Yes, why is it only mentioned once in the manual? Later, when I searched online, I found that
I was not the only one complaining. There was only one sentence on the entire DATASHEET that mentioned the specific usage of systick. I was confused for a long time and asked the boss, but he looked down on me. I was very upset and looked online, but there was still no information. Later, I finally saw an article about systick integrated by Xinda Studio. I thought it was good and shared it with you. I hope it will be helpful to you. Due to time constraints, there may be some unclear details and logic in the article. Please forgive me.
1. Introduction to systick
Systick is just a timer, but it is placed in NVIC. Its main purpose is to
provide a hardware interrupt (called tick interrupt) to the operating system. Students who have not studied operating systems
may be very depressed. What is a tick interrupt? Here is a simple explanation. When the operating system is running
, there will also be a "heartbeat". It works according to the beat of the "heartbeat", dividing the entire time period into many
small time slices. Each task can only run for the length of a "time slice" at a time and must exit
to allow other tasks to run. This ensures that no task will occupy the entire system. This heartbeat
can be triggered periodically by a timer, and this timer is systick. Obviously, this "heartbeat"
is not allowed to be accessed and modified by anyone at will. As long as its
enable bit in the SysTick control and status register is not cleared, it will never stop. It has four registers, which I list here:
STK_CSR, 0xE000E010 -- Control register
STK_LOAD, 0xE000E014 -- Reload register
STK_VAL, 0xE000E018 -- Current value register
STK_CALRB, 0xE000E01C -- Calibration value register
The following part refers to an article on the Internet, the URL is:
https://home.eeworld.com.cn/my/space.php?uid=116357&do=blog&id=31714
Thanks to the author "416561760's Blog" for providing such a detailed register description article.
1. STK_CSR control register: There are 4 bits in the register that have meaning
Bit 0: ENABLE, Systick enable bit (0: turn off Systick function; 1: turn on Systick
function)
Bit 1: TICKINT, Systick interrupt enable bit (0: turn off Systick interrupt; 1: turn on
Systick interrupt)
Bit 2: CLKSOURCE, Systick clock source selection (0: use HCLK/8 as Systick
clock; 1: use HCLK as Systick clock)
Bit 3: COUNTFLAG, Systick count comparison flag, if SysTick has counted to 0 after the last read of this register,
this bit is 1. If this bit is read, it will be automatically cleared.
2. STK_LOAD reload register:
Systick is a decrementing timer. When the timer decrements to 0, the value in the reload register will
be reloaded and continue to decrement. The STK_LOAD reload register is a 24-bit register with a maximum
count of 0xFFFFFF.
3. STK_VAL current value register:
It is also a 24-bit register. When read, it returns the current countdown value. Writing it clears it to zero and also
clears the COUNTFLAG flag in the SysTick control and status register.
4. STK_CALRB calibration value register:
Bit 31 NOREF: 1 = No external reference clock (STCLK is not available) 0 = External reference clock is available
Bit 30 SKEW: 1 = Calibration value is not accurate 1ms 0 = Calibration value is accurate 1ms
Bit [23:0]: Calibration value
Indicates the calibration value when the SysTick counter runs on HCLK
max/8 as external clock. The value is product dependent, please refer to
the Product Reference Manual, SysTick Calibration Value section. When
HCLK is programmed at the maximum frequency, the SysTick period is 1ms.
If calibration information is not known, calculate the calibration value
required from the frequency of the processor clock or external clock.
2. Systick programming Now we want to use the Systick timer to make an accurate delay function, such as making the LED
flash once
with an accurate delay of 1 second.
Idea: Use the systick timer as a down counter. After setting the initial value and enabling it, it will
decrement the counter by 1 every system clock cycle. When the count reaches 0, the SysTick counter automatically reloads the initial value and continues to
count, triggering an interrupt at the same time.
Then, each time the counter is decremented to 0, the time elapsed is: system clock cycle * counter initial value. We
use 72M as the system clock, so the time taken for each counter to decrement by 1 is 1/72M.
If the initial value of the counter is 72000, then each time the counter is decremented to 0, the time elapsed is (1/72M) * 72000 =
0.001, that is, 1ms. However, when we usually use it, we do not directly use 72M as the systick clock, but indirectly divide the AHB frequency (8 divisions), and the systick clock is 9M at this time. 1/9000000*9000=1ms, this is the specific method of configuring the systick timer mentioned in the STM32 specification.
Now the Delay(1) we have made is a 1 millisecond delay. Delay(1000) is 1 second.
With the above ideas, systick programming is very simple.
Then start to configure systick. In fact, the strict process of configuring systick is as follows:
How to use systick using ST's function library:
1. Call SysTick_CounterCmd() - Enable SysTick counter
2. Call SysTick_ITConfig () -- Enable SysTick interrupt
3. Call SysTick_CLKSourceConfig() -- Set SysTick clock source.
4. Call SysTick_SetReload() -- Set SysTick reload value.
5. Call SysTick_ITConfig () -- Enable SysTick interrupt
6. Call SysTick_CounterCmd() -- Open SysTick counter
Here everyone must pay attention to the fact that the current register value VAL must be equal to 0!
SysTick->VAL = (0x00);
Only when the VAL value is 0, the counter automatically reloads RELOAD.
Next, you can directly call the Delay() function to delay. In the implementation of the delay function, it should be noted
that the global variable TimingDelay must use volatile, otherwise it may be optimized by the compiler.
Q: What is the SysTick timer?
SysTick is a 24-bit countdown timer. When it counts to 0, it will
automatically reload the timing initial value from the RELOAD register. As long as its enable bit in the SysTick control and status register is not cleared
, it will never stop.
Q: Why set the SysTick timer?
(1) Generate the clock beat of the operating system.
The SysTick timer is bundled in the NVIC and is used to generate the SYSTICK exception (exception number: 15).
In the past, most operating systems required a hardware timer to generate the tick interrupt required by the operating system as
the time base of the entire system. Therefore, a timer is needed to generate periodic interrupts, and it is best
not to allow user programs to access its registers at will to maintain the rhythm of the operating system's "heartbeat".
(2) It is easy to port programs between different processors.
The Cortex-M3 processor contains a simple timer. Because all CM3 chips have
this timer, the porting of software between different CM3 devices is simplified. The clock
source of this timer can be an internal clock (FCLK, the free-running clock on the CM3) or an external clock (
STCLK signal on the CM3 processor).
However, the specific source of STCLK is determined by the chip designer, so the clock frequency between different products
may be very different. You need to check the chip device manual to decide what to choose as the clock source.
The Sys Tick timer can generate interrupts. CM3 has a special exception type for it and has a place in the vector table
. It makes it much easier to port the operating system and other system software between CM3 devices
because it is handled the same in all CM3 products.
(3) As an alarm to measure time.
In addition to serving the operating system, the SysTick timer can also be used for other purposes: as an alarm
, for measuring time, etc. It should be noted that when the processor is halted during debugging,
the SysTick timer will also be suspended.
Q: How does Systick work?
First, set the counter clock source, CTRL->CLKSOURCE (control register). Set the reload value (R
ELOAD register) and clear the count register VAL (CURRENT in the figure below). Set CTRL-
>ENABLE to start timing.
If it is an interrupt, allow Systick interrupts and handle them in the interrupt routine. If the query mode is used, read
the COUNTFLAG flag of the control register continuously to determine whether the timing is zero. Or take one of the following
methods
. When the SysTick timer counts from 1 to 0, it will set the COUNTFLAG bit; and the following method
can clear it:
1. Read the SysTick Control and Status Register (STCSR)
2. Write any data to the SysTick Current Value Register (STCVR)
Only when the VAL value is 0, the counter automatically reloads RELOAD.
The biggest mission of SysTick is to generate exception requests regularly as the system time base. The OS needs
this "tick" to promote task and time management. To enable the SysTick exception,
set STCSR.TICKINT. In addition, if the vector table is relocated to SRAM, it is also necessary to
create a vector for the SysTick exception and provide the entry address of its service routine.
Interrupt mode reference:
void RCC_Configuration(void)
{
}
Configure specific systick()
void InitSysTick(void)
{
}
The entry point of the interrupt is: SysTickHandler()
void SysTickHandler(void)
{
}
Previous article:Research and design of intelligent sensor acquisition and transmission control system
Next article:Understanding of the pull-up register in ARM
Recommended ReadingLatest update time:2024-11-16 17:56
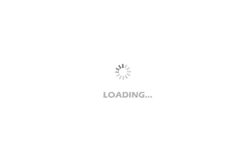
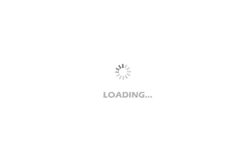
- Popular Resources
- Popular amplifiers
-
Automotive Electronics S32K Series Microcontrollers: Based on ARM Cortex-M4F Core
-
The STM32 MCU drives the BMP280 absolute pressure sensor program and has been debugged
-
DigiKey \"Smart Manufacturing, Non-stop Happiness\" Creative Competition - Small Weather Station - STM32F103 Sensor Driver Code
-
uCOS-III Kernel Implementation and Application Development Practical Guide - Based on STM32 (Wildfire)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications