void TIM1_Configuration (uint Period,uint Pulse)
{
}
void TIM2_Configuration (uint Period)
{
}
void NVIC_Configuration(void)
{
}
Previous article:Summary of 22 common concepts of ARM
Next article:TIM Configuration
Recommended ReadingLatest update time:2024-11-16 22:02
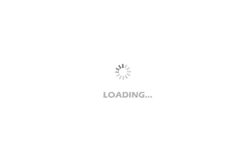
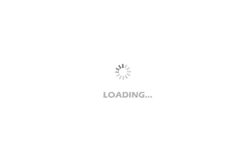
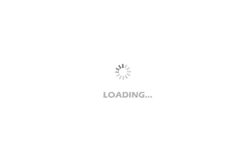
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- IC WKN Driver Laser Diode
- Buying analog discovery 2
- Ameya360: Will Intel's latest generation of discrete graphics cards be discontinued?
- Recruiting simulation engineers (software) Annual salary: 200,000-500,000 | Experience: more than 5 years | Work location: Shenzhen
- [Live broadcast on May 9] Discussing solutions to reduce downtime in the new manufacturing era, ADI live broadcast expert Zhao Yanhui is here again
- [Zhongke Bluexun AB32VG1 RISC-V board "run into" RTT evaluation] Software timer + hardware timer
- Why can't the LM2678S-ADJ original be adjusted to 24V after connecting the resistor? I modified the circuit myself (newbie help)
- [Domestic RISC-V Linux board Fang·Starlight VisionFive trial report] UART communication function usage
- Distinguish the difference between FPGA and CPLD based on structural characteristics and working principles
- Chat with Vicor engineers: How to increase payload capacity and flight time for commercial drones?