The schematic diagram of the timer circuit based on the microcontroller is shown below:

The first thing we learned when we studied microcontrollers was how to make LEDs flash, which was done using a delay program. Looking back now, this was not very appropriate. Why? Our main program made the light flash, so we couldn't do anything else. Does the microcontroller only work this way? Of course not, we can use a timer to make the light flash.
Example 1: Query method
ORG 0000H
AJMP START
ORG 30H
START:
MOV P1,#0FFH ; Turn off the light
MOV TMOD,#00000001B ;Timer/Counter 0 works in mode 1
MOV TH0,#15H
MOV TL0,#0A0H; the number is 5536
SETB TR0 ;Timer/Counter 0 starts running
LOOP:JBC TF0,NEXT; If TF0 is equal to 1, clear TF0 and go to NEXT
AJMP LOOP; otherwise jump to LOOP and execute
NEXT:CPL P1.0
MOV TH0,#15H
MOV TL0,#9FH; reset the initial value of the timer/counter
AJMP LOOP
END AJMP LOOP
END
Type the program, what do you see? The light is flashing, this is done by the timer, it is no longer the main program loop. Let's analyze the program briefly, why use JBC? TF0 is the overflow flag of timer/counter 0. When the timer overflows, this bit changes from 0 to 1, so by checking this bit, you can know whether the time has expired. After this bit is 1, the flag bit must be cleared to 0 by software, so that the bit will change from 0 to 1 when the next timer expires. Therefore, the JBC instruction is used. This instruction clears the bit to 0 while judging 1 to transfer.
The above program can make the light flash, but the main program can't do anything else except flashing the light! No, that's not right. We can insert some instructions between LOOP:... and AJMP LOOP to do other things, as long as the execution time of these instructions is less than the timing time. Then when we use the software delay program, can't we also use some instructions to replace DJNZ? Yes, but that requires you to accurately calculate the time of the instructions used, and then subtract the corresponding number of DJNZ cycles, which is very inconvenient. Now we only require that the time of the instructions used is less than the timing time, which is obviously too low. Of course, this method is still not good, so we often use the following method to achieve it. [page]
Program 2: Implemented using interrupts
ORG 0000H
AJMP START
ORG 000BH ;Interrupt vector address of timer 0
AJMP TIME0; Jump to the real timer program
ORG 30H
START:
MOV P1,#0FFH ; Turn off the light
MOV TMOD,#00000001B ;Timer/Counter 0 works in mode 1
MOV TH0,#15H
MOV TL0,#0A0H; the number is 5536
SETB EA ; Enable general interrupt
SETB ET0 ; Enable timer/counter 0
SETB TR0 ;Timer/Counter 0 starts running
LOOP: AJMP LOOP; When actually working, you can write any program here
TIME0: ; Timer 0 interrupt handler
PUSH ACC
PUSH PSW; Push PSW and ACC into stack protection
CPL P1.0
MOV TH0,#15H
MOV TL0,#0A0H ; reset timing constant
POP PSW
POP ACC
RETI
END
In the above routine, when the timer time is reached, TF0 changes from 0 to 1, which will trigger an interrupt. The CPU will automatically go to 000B to find the program and execute it. Since there are only 8 bytes of space left for the timer interrupt, it is obviously not enough to write all the interrupt handlers. Therefore, a jump instruction is arranged at 000B to jump to the program that actually handles the interrupt. In this way, the interrupt program can be written anywhere and can be of any length. After entering the timer interrupt, the current status must be saved first. The program only demonstrates the saving of ACC and PSW. In actual work, the values of the units that may change should be pushed into the stack for protection as needed (in this program, no value actually needs to be saved, it is just a demonstration here).
After running the two MCU programs above, we found that the flashing of the light was very fast and could not be distinguished at all. We just felt that the light was shaking visually. Why? We can calculate that the preset number in the timer is 5536, so every 60,000 pulses is the timing time. How long is the time of these 60,000 pulses? Our crystal oscillator is 12M, so it is 60,000 microseconds, that is, 60 milliseconds, so the speed is very fast. If I want to achieve a 1S timing, what should I do? Under the crystal oscillator frequency, the longest timing is 65.536 milliseconds! A routine is given above.
ORG 0000H
AJMP START
ORG 000BH ;Interrupt vector address of timer 0
AJMP TIME0; Jump to the real timer program
ORG 30H
START:
MOV P1,#0FFH ; Turn off the light
MOV 30H,#00H ; software counter pre-clear 0
MOV TMOD,#00000001B ;Timer/Counter 0 works in mode 1
MOV TH0,#3CH
MOV TL0,#0B0H; the number is 15536
SETB EA ; Enable general interrupt
SETB ET0 ; Enable timer/counter 0
SETB TR0 ;Timer/Counter 0 starts running
LOOP: AJMP LOOP; When actually working, you can write any program here
TIME0: ; Timer 0 interrupt handler
PUSH ACC
PUSH PSW; Push PSW and ACC into stack protection
INC 30H
MOV A,30H[page]
CJNE A,#20,T_RET ;Has the value in cell 30H reached 20?
T_L1: CPL P1.0; arrived, invert P10
MOV 30H,#0 ; Clear software counter
T_RET:
MOV TH0,#15H
MOV TL0,#9FH ; Reset timing constant
POP PSW
POP ACC
RETI
END
Let's analyze it by ourselves first to see how it is implemented? The concept of software counter is used here. The idea is to use timer/counter 0 to make a 50 millisecond timer. When the timer is up, P10 is not negated immediately, but the value in the software counter is added by 1. If the software counter reaches 20, P10 is negated and the value in the software counter is cleared. Otherwise, it returns directly. In this way, P10 is negated once every 20 timer interrupts, so the timing time is extended to 20*50, that is, 1000 milliseconds.
This idea is very useful in engineering. Sometimes we need several timers, but there are only 2 in 51. What should we do? In fact, as long as the time of these timers has a certain common divisor, we can use software timer to implement it. For example, I want to realize that the light connected to P10 port presses 1S each time, and the light connected to P11 port flashes every 2S. How to achieve it? Yes, we use two counters. When it counts to 20, it negates P10 and clears it to zero, as shown above. When the other counts to 40, it negates P11 and then clears it to 0. Isn't that enough? The program for this part is as follows
ORG 0000H
AJMP START
ORG 000BH ;Interrupt vector address of timer 0
AJMP TIME0; Jump to the real timer program
ORG 30H
START:
MOV P1,#0FFH ; Turn off the light
MOV 30H,#00H ; software counter pre-clear 0
MOV TMOD,#00000001B ;Timer/Counter 0 works in mode 1
MOV TH0,#3CH
MOV TL0,#0B0H; the number is 15536
SETB EA ; Enable general interrupt
SETB ET0 ; Enable timer/counter 0
SETB TR0 ;Timer/Counter 0 starts running
LOOP: AJMP LOOP; When actually working, you can write any program here
TIME0: ; Timer 0 interrupt handler
PUSH ACC
PUSH PSW; Push PSW and ACC into stack protection
INC 30H
INC 31H ;both counters increase by 1
MOV A,30H
CJNE A,#20,T_NEXT ;Has the value in cell 30H reached 20?
T_L1: CPL P1.0; arrived, invert P10
MOV 30H,#0 ; Clear software counter
T_NEXT:
MOV A,31H
CJNE A,#40,T_RET ;Has the value in cell 31h reached 40?
T_L2:
CPL P1.1
MOV 31H,#0; Arrived, invert P11, clear the counter, return
T_RET:
MOV TH0,#15H
MOV TL0,#9FH ; Reset timing constant
POP PSW
POP ACC
RETI
END
Previous article:AT89S51 watchdog program example
Next article:Causes of 51 MCU serial communication errors
Recommended ReadingLatest update time:2024-11-16 21:54
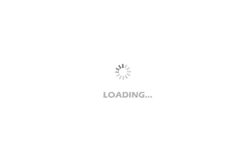
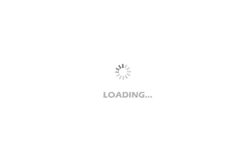
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Espressif ESP32-C3-DevKitM-1 review is coming soon. Let’s learn about this board first.
- After deleting a wire that was previously laid, AD09 got stuck.
- Canaan wants to record a video about K510. What kind of content do you hope to see? (Attached is the official information about K510)
- Share some difficult problems with the LM25116EN chip
- [RVB2601 Creative Application Development] Dynamically loading MBRE Postscript
- Distance of ESD tip discharge needle
- Bone Vibration Sensor LIS25BA-Anti-Wind Noise Example
- SensorTile.box Trial (3) Expert Mode Trial
- Introduction to CEDV Electricity Calculation Method
- [Anxinke UWB indoor positioning module NodeMCU-BU01] 04: Test results and problems of connecting to OLED screen