------Storage and reading of 24C02-----------
1. Use 24C02 to save the content of an array, and then read it out;
2. Be proficient in the operation of the IIC bus;
3. Start signal, stop signal, response and non-response signals, busy signal,
read a byte data subroutine from the current position, write a byte subroutine from the current position,
read a byte data subroutine from the specified position, and write a byte subroutine from the specified position.
4. Operation timing of various signals;
5. This program can also be used as a general subroutine of IIC;
6. This program has been tested on the Puzhong MCU training box;
*/
#include
#include
#define uint unsigned int
#define uchar unsigned char
unsigned char code dula[] = {0x3f,0x06,0x5b,0x4f,
0x66,0x6d,0x7d,0x07,0x7f,0x6f,0x40};
uchar wula[]={0xfe,0xfd,0xfb,0xf7,0xef,0xdf,0xbf,0x7f};
sbit sda=P2^1; //define serial data port
sbit scl=P2^0; //define serial clock port
void delay(uint z);
void start();
void stop();
void ack(bit aa);
uchar iic_readbyte();
void iic_writebyte(uchar date1);
void checkack();
uchar iic_addr_read(uchar addr);
void iic_addr_write(uchar addr,uchar date1);
void iic_addr_readn(uchar addr,uchar *s,uchar n);
void iic_addr_writen(uchar addr,uchar *s,uchar n);
void disp();
void nop5();
uchar write1[]={1,2,10,0,0,10,4,8,9,3};
uchar read1[]={0,0,0,0,0,0,0,0,0,0};
bit ack1;
uchar temp3;
//uchar flag1,flag2,num1,num2;
void main()
{
/* The first method to read and write multiple bytes from 24C02
uchar jj;
for(jj=0;jj<8;jj++)
{
iic_addr_write(jj+5,write1[jj]);
delay(10); //This delay is very important;
}
delay(10);
for(jj=0;jj<8;jj++)
{
read1[jj]=iic_addr_read(jj+5);
delay(10);//This delay is very important;
} */
//
//The second method reads and writes multiple bytes from 24C02
iic_addr_writen(8,write1,8);
delay(50);
iic_addr_readn(8,read1,8);
while(1)
{disp();
}
}
//-----5US delay subroutine--------------
void nop5()
{
_nop_();_nop_();_nop_();_nop_();_nop_();
}
//-------XMS delay subroutine----------------
void delay(uint z)
{
uint x,y;
for(x=0;x
}
//-------Start signal-----------
void start()
{
sda=1;
nop5();
scl=1;
nop5();
sda=0;
nop5();
scl=0;
nop5();
}
//------Stop signal-----------
void stop()
{
sda=0;
nop5();
scl=1;
nop5();
sda=1;
nop5();
scl=1;
nop5();
}
//=------Acknowledgement and non-acknowledgement signals---------
void ack(bit aa)
{
scl=0;
nop5();
if(aa==1)
sda=1;
else
sda=0;
scl=1;
nop5();
scl=0;
nop5();
}
//-------Busy signal------------
void checkack()
{ uchar i;
sda=1;
scl=0;
nop5();
scl=1;
nop5();
while((sda==1)&&(i<250))
{i++;}
scl=0;
nop5();
}
//-------Read a byte of data from the current position---------------
uchar iic_readbyte()
{
uchar i,temp;
sda=1;
scl=0;
nop5();
for(i=0;i<8;i++)
{
temp=temp<<1;
scl=1;
nop5();
temp=temp|sda;
scl=0;
// nop5();
// nop5();
}
scl=0;
nop5();
return(temp);
}
//-----Write a byte from the current position subroutine-------------
void iic_writebyte(uchar date1 )
{
uchar i,temp;
temp=date1;
for(i=0;i<8;i++)
{
temp=temp<<1;
sda=CY;
scl=1;
nop5();
scl=0;
//nop5();
// nop5();
}
checkack();
delay(1);
scl=0;
nop5();
}
//---Read a byte data subroutine from the specified position-----------------
uchar iic_addr_read(uchar addr)
{ uchar k2;
start();
iic_writebyte(0xa0);
delay(1);
iic_writebyte(addr);
delay(1);
start();
delay(10);
iic_writebyte(0xa1);
delay(10);
k2=iic_readbyte();
ack(1);
stop();
return(k2);
}
//---Write a byte from the specified position subroutine-------------
void iic_addr_write(uchar addr,uchar date1)
{
start();
iic_writebyte(0xa0);
delay(1);
iic_writebyte(addr);
delay(1);
iic_writebyte(date1);
delay(1);
stop();
}
//----------Write multiple bytes from the specified position subroutine-------------------
void iic_addr_writen(uchar addr,uchar *s,uchar n)
{
uchar i;
start();
iic_writebyte(0xa0);
//delay(1);
iic_writebyte(addr);
// delay(1);
for(i=0;i
iic_writebyte(*s);
delay(20);//This delay must be longer, otherwise it is easy to make mistakes
s++; //Because the device reacts slowly, give it enough time;
}
stop();
}
//-------Read multiple bytes from the specified position subroutine-------------------------
void iic_addr_readn(uchar addr,uchar *s,uchar n)
{
uchar i;
start();
iic_writebyte(0xa0);
//delay(1);
iic_writebyte(addr);
//delay(10);
start();
//delay(15);
iic_writebyte(0xa1);
//delay(10);
for(i=0;i
*s=iic_readbyte();
delay(1);
ack(0);
delay(20);//This delay must be longer, otherwise it is easy to make mistakes
++; //Because the device reacts slowly, give it enough time;
}
*s=iic_readbyte();
ack(1);
stop();
}
//-----显示数据----------
void disp()
{uchar i;
for(i=0;i<8;i++)
{P0=dula[read1[i]];
P1=wula[i];
delay(3);
P0=0;
}
}
Previous article:51 MCU I2C bus driver
Next article:Simulate traffic light control (MCU C program)
Recommended ReadingLatest update time:2024-11-16 22:54
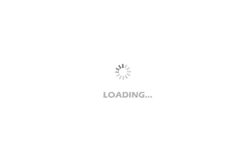
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Plant growth monitoring system source code based on Raspberry Pi 5
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TI Signal Chain and Power Q&A Series Live Broadcast - Gate Driver Special Live Broadcast with Prizes in Progress!
- Main application categories of millimeter wave radar
- pybL development board pinout
- Npn tube, Iceo is the reverse cutoff current, C and E are both N, where does the reverse come from, what does the reverse here mean...
- EEWORLD University ---- MSP CapTIvate Adaptive Sensor PCB Design Guide
- Children's Day is coming, what do you want to reminisce about?
- 【Running posture training shoes】No.009-Work submission
- 【Chuanglong TL570x-EVM】HDMI data cable adapter
- About MSP430 Miscellaneous Talks --Precise Delay of delay_cycles
- One article to understand the RF chip industry chain and its domestic status!