/*
Target:89S51
Program description: This example shows how to use Port 1 of 8051 to connect to LCD display. P3.3, P3.4 and P3.5 of Port 3 are connected to the control lines of LCD display. When the program is executed, the LCD display will show a digital clock. Port 2 is connected to the 4×4 keypad, which can modify the time. Button B of the 4×4 keypad can modify the time.
/************************************************* ***** */
#include
#include
#define TIMER0_COUNT 0xD8F0 /*10000h-((12,000,000/(12*100))*/
/* Digital clock working mode*/
#define SET 11
#define TRUE 1
#define FALSE 0
#define putchar write_LCD_da
typedef struct {
char hour;
char minute;
char second;
} time;
typedef struct {
char year;
char month;
char day;
} date;
time now={23,59,0},display;
date today={04,05,29},tmpday;
static unsigned timer0_tick=100,mode=0,operation;
char co
char co
"SUN"};
char co
char co
char gotkey();
void display_time(void)
{
gotoxy(1,0);
display_LCD_number(display.hour);
display_LCD_string(":");
display_LCD_number(display.minute);
display_LCD_string(":");
display_LCD_number(display.second);
}
void display_date()
{
char i,days=4;
gotoxy(2,2);
display_LCD_number(today.year);
display_LCD_string("/");
display_LCD_number(today.month);
display_LCD_string("/");
display_LCD_number(today.day);
display_LCD_string(" ");
if (today.month > 1)
for(i=0;i<=today.month-2;i++)
days+=(dayofmonth[i]%7);
if( today.year !=0 ) days+=((today.year-1)/4)+today.year+1;
if (today.year%4==0 && today.month >2) days++;
days=(days+today.day) % 7;
display_LCD_string(&weekday[days][0]);
}
int getdigit(unsigned char x,unsigned char y)
{
char keys;
do {
gotoxy(x,y);
putchar('_');
keys = gotkey();
gotoxy(x,y);
putchar(int2char[keys]);
} while(keys>9);
return(keys);
}
int gettime()
{
char temp;
do {
while((temp=getdigit(1,0))>2); //The tens digit cannot be greater than 2
temp=temp*10+getdigit(1,1);
if (temp > 23) display_time();
} while (temp > 23);
display.hour=temp;
while((temp=getdigit(1,3))>5);
display.minute=temp*10+getdigit(1,4);
return(TRUE);
}
char monthday(char year,char month)
{
if(month==2 && year%4==0) // February in a leap year has 29 days
return(29);
else
return (dayofmonth[month-1]); //The number of days in the month in a non-leap year
}
int getdate()
{
char temp,days;
temp=getdigit(2,2);
tmpday.year=temp*10+getdigit(2,3);
do {
while((temp=getdigit(2,5))>1); //The ten digit of the month cannot be greater than 1
temp=temp*10+getdigit(2,6);
if (temp > 12) display_date(); //The month number cannot be greater than 12
} while (temp > 12);
tmpday.month=temp;
do {
while((temp=getdigit(2,8))>3); //The ten digit of the day cannot be greater than 3
temp=temp*10+getdigit(2,9);
days=monthday(tmpday.year,tmpday.month);
if(temp > days || temp==0) display_date();
} while (temp > days || temp==0);
//If the entered date is greater than the date of the month, re-enter
tmpday.day=temp;
return(TRUE);
}
static void timer0_isr(void) interrupt TF0_VECTOR using 1
{
TR0=0;
TL0=(TIMER0_COUNT & 0x00FF);
TH0=(TIMER0_COUNT >> 8);
TR0=1;
if(--timer0_tick) return;
timer0_tick=100;
now.second++; //second plus 1
if (now.second==60) { //If seconds equals 60
now.second=0; //seconds are restored to 0
now.minute++; // add 1 to the minute
if (now.minute==60) { //If the minute is equal to 60
now.minute=0; //Minutes are restored to 0
now.hour++; // add 1 to the hour
if (now.hour==24) { //If the hour is equal to 24
now.hour=0; //The hour is restored to 0
today.day++; // add 1 to the day
if (today.day>monthday(today.year,
today.month)) {
today.day=1;
//If the day exceeds the maximum number of days in the month, it becomes 1
today.month++;
//month plus 1
if (today.month == 13) {
//If month is equal to 13
today.month=1;
//Month returns to 1
today.year++;
//Year plus 1
}
}
display_date();
}
}
}
if (operation==SET ) return;
display=now;
display_time();
}
static void timer0_initialize(void)
{
EA=0;
TR0=0;
TMOD &= 0XF0;
TMOD |= 0x01;
TL0=(TIMER0_COUNT & 0x00FF);
TH0=(TIMER0_COUNT >> 8);
PT0=0;
ET0=1;
TR0=1;
EA=1;
}
void main (void) {
char keys;
init_LCD();
clear_LCD();
gotoxy(2,0);
display_LCD_string("20");
display=now;
display_time();
display_date();
gotoxy(1,9);
display_LCD_string(&command[mode][0]);
timer0_initialize();
do {
keys = gotkey();
if(keys==SET) {
operation=SET;
if ( gettime()) now=display;
if ( getdate()) {
today=tmpday;
display_date();
}
}
operation=0;
} while(1);
}
Previous article:89S51 plays the song Happy Birthday c program
Next article:PWM square wave generation 51 program
Recommended ReadingLatest update time:2024-11-16 18:04
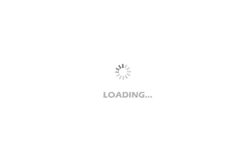
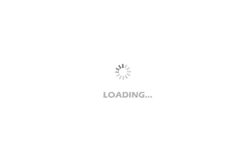
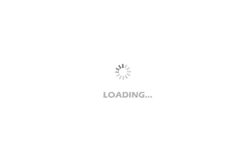
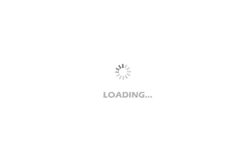
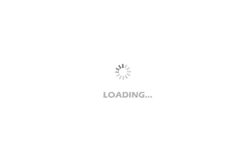
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【Silicon Labs Development Kit Review 03】+IO/Uart Usage
- Even if we give the complete set of drawings, Chinese people still cannot build high-end lithography machines?
- System Verilog 1800-2012 Syntax Manual
- aos multithreading and mutex lock
- Now many circuits do not have watchdogs, but some watchdog circuits are necessary. I would like to ask in which applications,...
- Brief discussion: Electromagnetic compatibility (EMC) radio frequency electromagnetic field radiation immunity test plan
- How to improve the frequency accuracy of the resonant circuit?
- [FS-IR02 + D1CS-D54] - 3: Electrical performance index detection (D1CS-D54)
- RF application scenarios——Q value
- Last three days! Apply for the free Zhongke Yihai Micro-Shenzhen series FPGA development board EQ6HL45