Introduction
The application of single-chip microcomputers is becoming more and more extensive, and there are more and more types of them. Since the embedded C language has strong readability and good portability, it greatly reduces the labor intensity of software engineers compared with assembly language, so more and more single-chip microcomputer engineers have begun to use C language programming. However, the portability of C language is limited to subroutines that are not related to hardware, while subroutines related to specific hardware cannot be ported. In single-chip microcomputer applications, bit operations (especially bit operations on pins) are very common, such as reading and writing EEPROM data and IC card data, field-type LCD display, etc. Many integrated circuits with serial destinations require single-chip microcomputers to use software to do I/O port reading and writing programs. How to make these subroutines have good versatility and high efficiency in code generation is a problem that many software engineers are considering. Here are two porting methods for C language bit operations.
1 Use logical operations to implement bit operations
Please see the following subroutine:
This is a subroutine to read a byte from the 88SC102 card through the microcontroller pin. The program uses the writing style in μC/OS-II, that is, variables and functions are written in "camel case", and constants and inline functions defined by define are written in all uppercase and underlined.
This program drives a pin to output CARD_CLK high and low signals, and reads CARD_SDA data from another pin one bit at a time.
1.1 For MSP430 series microcontrollers
This program is applied to MSP430 microcontrollers (this article uses MSP430F413 microcontrollers), and the following definitions should be included in the header file:
The compilation results are as follows:
This is almost the same result as programming by hand in assembly. The code is very efficient. [page]
1.2 For 51 series MCU
To apply this program in 51 series MCU, the following definition should be added to the header file:
From the assembly results, we can see that the direct clearing and setting of bits has reached the simplest level, but the reading of bit values is not ideal.
1.3 Used in 196/296 series microcontrollers
In microcontrollers such as 80C196MC and 80C296SA, the on-chip I/O ports can be window-mapped to low-end addresses. In this way, the I/O ports can be directly addressed, so the program code is the shortest and the execution speed is the fastest, but this makes the C program unportable. If window technology is not used, the on-chip I/O ports are memory-mapped and operate in the same way as ordinary memory addresses. Add the following definition to the header file to use the original program:
The assembled code is 56 bytes, and the code efficiency is also very high.
Using logical operations to implement bit operations, C programs are simple and clear, easy to transplant, and more readable. However, the 96 series microcontrollers cannot use JBC and JBS bit operation instructions, and the 51 series microcontrollers cannot use their unique bit operation instructions such as JB and JNB to improve code efficiency. Using the bit segment structure to implement bit operations can make up for this deficiency. [page]
2 Use the bit segment structure to implement bit operations
Rewrite the original program as follows:
2.1 Application in 51 series microcontrollers
To use ACC in C51, it is not necessary to define it in each subroutine, so add #define C51_ASM at the beginning of the file. In this way, the fourth, fifth, and sixth sentences will be ignored. Add the following definition in the header file:
The rest of the definitions are as described in the first part of this article. As a result, the assembly of sentence ⑧ becomes "MOV C, CardSDA" and "MOV ACC_0, C". In sentence ⑩, the function returns the parameter through R7, and the program has been simplified.
You can also define a bit segment structure like 196/296, using the JB instruction. Interested readers can try it themselves.
2.2 Application in 196/296 series microcontrollers
To apply this program in 196/296, you need to add a definition of a local variable ACCImg, which is the fourth, fifth, and sixth sentences in the previous program. Then add the following bit segment structure definition in the header file:
The port address variable must be defined as the following data type: bdata PIN; [page]
At the same time, add the macro definition in the header file:
In this way, ACCImg is defined as a low-end register, and ACC is its byte access form. The eighth sentence in the source program reads the pin, and the result of the assembly uses the JBC instruction. The whole program reduces the bytes compared to not using the bit segment, achieving the purpose of optimizing the code.
]
2.3 Application in MSP430 series microcontrollers
MSP430 series microcontrollers do not have bit operation instructions, so there is no need to define a bit segment structure. Just define ACC as an unsigned 8-bit number. The header file is defined as follows:
The result of the assembly is exactly the same as that of the bit operation using logical operations.
Conclusion
There are three types of bit operations on pins: directly setting or clearing, inputting data from the port, and outputting data from the port. The first two have been introduced above. The C program to output data from the port is as follows:
Among them: the first sentence OUT_SIO_DA(), the 5l series can be defined as a bit operation SIO_SDA=ACC_7; the 196/296 and 430 series can be defined as an if statement as above.
The name ACC is used as a local variable in the bit segment operation program. In C51, this happens to be the main accumulator, which is very practical for programs of half-duplex devices such as 240l and IC cards, but it is not so convenient when the SPI bus input/output is operated simultaneously.
There is no transplantation obstacle to using logical operations to implement bit operations. The bit operations in μC/OS-II are all implemented using logical operations. The bit segment definition may have different allocation orders for different compilers, but considering that 32-bit high-speed CPUs will not use software to simulate this serial port operation, such programs will only be used in medium- and low-speed microcontrollers without on-chip cache such as 5l, 196/296, MSP430, etc., so the method of using bit segments to operate pins still makes sense. Whether to use logical operations or bit segments for bit operations depends entirely on personal preference. The compilers used in this paper are KeiI C51 V7.03, IARC430 V2.10A and Tasking C96 V5.0.
Previous article:Building an embedded system development environment under RTW
Next article:Design of isolated word speech recognition algorithm based on system on chip SoC
Recommended ReadingLatest update time:2024-11-17 00:50
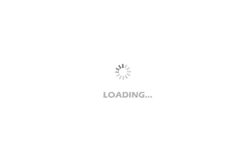
- Popular Resources
- Popular amplifiers
-
Semantic Segmentation for Autonomous Driving: Model Evaluation, Dataset Generation, Viewpoint Comparison, and Real-time Performance
-
Machine Learning and Embedded Computing in Advanced Driver Assistance Systems (ADAS)
-
Intelligent program synthesis framework and key scientific problems for embedded software
-
arm_embedded_machine_learning_design_dummies_guide
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- HuaDa HC32F460 series MCU internal FLASH made into USB flash drive
- PCB wiring and layout and circuit design 268 design specifications
- [ESK32-360 Review] + Construction of development environment
- Several circuit designs that must be understood by microcontrollers
- Several questions about common source amplifier circuits.
- Reliable communications for short-range wireless systems
- I can't read the TMP275 temperature sensor. I use the STM32 I/O to simulate IIC. Please help.
- Understanding GaN Device Thermal Analysis
- 5. Control objects in previous “Control” competitions
- Album of previous competition questions of the National Undergraduate Electronic Design Competition