tm t)
* Description : Write the current time of the RTC clock
* Input : struct tm t
* Output : None
* Return : time_t
******************************************************************************/
time_t Time_ConvCalendarToUnix(struct tm t)
{
t.tm_year -= 1900; //The year stored in the external tm structure is in 2008 format
. //The year format defined in time.h is the year starting from 1900.
//So, this factor should be taken into account when converting dates.
return mktime(&t);
}
/***********************************************************************************
* Function Name : Time_GetUnixTime()
* Description : Get the current Unix timestamp value from RTC
* Input : None
* Output : None
* Return : time_t t
*******************************************************************************/
time_t Time_GetUnixTime(void)
{
return (time_t)RTC_GetCounter();
}
/***********************************************************************************
* Function Name : Time_GetCalendarTime()
* Description : Get the current calendar time (struct tm) from RTC
* Input: None
* Output: None
* Return: time_t t
************************************* ******************************************/
struct tm Time_GetCalendarTime(void)
{
time_t t_t;
struct tm t_tm;
t_t = (time_t)RTC_GetCounter();
t_tm = Time_ConvUnixToCalendar(t_t);
return t_tm;
}
/********************** *************************************************** *******
* Function Name: Time_SetUnixTime()
* Description: Write the given Unix timestamp to RTC
* Input: time_t t
* Output: None
* Return: None
********** *************************************************** *******************/
void Time_SetUnixTime(time_t t)
{
PWR_BackupAccessCmd(ENABLE);
RTC_WaitForLastTask();
RTC_SetCounter((u32)t);
RTC_WaitForLastTask();
PWR_BackupAccessCmd(DISABLE);
return;
}
/************************* ************************************************** ****
* Function Name : Time_SetCalendarTime()
* Description : Convert the given Calendar format time into UNIX timestamp and write it to RTC
* Input : struct tm t
* Output : None
* Return : None
******* ************************************************** **********************/
void Time_SetCalendarTime(struct tm t)
{
Time_SetUnixTime(Time_ConvCalendarToUnix(t));
return;
}
Previous article:USART sends and receives interrupts without intentional nesting
Next article:stm32 stack setting size causes error
Recommended ReadingLatest update time:2024-11-16 13:40
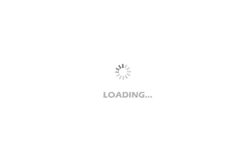
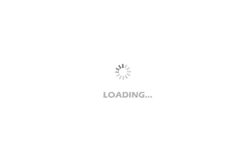
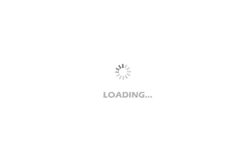
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Collection of popular domestic chip data downloads
- LIS25BA Bone Vibration Sensor Adapter Board Related Information
- [CC1352P Review] A brief analysis of the operation process of rfEasyLinkTx
- DIY IP5306 power bank, the discharge current is too small (only 300ma) (with schematic diagram)
- [RVB2601 Creative Application Development] Build CDK Development Environment
- This strange cut made the PCB hang inexplicably
- It seems that I have a good relationship with Shide——Part 2
- 【Silicon Labs BG22-EK4108A Bluetooth Development Review】I. Hardware Appreciation and Development Environment Introduction
- [ESP32-S2-Kaluga-1 Review] 4. LCD example compilation pitfalls
- HuaDa MCU FLASH operation instructions and precautions