1. Experimental task:
Use the timer/counter T0 of the AT89S51 microcontroller to generate a one-second timer as the second counting time. When one second is generated, the second count increases by 1. When the second count reaches 60, it automatically starts from 0. The hardware circuit is shown in the figure below.
2. Circuit schematic diagram
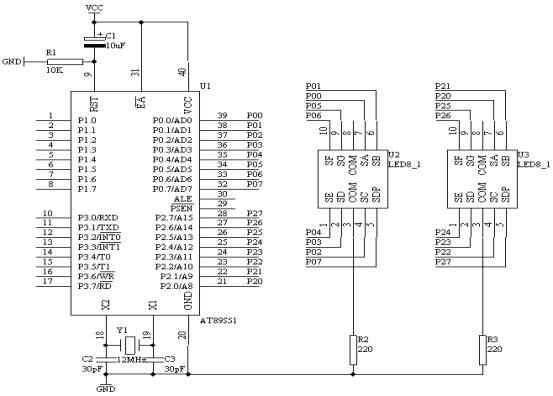
Figure 4.15.1
3. Hardware connection on the system board
(1. Connect the P0.0/AD0-P0.7/AD7 ports in the "MCU System" area to any a-h ports in the "Four-way Static Digital Display Module" area with an 8-core cable; requirements: P0.0/AD0 corresponds to a, P0.1/AD1 corresponds to b, ..., P0.7/AD7 corresponds to h.
(2. Connect the P2.0/A8-P2.7/A15 ports in the "MCU System" area to any a-h ports in the "Four-way Static Digital Display Module" area with an 8-core cable; requirements: P2.0/A8 corresponds to a, P2.1/A9 corresponds to b, ..., P2.7/A15 corresponds to h.
4. Programming content
The internal 16-bit timer/counter of the AT89S51 microcontroller is a programmable timer/counter, which can work in 13-bit timing mode, 16-bit timing mode and 8-bit timing mode. It can be completed by setting the special function register TMOD. When the timer/counter works is also completed by setting the TCON special function register through software.
Now we choose the 16-bit timing working mode. For T0, the maximum timing is only 65536us, that is, 65.536ms, which cannot achieve the 1-second timing we need. Therefore, we must use software to deal with this problem. Suppose we take the maximum timing of T0 as 50ms, that is, to time 1 second, it takes 20 times of 50ms timing. For these 20 times, we can use software methods to count.
Therefore, we set TMOD = 00000001B, that is, TMOD = 01H.
Next, we need to load the preset initial values of TH0 and TL0 of T0 timer/counter. The following formula can be used to calculate
TH0 = (216-50000) / 256
TL0 = (216 - 50000) MOD 256
When T0 is working, how do we know that the 50ms timing time has expired? This time we detect the TF0 flag in the TCON special function register. If TF0 = 1, it means that the timing time has expired.
5. Program flowchart

Figure 4.15.2
6. Assembly source program (query method)
SECOND EQU 30H
TCOUNT EQU 31H
ORG 00H
START: MOV SECOND,#00H
MOV TCOUNT,#00H
MOV TMOD,#01H
MOV TH0,#(65536-50000) / 256
MOV TL0,#(65536- 50000) MOD 256
SETB TR0
DISP: MOV A,SECOND
MOV B,#10
DIV AB
MOV DPTR,#TABLE
MOVC A,@A+DPTR
MOV P0,A
MOV A,B
MOVC A,@A+DPTR
MOV P2,A
WAIT: JNB TF0,WAIT
CLR TF0
MOV TH0,#(65536-50000) / 256
MOV TL0,#(65536-50000) MOD 256
INC TCOUNT
MOV A,TCOUNT
CJNE A,#20,NEXT
MOV TCOUNT,#00H
INC SECOND
MOV A,SECOND
CJNE A,#60,NEX
MOV SECOND,#00H
NEX: LJMP DISP
NEXT: LJMP WAIT
TABLE: DB 3FH,06H,5BH,4FH,66H,6DH,7DH,07H,7FH,6FH
END
7. C language source program (query method)
#include
unsigned char code dispcode[]={0x3f,0x06,0x5b,0x4f,
0x66,0x6d,0x7d,0x07,
0x7f,0x6f,0x77,0x7c,
0x39,0x5e ,0x79,0x71,0x00};
unsigned char second;
unsigned char tcount;
void main(void)
{
TMOD=0x01;
TH0=(65536-50000)/256;
TL0=(65536-50000)%256;
TR0=1;
tcount=0;
second=0;
P0=dispcode[second/10];
P2=dispcode[second%10];
while(1)
{
if(TF0==1)
{
tcount++;
if(tcount==20)
{
tcount=0;
second++;
if(second==60)
{
second=0;
}
P0=dispcode[second/10];
P2=dispcode[second%10];
}
TF0=0;
TH0=(65536-50000)/256;
TL0=(65536-50000)%256;
}
}
}
1. Assembly source program (interrupt method)
SECOND EQU 30H
TCOUNT EQU 31H
ORG 00H
LJMP START
ORG 0BH
LJMP INT0X
START: MOV SECOND,#00H
MOV A,SECOND
MOV B,#10
DIV AB
MOV DPTR,#TABLE
MOVC A,@A+ DPTR
MOV P0,A
MOV A,B
MOVC A,@A+DPTR
MOV P2,A
MOV TCOUNT,#00H
MOV TMOD,#01H
MOV TH0,#(65536-50000) / 256
MOV TL0,#(65536-50000) MOD 256
SETB TR0
SETB ET0
SETB EA
SJMP $
INT0X:
MOV TH0,#(65536-50000) / 256
MOV TL0,#(65536-50000) MOD 256
INC TCOUNT
MOV A,TCOUNT
CJNE A,#20,NEXT
MOV TCOUNT,#00H
INC SECOND
MOV A,SECOND
CJNE A,#60,NEX
MOV SECOND,#00H
NEX: MOV A,SECOND
MOV B,#10
DIV AB
MOV DPTR,#TABLE
MOVC A,@A+DPTR
MOV P0,A
MOV A,B
MOVC A,@A+DPTR
MOV P2,A
NEXT: RETI
TABLE: DB 3FH,06H,5BH,4FH,66H,6DH,7DH,07H,7FH,6FH
END
2. C language source program (interrupt method)
#include
unsigned char code dispcode[]={0x3f,0x06,0x5b,0x4f,
0x66,0x6d,0x7d,0x07,
0x7f,0x6f,0x77,0x7c,
0x39,0x5e ,0x79,0x71,0x00};
unsigned char second;
unsigned char tcount;
void main(void)
{
TMOD=0x01;
TH0=(65536-50000)/256;
TL0=(65536-50000)%256;
TR0=1;
ET0=1;
EA=1;
tcount=0;
second=0;
P0=dispcode[second/10];
P2=dispcode[second%10];
while(1);
}
void t0(void) interrupt 1 using 0
{
tcount++;
if(tcount==20)
{
tcount=0;
second++;
if(second==60)
{
second =0;
}
P0=dispcode[second/10];
P2=dispcode[second%10];
}
TH0=(65536-50000)/256;
TL0=(65536-50000)%256;
}
Reference address:15. Timing counter T0 as timing application technology (I)
Use the timer/counter T0 of the AT89S51 microcontroller to generate a one-second timer as the second counting time. When one second is generated, the second count increases by 1. When the second count reaches 60, it automatically starts from 0. The hardware circuit is shown in the figure below.
2. Circuit schematic diagram
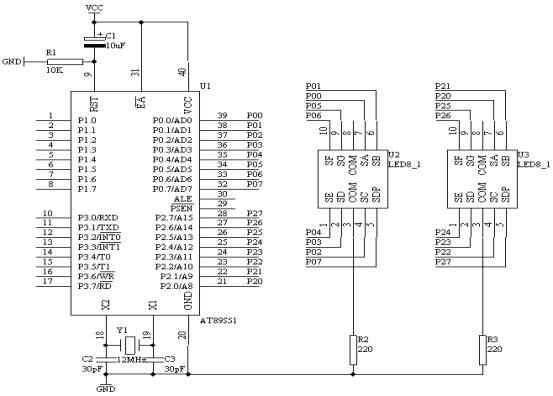
(1. Connect the P0.0/AD0-P0.7/AD7 ports in the "MCU System" area to any a-h ports in the "Four-way Static Digital Display Module" area with an 8-core cable; requirements: P0.0/AD0 corresponds to a, P0.1/AD1 corresponds to b, ..., P0.7/AD7 corresponds to h.
(2. Connect the P2.0/A8-P2.7/A15 ports in the "MCU System" area to any a-h ports in the "Four-way Static Digital Display Module" area with an 8-core cable; requirements: P2.0/A8 corresponds to a, P2.1/A9 corresponds to b, ..., P2.7/A15 corresponds to h.
4. Programming content
The internal 16-bit timer/counter of the AT89S51 microcontroller is a programmable timer/counter, which can work in 13-bit timing mode, 16-bit timing mode and 8-bit timing mode. It can be completed by setting the special function register TMOD. When the timer/counter works is also completed by setting the TCON special function register through software.
Now we choose the 16-bit timing working mode. For T0, the maximum timing is only 65536us, that is, 65.536ms, which cannot achieve the 1-second timing we need. Therefore, we must use software to deal with this problem. Suppose we take the maximum timing of T0 as 50ms, that is, to time 1 second, it takes 20 times of 50ms timing. For these 20 times, we can use software methods to count.
Therefore, we set TMOD = 00000001B, that is, TMOD = 01H.
Next, we need to load the preset initial values of TH0 and TL0 of T0 timer/counter. The following formula can be used to calculate
TH0 = (216-50000) / 256
TL0 = (216 - 50000) MOD 256
When T0 is working, how do we know that the 50ms timing time has expired? This time we detect the TF0 flag in the TCON special function register. If TF0 = 1, it means that the timing time has expired.
5. Program flowchart

SECOND EQU 30H
TCOUNT EQU 31H
ORG 00H
START: MOV SECOND,#00H
MOV TCOUNT,#00H
MOV TMOD,#01H
MOV TH0,#(65536-50000) / 256
MOV TL0,#(65536- 50000) MOD 256
SETB TR0
DISP: MOV A,SECOND
MOV B,#10
DIV AB
MOV DPTR,#TABLE
MOVC A,@A+DPTR
MOV P0,A
MOV A,B
MOVC A,@A+DPTR
MOV P2,A
WAIT: JNB TF0,WAIT
CLR TF0
MOV TH0,#(65536-50000) / 256
MOV TL0,#(65536-50000) MOD 256
INC TCOUNT
MOV A,TCOUNT
CJNE A,#20,NEXT
MOV TCOUNT,#00H
INC SECOND
MOV A,SECOND
CJNE A,#60,NEX
MOV SECOND,#00H
NEX: LJMP DISP
NEXT: LJMP WAIT
TABLE: DB 3FH,06H,5BH,4FH,66H,6DH,7DH,07H,7FH,6FH
END
7. C language source program (query method)
#include
unsigned char code dispcode[]={0x3f,0x06,0x5b,0x4f,
0x66,0x6d,0x7d,0x07,
0x7f,0x6f,0x77,0x7c,
0x39,0x5e ,0x79,0x71,0x00};
unsigned char second;
unsigned char tcount;
void main(void)
{
TMOD=0x01;
TH0=(65536-50000)/256;
TL0=(65536-50000)%256;
TR0=1;
tcount=0;
second=0;
P0=dispcode[second/10];
P2=dispcode[second%10];
while(1)
{
if(TF0==1)
{
tcount++;
if(tcount==20)
{
tcount=0;
second++;
if(second==60)
{
second=0;
}
P0=dispcode[second/10];
P2=dispcode[second%10];
}
TF0=0;
TH0=(65536-50000)/256;
TL0=(65536-50000)%256;
}
}
}
1. Assembly source program (interrupt method)
SECOND EQU 30H
TCOUNT EQU 31H
ORG 00H
LJMP START
ORG 0BH
LJMP INT0X
START: MOV SECOND,#00H
MOV A,SECOND
MOV B,#10
DIV AB
MOV DPTR,#TABLE
MOVC A,@A+ DPTR
MOV P0,A
MOV A,B
MOVC A,@A+DPTR
MOV P2,A
MOV TCOUNT,#00H
MOV TMOD,#01H
MOV TH0,#(65536-50000) / 256
MOV TL0,#(65536-50000) MOD 256
SETB TR0
SETB ET0
SETB EA
SJMP $
INT0X:
MOV TH0,#(65536-50000) / 256
MOV TL0,#(65536-50000) MOD 256
INC TCOUNT
MOV A,TCOUNT
CJNE A,#20,NEXT
MOV TCOUNT,#00H
INC SECOND
MOV A,SECOND
CJNE A,#60,NEX
MOV SECOND,#00H
NEX: MOV A,SECOND
MOV B,#10
DIV AB
MOV DPTR,#TABLE
MOVC A,@A+DPTR
MOV P0,A
MOV A,B
MOVC A,@A+DPTR
MOV P2,A
NEXT: RETI
TABLE: DB 3FH,06H,5BH,4FH,66H,6DH,7DH,07H,7FH,6FH
END
2. C language source program (interrupt method)
#include
unsigned char code dispcode[]={0x3f,0x06,0x5b,0x4f,
0x66,0x6d,0x7d,0x07,
0x7f,0x6f,0x77,0x7c,
0x39,0x5e ,0x79,0x71,0x00};
unsigned char second;
unsigned char tcount;
void main(void)
{
TMOD=0x01;
TH0=(65536-50000)/256;
TL0=(65536-50000)%256;
TR0=1;
ET0=1;
EA=1;
tcount=0;
second=0;
P0=dispcode[second/10];
P2=dispcode[second%10];
while(1);
}
void t0(void) interrupt 1 using 0
{
tcount++;
if(tcount==20)
{
tcount=0;
second++;
if(second==60)
{
second =0;
}
P0=dispcode[second/10];
P2=dispcode[second%10];
}
TH0=(65536-50000)/256;
TL0=(65536-50000)%256;
}
Previous article:16. Timing counter T0 as timing application technology (II)
Next article:14. 4×4 matrix keyboard recognition technology
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
MoreDaily News
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
Guess you like
- TMS320C6711 serial communication initialization program
- Data searchRSL10-COIN-GEVB
- MSP430 MCU Development Record (5)
- EEWORLD University Hall----Using JTAG with UCD3138
- Share: What is the use of the 100 Ω resistor before the MOSFET gate?
- POL thermal resistance measurement and SOA evaluation
- Registration for the live broadcast with prizes is in progress: Infineon's intelligent motor drive solution
- [TI Live Review] TI Robot System Learning Kit Lecture (including video, ppt, QA)
- [AB32VG1 development board review] MINI environmental status detector (and final report)
- mpy allows passing bytes/bytearray to json.loads