//Function pin definition
//#define A0 BIT(PC6) //Data 1/Command 0 selection
sbit A0 = P1^4;
//#define WR BIT(PC5) //Read 1/Write 0
sbit _WR = P1^3;
//#define E1 BIT(PC4) //Chip select 1(Master)
sbit E1 = P1^1;
//#define E2 BIT(PC7) //Chip select 2(slave)
sbit E2 = P1^2;
//#define lcd_data PORTA //Data
#define lcd_data P0
//Common operation macro definitions
#define set_E1() (E1=1) //1 chip select M
#define set_E2() (E2=1) //1 chip select S
#define set_A0() (A0=1) //1 data
#define set_WR() (_WR=1) //1 read
#define clr_E1() (E1=0) //0
#define clr_E2() (E2=0) //0
#define clr_A0() (A0=0) //0命令
#define clr_WR() (_WR=0) //0写
//LCD display control command table
#define disp_off 0xAE //Display off
#define disp_on 0xAF //Display on
#define disp_start_line 0xC0 //Display start address (last 5 bits - represents rows 0-31)
#define page_addr_set 0xB8 //Page address setting (0~3)
#define col_addr_set 0x00 //Column address setting (0~61)
#define status_busy 0x80 //0=ready
#define mode_write 0xEE //Write mode
#define dynamic_driver 0xA4 //Dynamic driver
#define adc_select 0xA0 //clockwise
#define clk32 0xA9 //Refresh clock setting 1/32
#define clk16 0xA8 //Refresh clock setting 1/16
#define reset 0xE2 //Software reset
////The new driver focuses on simplifying the code, so the externally callable functions are: system initialization, screen clearing, busy flag////
//// ASCII and Chinese character mixed output function, RAM buffer data display output (generally used for outputting numbers)/////////
#include
#include
#define uchar unsigned char
#define uint unsigned int
#define NOP() _nop_();
uchar dot_buffer[32]; //dot matrix buffer
uchar disp_buffer[4]; //RAM data display buffer
void lcd_init(void); //LCD initialization
void lcd_clr(void); //LCD clear screen
void wait_ready(void); //Wait for ready
void draw_bmp(uchar col,uchar layer,uchar width,uchar *bmp);
//Dot matrix code display output
void disp_one_ascii(uchar col,uchar layer,uchar ascii_code,uchar mode);
//Single ASCII code output (ascii_code is ASCII code)
void disp_ram_data(uchar col,uchar layer,uchar n,uchar mode);
//RAM data (digital) display output
void dprintf(uchar col,uchar layer,uchar *buf,uchar mode);
//General mixed string display
typedef struct data_gb16 //Chinese character font data structure
{
uchar index[2];
uchar zimo[32];
};
struct data_gb16 code hz16[] =
{
"延", 0x00,0x84,0xC4,0xA4,0x94,0x8C,0x00,0xE4,
0x04,0x04,0xFC,0x42,0x63,0x42,0x00,0x00,
0x80,0x44,0x28,0x10,0x2C,0x43,0x40,0x4F,
0x48,0x48,0x4F,0x48,0x4C,0x68,0x20,0x00,
"时", 0x00,0xFC,0x84,0x84,0x84,0xFE,0x14,0x10,
0x90,0x10,0x10,0x10,0xFF,0x10,0x10,0x00,
0x00,0x3F,0x10,0x10,0x10,0x3F,0x00,0x00,
0x00,0x23,0x40,0x80,0x7F,0x00,0x00,0x00
};
uchar code ascii[] = {
//前面0x20(32个)ASCII码为控制命令 //本程序中不用
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,//
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x38,0xFC,0xFC,0x38,0x00,0x00,//!
0x00,0x00,0x00,0x0D,0x0D,0x00,0x00,0x00,
0x00,0x0E,0x1E,0x00,0x00,0x1E,0x0E,0x00,//"
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x20,0xF8,0xF8,0x20,0xF8,0xF8,0x20,0x00,//#
0x02,0x0F,0x0F,0x02,0x0F,0x0F,0x02,0x 00, 0x38,0x7C
,0x44,0x47,0x47,0xCC,0x98,0x00,//$
0x03,0x06,0x04,0x1C,0x1C,0x07,0x03,0x00,
0x30,0x30,0x00,0x80,0xC0,0x60,0x30,0x00,//%
0x0C,0x06,0x03,0x01,0x00,0x0C,0x0C,0x00,
0x80,0xD8,0x7C,0xE4,0xBC,0xD8,0x40,0x0 0,//&
0x07,0x0F,0x08,0x08,0x07,0x0F,0x08,0x00,
0x00,0x10,0x1E,0x0E,0x00,0x00,0x00,0x00,//'
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0xF0,0xF8,0x0C,0x04,0x00,0x00,//(
0x00,0x00,0x03,0x07,0x0C,0x08,0x00,0x 00,
0x00,0x00,0x04,0x0C,0xF8,0xF0,0x00,0x00,//)
0x00,0x00,0x08,0x0C,0x07,0x03,0x00,0x00,
0x80,0xA0,0xE0,0xC0,0xC0,0xE0,0xA0,0x80,//*
0x00,0x02,0x03,0x01,0x01,0x03,0x02,0x00,
0x00,0x80,0x80,0xE0,0xE0,0x80,0x80,0x 00,//+
0x00,0x00,0x00,0x03,0x03,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,//,
0x00,0x00,0x10,0x1E,0x0E,0x00,0x00,0x00,
0x80,0x80,0x80,0x80,0x80,0x80,0x80,0x00,//-
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x 00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,//.
0x00,0x00,0x00,0x0C,0x0C,0x00,0x00,0x00,
0x00,0x00,0x00,0x80,0xC0,0x60,0x30,0x00,///
0x0C,0x06,0x03,0x01,0x00,0x00,0x00,0x00,
0xF8,0xFC,0x04,0xC4,0x24,0xFC,0xF8,0x00 ,//0
0x07,0x0F,0x09,0x08,0x08,0x0F,0x07,0x00,
0x00,0x10,0x18,0xFC,0xFC,0x00,0x00,0x00,//1
0x00,0x08,0x08,0x0F,0x0F,0x08,0x08,0x00,
0x08,0x0C,0x84,0xC4,0x64,0x3C,0x18,0x00,//2
0x0E,0x0F,0x09,0x08,0x08,0x0C,0x0C,0x 00,
0x08,0x0C,0x44,0x44,0x44,0xFC,0xB8,0x00,//3
0x04,0x0C,0x08,0x08,0x08,0x0F,0x07,0x00,
0xC0,0xE0,0xB0,0x98,0xFC,0xFC,0x80,0x00,//4
0x00,0x00,0x00,0x08,0x0F,0x0F,0x08,0x00,
0x7C,0x7C,0x44,0x44,0xC4,0xC4,0x84,0x00 ,//5
0x04,0x0C,0x08,0x08,0x08,0x0F,0x07,0x00,
0xF0,0xF8,0x4C,0x44,0x44,0xC0,0x80,0x00,//6
0x07,0x0F,0x08,0x08,0x08,0x0F,0x07,0x00,
0x0C,0x0C,0x04,0x84,0xC4,0x7C,0x3C,0x00,//7
0x00,0x00,0x0F,0x0F,0x00,0x00,0x00,0x 00,
0xB8,0xFC,0x44,0x44,0x44,0xFC,0xB8,0x00,//8
0x07,0x0F,0x08,0x08,0x08,0x0F,0x07,0x00,
0x38,0x7C,0x44,0x44,0x44,0xFC,0xF8,0x00,//9
0x00,0x08,0x08,0x08,0x0C,0x07,0x03,0x00,
0x00,0x00,0x00,0x30,0x30,0x00,0x00,0x00,//:
0x00,0x00,0x00,0x06,0x06,0x00,0x00,0x00,
0x00,0x00,0x00,0x30,0x30,0x00,0x00,0x00,//;
0x00,0x00,0x08,0x0E,0x06,0x00,0x00,0x00,
0x00,0x80,0xC0,0x60,0x30,0x18,0x08,0x00,//<
0x00,0x00,0x01,0x03,0x06,0x0C,0x08,0x00,
0x40,0x40,0x40,0x40,0x40,0x40,0x40,0x00,//=
0x02,0x02,0x02,0x02,0x02,0x02,0x02,0x00,
0x00,0x08,0x18,0x30,0x60,0xC0,0x80,0x00,//>
0x00,0x08,0x0C,0x06,0x03,0x01,0x00,0x00,
0x18,0x1C,0x04,0xC4,0xE4,0x3C,0x18,0x00,//?
0x00,0x00,0x00,0x0D,0x0D,0x00,0x00,0x00,
0xF0,0xF8,0x08,0xC8,0xC8,0xF8,0xF0,0x00,//@
0x07,0x0F,0x08,0x0B,0x0B,0x0B,0x01,0x00,
0xE0,0xF0,0x98,0x8C,0x98,0xF0,0xE0,0x00,//A
0x0F,0x0F,0x00,0x00,0x00,0x0F,0x0F,0x00,
0x04,0xFC,0xFC,0x44,0x44,0xFC,0xB8,0x00,//B
0x08,0x0F,0x0F,0x08,0x08,0x0F,0x07,0x00,
0xF0,0xF8,0x0C,0x04,0x04,0x0C,0x18,0x00,//C
0x03,0x07,0x0C,0x08,0x08,0x0C,0x06,0x00,
0x04,0xFC,0xFC,0x04,0x0C,0xF8,0xF0,0x00,//D
0x08,0x0F,0x0F,0x08,0x0C,0x07,0x03,0x00,
0x04,0xFC,0xFC,0x44,0xE4,0x0C,0x1C,0x00,//E
0x08,0x0F,0x0F,0x08,0x08,0x0C,0x0E,0x00,
0x04,0xFC,0xFC,0x44,0xE4,0x0C,0x1C,0x00,//F
0x08,0x0F,0x0F,0x08,0x00,0x00,0x00,0x00,
0xF0,0xF8,0x0C,0x84,0x84,0x8C,0x98,0x00,//G
0x03,0x07,0x0C,0x08,0x08,0x07,0x0F,0x00,
0xFC,0xFC,0x40,0x40,0x40,0xFC,0xFC,0x00,//H
0x0F,0x0F,0x00,0x00,0x00,0x0F,0x0F,0x00,
0x00,0x00,0x04,0xFC,0xFC,0x04,0x00,0x00,//I
0x00,0x00,0x08,0x0F,0x0F,0x08,0x00,0x00,
0x00,0x00,0x00,0x04,0xFC,0xFC,0x04,0x00,//J
0x07,0x0F,0x08,0x08,0x0F,0x07,0x00,0x00,
0x04,0xFC,0xFC,0xC0,0xF0,0x3C,0x0C,0x00,//K
0x08,0x0F,0x0F,0x00,0x01,0x0F,0x0E,0x00,
0x04,0xFC,0xFC,0x04,0x00,0x00,0x00,0x00,//L
0x08,0x0F,0x0F,0x08,0x08,0x0C,0x0E,0x00,
0xFC,0xFC,0x38,0x70,0x38,0xFC,0xFC,0x00,//M
0x0F,0x0F,0x00,0x00,0x00,0x0F,0x0F,0x00,
0xFC,0xFC,0x38,0x70,0xE0,0xFC,0xFC,0x00,//N
0x0F,0x0F,0x00,0x00,0x00,0x0F,0x0F,0x00,
0xF0,0xF8,0x0C,0x04,0x0C,0xF8,0xF0,0x00,//O
0x03,0x07,0x0C,0x08,0x0C,0x07,0x03,0x00,
0x04,0xFC,0xFC,0x44,0x44,0x7C,0x38,0x00,//P
0x08,0x0F,0x0F,0x08,0x00,0x00,0x00,0x00,
0xF8,0xFC,0x04,0x04,0x04,0xFC,0xF8,0x00,//Q
0x07,0x0F,0x08,0x0E,0x3C,0x3F,0x27,0x00,
0x04,0xFC,0xFC,0x44,0xC4,0xFC,0x38,0x00,//R
0x08,0x0F,0x0F,0x00,0x00,0x0F,0x0F,0x00,
0x18,0x3C,0x64,0x44,0xC4,0x9C,0x18,0x00,//S
0x06,0x0E,0x08,0x08,0x08,0x0F,0x07,0x00,
0x00,0x1C,0x0C,0xFC,0xFC,0x0C,0x1C,0x00,//T
0x00,0x00,0x08,0x0F,0x0F,0x08,0x00,0x00,
0xFC,0xFC,0x00,0x00,0x00,0xFC,0xFC,0x00,//U
0x07,0x0F,0x08,0x08,0x08,0x0F,0x07,0x00,
0xFC,0xFC,0x00,0x00,0x00,0xFC,0xFC,0x00 ,//V
0x01,0x03,0x06,0x0C,0x06,0x03,0x01,0x00,
0xFC,0xFC,0x00,0x80,0x00,0xFC,0xFC,0x00,//W
0x03,0x0F,0x0E,0x03,0x0E,0x0F ,0x03,0x00,
0x0C,0x3C,0xF0,0xC0,0xF0,0x3C,0x0C,0x00,//X
0x0C,0x0F,0x03,0x00,0x03,0x0F,0x0C,0x00,
0x00,0x3C,0x7C,0xC0,0xC0,0x7C,0x3C,0x 00 ,//Y
0x00,0x00,0x08,0x0F,0x0F,0x08,0x00,0x00,
0x1C,0x0C,0x84,0xC4,0x64,0x3C,0x1C,0x00,//Z
0x0E,0x0F,0x09,0x08,0x08,0x0C,0x0E,0x00,
0x80,0x80,0x80,0x80,0xe0,0xC0,0x80,0x00,//->0x5b (custom display characters)
0x01,0x01,0x01,0x01 ,0x07,0x03,0x01,0x00
//Other custom characters can be added starting from 0x5c
};
///
...
//instruction
clr_WR(); //write trigger
NOP();
lcd_data = instruction; //instruction code
NOP();
clr_E1(); //off M
}
///
...
//Write trigger
NOP();
lcd_data = c; //Data
NOP();
clr_E1(); //Close M
}
///
...
//instruction
clr_WR(); //write trigger
NOP();
lcd_data=instruction; //instruction code
NOP();
clr_E2(); //off
}
///
...
= c;
NOP();
clr_E2();
}
///
...
DDRA = 0xff; //Reset PA port output
}
///
...
send_mi(disp_off); //Turn off the display
send_si(disp_off);
send_mi(dynamic_driver); //Dynamic driver
send_si(dynamic_driver);
send_mi(clk32); //1/32 duty cycle
send_si(clk32);
send_mi(adc_select); //clockwise
send_si(adc_select);
send_mi(mode_write); //Write mode
send_si(mode_write);
send_mi(col_addr_set);
send_mi(disp_start_line); //Return to zero column, set the display start line
send_si(col_addr_set);
send_si(disp_start_line);
send_mi(disp_on); //Turn on display
send_si(disp_on);
}
///
...
//Set the main window to 0 columns
send_si(0); //Set the slave window to 0 columns
for (i=0;i<62;i++)
{
send_md(0x00);
send_sd(0x00);
}
}
}
///
...
///
...
///
...
///
...
///
...
// col The starting position of the graphic 0~121
// layer The position of the graphic (Y coordinate) 0-lower half, not 0-upper half
// width Graphic width 8, 16 optional
// bmp graphic data pointer
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////
void draw_bmp(uchar col,uchar layer,uchar width,uchar *bmp)
{
uchar x;
uchar address; //address represents the physical address of the video memory
uchar p=0;
uchar page=0;
uchar window=0; //page represents the upper and lower pages, window represents the left and right windows (0 left, 1 right)
if (layer) page=2; //Left-main window, right-slave windowfor
(x=col; x
if (x>121)return; //Prevent garbled display
if (x>60) //Left and right window positioning
{
window=1; //Right-slave window
address=x%61;
}
else
address=x; //Main window output
set_page(page); //upper layer data output
set_address(address);
if (window)
putchar_r(bmp[p]);
else
putchar_l(bmp[p]);
set_page(page+1); //lower layer data output
set_address(address); //columns remain unchanged
if (window)
putchar_r(bmp[p+width]);
else
putchar_l(bmp[p+width]);
p++;
}
}
///
...
layer,uchar ascii_code,uchar mode)
{
uchar i;
for(i=0;i<16;i++) //ASCII code display occupies 16 bytes
{
if(mode)dot_buffer[i]=~ascii[(ascii_code-0x20)*16 + i];
else dot_buffer[i]= ascii[(ascii_code-0x20)*16 + i];
}
draw_bmp(col ,layer,8,dot_buffer);
}
///
...
if(mode)disp_one_ascii(col,layer,disp_buffer[n]+0x30,1);
else disp_one_ascii(col,layer,disp_buffer[n]+0x30,0);
col += 8;
}
}
////////////////////////////////////////////////////// ////////////////////////////////
//Function: void dprintf(uchar col,uchar layer,uchar *ptr,uchar mode)
//Description: ASCII (8*16) and Chinese characters (16*16) display function
///////////////////////////////// ////////////////////////////////////////////////////
void dprintf(uchar col,uchar layer,uchar *ptr,uchar mode)
{
uchar c1,c2;
uchar i,j,k;
uchar ulen;
//uchar ucol,ulayer,umode;
uchar ucol,ulayer;
ulen = 0;
ucol = col;
ulayer = layer;
while (ptr[ulen]!= 0)ulen++; //detection String length
i=0;
while(i
c1 = ptr[i];
c2 = ptr[i+1];
//The difference between ASCII characters and Chinese characters is that 128 is the delimiter; characters greater than 128 are Chinese characters
if(c1 <=128) //ASCII
{
if(mode)disp_one_ascii(ucol,ulayer,c1,1);
else disp_one_ascii(ucol,ulayer,c1,0);
ucol+=8;
i++; //ASCII code Processing
}
else //Chinese
{
for(j=0;j
if((c1 == hz16[j].index[0]) && (c2 == hz16[j].index[1]))
break;
}
for(k=0 ;k<32;k++)
{ if(mode)dot_buffer[k]=~hz16[j].zimo[k];
else dot_buffer[k]= hz16[j].zimo[k];
}
draw_bmp(ucol,ulayer ,16,dot_buffer);
ucol+=16;
i+=2; //Chinese processing
}
}
}
///
...
for(i=0;i<10;i++)for(j=1;j;j++); //Power-on delay
lcd_init();
lcd_clr();
dprintf(10,1,"I AM CHINESE!",0); //Description/Display from the 10th column in the up row/Normal display
disp_ram_data(10,0,2,1); //Description/Display from the 10th column in the down row/Reverse display
while(1);
}
Previous article:12232F serial port display program
Next article:Summary of 51 MCU IO ports
Recommended ReadingLatest update time:2024-11-16 13:23
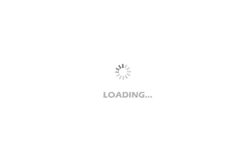
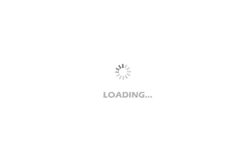
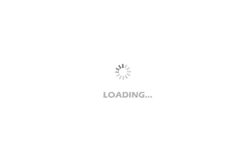
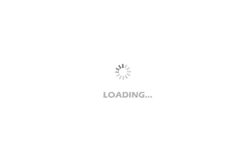
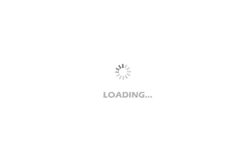
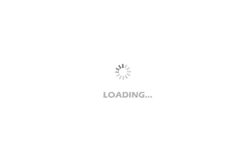
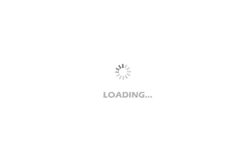
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications