Five commonly used random number generators include the square median generator, the product median generator, the linear congruential generator, the constant multiplier generator, and the Fibonacci generator. You can ask Baidu for the principle, so I won't introduce it here, but only summarize the code implementation.
Square median generator
clc
clear all
close all
% Call function---parameters can be modified
[x0,u0] = Square_mid_rand(156, 2, 20);
% define function
function [xu] = Square_mid_rand(x0, k, maxIter)
x = []; % Initialize an empty matrix
u = [];
x(1) = x0; % Assignment operation, that is, x0 is placed in the first component of vector x
u(1) = x(1) / 10^(2*k);
for i = 2 : maxIter % loop
x(i) = rem(floor(x(i-1)^2 / 10^k), 10^(2*k)); % Random number iteration generator
u(i) = x(i) / 10^(2*k);
end
% Draw the image
subplot(1,2,1);
hold on; % Maintain the current state.
plot(u(3:end), '*-'); % The data is from the third component of u to the last component, * marks the key point
sTitle = sprintf('Square middle random number generator (x0=%d, k=%d)', x0, k); % format the output string
title(sTitle);
hold off;
subplot(1,2,2);
hold on;
hist(u(3:end));
title('Random number histogram distribution');
hold off;
end
Product Center Generator
clc
clear all
close all
% Call function---parameters can be modified
[x0, u0] = multiply_mid_rand(5167, 3729, 2, 20)
% define function
function [xu] = multiply_mid_rand(x0, x1, k, maxIter)
x = [];
u = [];
x(1) = x0; x(2) = x1;
u(1) = x(1) / 10^(2*k);
for i = 3 : maxIter
x(i) = rem(floor(x(i-1) * x(i-2) / 10^k), 10^(2*k));
u(i) = x(i) / 10^(2*k);
end
hold on;
subplot(1,2,1);
plot(u(3:end), '*-');
sTitle = sprintf('Random number generator for product (x0=%d, x1=%d, k=%d)', x0, x1, k);
title(sTitle);
subplot(1,2,2);
hist(u(3:end));
title('Random number histogram distribution');
hold off;
end
3. Linear congruential generator
clc
clear all
close all
% Call function---parameters can be modified
[x0,u0] = linear_mod_random(5, 5, 9, 5, 20)
% define function
function [x,u] = linear_mod_random(a, c, m, x0, iterMax)
x = [];
u = [];
x(1) = x0;
u(1) = x(1) / m;
for i = 2 : iterMax
x(i) = a * x(i-1) + c;
x(i) = mod(x(i), m);
u(i) = x(i) / m;
end
hold on;
subplot(1,2,1);
plot(x(2:end), '*-');
sTitle = sprintf('Linear congruential random number generator (a=%d, c=%d, m=%d, x0=%d)', a, c, m, x0);
title(sTitle);
subplot(1,2,2);
hist(u(2:end));
title('Random number histogram distribution');
hold off;
end
4. Constant multiplier generator
clc
clear all
close all
% Call function---parameters can be modified
[x0,u0] = con_mid_rand(6983, 2687, 2, 20);
% define function
function [xu] = con_mid_rand(x0, M, k, maxIter)
x = [];
u = [];
x(1) = x0;
u(1) = x(1) / 10^(2*k);
for i = 2 : maxIter
x(i) = rem(floor(x(i-1) * M / 10^k), 10^(2*k));
u(i) = x(i) / 10^(2*k);
end
hold on;
subplot(1,2,1);
plot(u(2:end), 'r*-');
Title = sprintf('Constant multiplier random number generator (x0=%d, M=%d, k=%d)', x0, M, k);
title(Title);
subplot(1,2,2);
hist(u(2:end));
title('Random number histogram distribution');
hold off;
end
5. Fibonacci Generator
clc
clear all
close all
% Call function---parameters can be modified
[x0,u0] = fib_rand(9, 20);
% define function
function [xu] = fib_rand(m, MaxIter)
x = []; modX = [];
u = [];
x(1) = 1; x(2) = 1;
for i = 3 : MaxIter
x(i) = x(i-1) + x(i-2);
modX(i) = rem(x(i), m);
u(i) = modX(i) / m;
end
hold on;
subplot(1,2,1);
plot(modX(3:end), 'r*-');
sTitle = sprintf('Fibonacci random number generator (m=%d)',m);
title(sTitle);
subplot(1,2,2);
hist(u(3:end));
title('Random number histogram distribution');
hold off;
end
The above are five random number generators simply implemented using MATLAB. In the future, we will add the performance test of the combination generator and the generated random number sequence.
Previous article:Design and Implementation of Function Signal Generator
Next article:Function signal generator based on Multisim - square wave, triangle wave, sine wave
Recommended ReadingLatest update time:2024-11-16 22:48
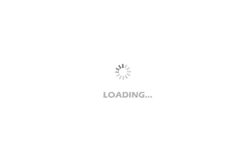
- Keysight Technologies Helps Samsung Electronics Successfully Validate FiRa® 2.0 Safe Distance Measurement Test Case
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Seizing the Opportunities in the Chinese Application Market: NI's Challenges and Answers
- Tektronix Launches Breakthrough Power Measurement Tools to Accelerate Innovation as Global Electrification Accelerates
- Not all oscilloscopes are created equal: Why ADCs and low noise floor matter
- Enable TekHSI high-speed interface function to accelerate the remote transmission of waveform data
- How to measure the quality of soft start thyristor
- How to use a multimeter to judge whether a soft starter is good or bad
- What are the advantages and disadvantages of non-contact temperature sensors?
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- i.MX6ULL Embedded Linux Development——by DDZZ669
- The three-phase high current (30A~40A) of the motor is connected through the PCB, and the heat dissipation hole blind hole problem
- EEWORLD University ---- Key Isolation Gate Driver Specifications
- RTT Studio is an open source and free one-stop development tool for embedded systems. It’s really great to use it!
- PCB Design and Wiring Skills Album
- Help with information about injection locking
- [Zero-knowledge esp8266 tutorial] Quick start 30 rc-switch wireless transceiver module usage
- Where can I download the Datasheet from the official website of HuaDa Semiconductor?
- [BearPi-HM Nano, play with Hongmeng's "touch and pay" function] Part 3: Is the OLED screen lit up?
- Fluke always has an infrared thermal imager that suits your needs! Participate in the event to win gifts and start