1.1 Introduction to Touch Buttons
The touch button is one of the many buttons. It is actually equivalent to an electronic switch. Just press the button lightly to turn on the switch, and release it to turn off the switch. Due to the characteristics of micro switches and the advantages of small size and light weight, the touch button has been widely used in household appliances. The application scenarios include: TV buttons, remote control buttons, computer buttons, keyboard buttons, display buttons, lighting buttons, etc., as shown in the figure below.
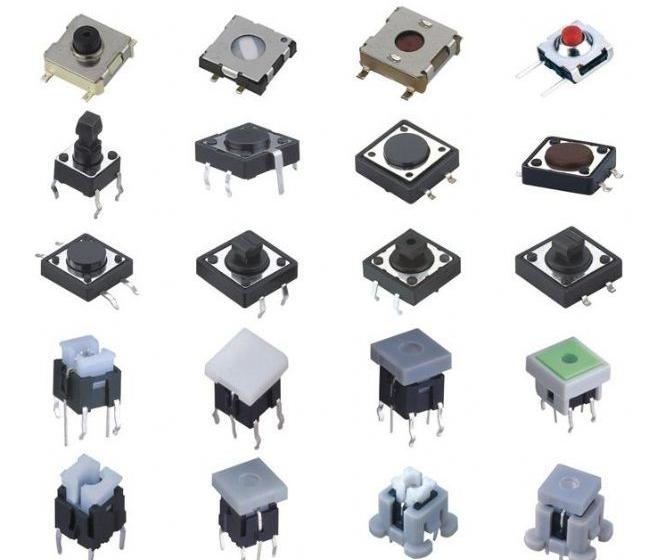
1.2 Principle of Touch Button
Taking a four-pin touch button as an example, the internal structure of the four-pin touch switch is shown in the figure below.
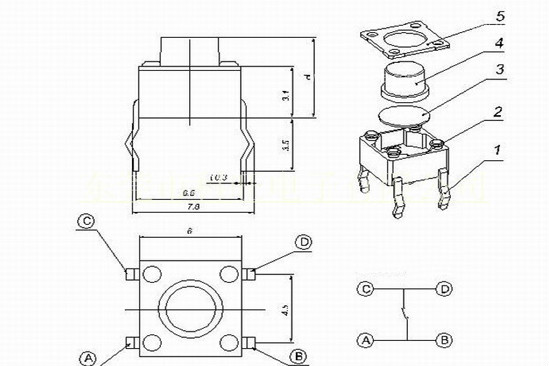
The working principle of this switch is actually similar to that of an ordinary push button switch . It is composed of a normally open contact and a normally closed contact. In a four-pin touch switch, the function of the normally open contact is that when pressure is applied to the normally open contact, the circuit is connected; when the pressure is removed, it returns to the original normally closed contact, which is the so-called disconnection. This pressure is the action of using our hands to open and close the button.
2.1 Introduction to Interrupts
Due to some random event (external or internal), the microcontroller temporarily interrupts the running program and executes a special service program (interrupt service subroutine or interrupt handler) to handle the event. After the event is handled, it returns to the interrupted program to continue execution. This process is called an interrupt, and the interrupt source is called an interrupt source. For example, when watching TV, the doorbell rings suddenly, then the doorbell ringing is equivalent to the interrupt source. Some interrupts can also be interrupted by other high-priority interrupts, so this situation is called interrupt nesting. Since there are many interrupts in the microcontroller, we mainly talk about external interrupts here.
Each GPIO pin of STM32F103 can be used as an interrupt input port for external interrupts. Each interrupt has a status bit, and each interrupt/event has independent trigger and mask settings. The external interrupt lines of STM32 and their corresponding events are:
(1) Line 0~15: corresponding to the input interrupt of the external IO port;
(2) Line 16: connected to PVD output;
(3) Line 17: connected to the RTC alarm event;
(4) Line 18: connected to the USB wake-up event;
(5) Line 19: Connected to Ethernet wake-up event.
Here we use the GPIO input interrupts of Line 0 to 15, where 0 to 15 correspond to each group of GPIO pins 0 to 15. The following table shows the interrupt lines corresponding to the pins and the corresponding interrupt service function names.
Pins
|
Interrupt flag
|
Interrupt handling function
|
PA0~PG0
|
EXIT0
|
EXTI0_IRQHandler
|
PA1~PG1
|
EXIT1
|
EXTI1_IRQHandler
|
PA2~PG2
|
EXIT2
|
EXTI2_IRQHandler
|
PA3~PG3
|
EXIT3
|
EXTI3_IRQHandler
|
PA4~PG4
|
EXIT4
|
EXTI4_IRQHandler
|
PA5~PG5
|
EXIT5
|
EXTI9_5_IRQHandler
|
PA6~PG6
|
EXIT6
|
PA7~PG7
|
EXIT7
|
PA8~PG8
|
EXIT8
|
PA9~PG9
|
EXIT9
|
PA10~PG10
|
EXIT10
|
EXTI15_10_IRQHandler
|
PA11~PG11
|
EXIT11
|
PA12~PG12
|
EXIT12
|
PA13~PG13
|
EXIT13
|
PA14~PG14
|
EXIT14
|
PA15~PG15
|
EXIT15
|
2.2 Interrupt Priority Management
When multiple interrupts are configured, what should we do if multiple interrupts are triggered at the same time? Which one will be executed first and which one will be executed later? In STM32, there is a mechanism specifically used to handle interrupt priority issues, called the interrupt grouping mechanism. The grouping is configured in the register SCB->AIRCR. See the table below.
Group
|
AIRCR[10:8]
|
IP bit[7:4] allocation
|
Allocation results
|
0
|
111
|
0:4
|
0-bit preemption priority, 4-bit response priority
|
1
|
110
|
1:3
|
1-bit preemption priority, 3-bit response priority
|
2
|
101
|
2:2
|
2-bit preemption priority, 2-bit response priority
|
3
|
100
|
3:1
|
3-bit preemption priority, 1-bit response priority
|
4
|
011
|
4:0
|
4-bit preemption priority, 0-bit response priority
|
Group 0 is 4 bits used to set the response priority, 2^4=16 bits are all response priority;
Group 1 is divided into (2^1) two preemption priorities, and there are (2^3) eight response priorities in these two preemption priorities, (2^1) * (2^3) = 16;
Group 2 is divided into (2^2) four preemption priorities, and each of these four preemption priorities has (2^2) four response priorities, (2^2) * (2^2) = 16;
Group 3 is divided into eight preemption priorities (2^3), and there are two response priorities (2^1) in these eight preemption priorities, (2^3)*(2^1) = 16;
Group 4 is divided into (2^4) sixteen, all with preemptive priority (2^4) = 16;
The STM32 interrupt grouping often uses the code "NVIC_PriorityGroupConfig", as shown in the following example:
NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
This sample code sets NVIC interrupt group 2, which is a 2-bit preemption priority and a 2-bit response priority. In other words, four preemption priorities can be configured, and four response priorities can be configured in these four preemption priorities.
So what is preemption priority and what is response priority? The main differences are as follows:
(1) Preemption priority. An interrupt with a high preemption priority can interrupt an interrupt with a low preemption priority;
(2) Response priority. When preempting interrupts with the same priority, the one with the higher response priority will be executed first.
2.3 External interrupt triggering method
There are three main ways for STM32 to trigger external interrupts:
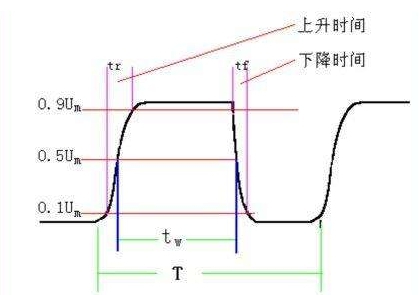
Rising edge triggering refers to the process in which the level changes from a low level ("0") to a high level ("1"), which corresponds to the rising time part in the above figure.
Falling edge triggering refers to the process in which the level changes from a high level ("1") to a low level ("0"), which corresponds to the falling time part in the above figure.
Rising edge or falling edge trigger means that both (1) and (2) will trigger an interrupt.
2.4 Commonly used registers for external interrupts
The commonly used registers for STM32 to configure external interrupts are as follows:
(1) Rising edge trigger selection register (EXTI_RTSR): This register is used to configure whether the interrupt on the interrupt line x allows rising edge triggering.
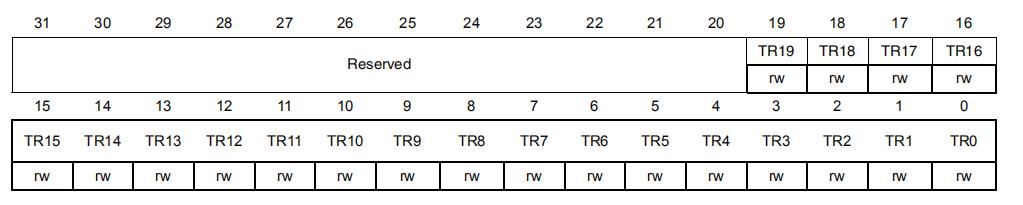
TRx: refers to the rising edge trigger event configuration bit of interrupt line x, where 0 means prohibiting the input line rising edge trigger, and 1 means allowing the input line rising edge trigger.
- Falling edge trigger selection register (EXTI_FTSR), which is used to configure whether the interrupt on the interrupt line x allows falling edge triggering.

TRx: refers to the falling edge trigger event configuration bit of interrupt line x, where 0 means prohibiting the falling edge trigger of the input line, and 1 means allowing the falling edge trigger of the input line.
2.5 Interruption experiment
The content of this experiment is to use the left joystick button on the remote control to control the indicator light on the remote control. Here, the GPIO of the left joystick button is configured to trigger the falling edge of the external interrupt. Once triggered, the state of the remote control indicator light is reversed, that is, the original on is off and the original off is on.
The left joystick button of the remote control is shown in the figure below.
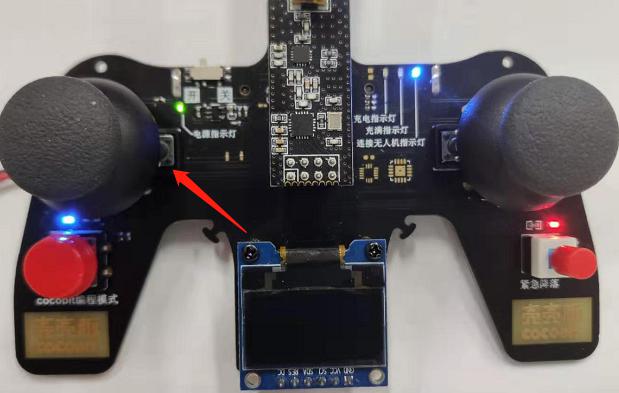
Looking at the schematic diagram, we can see that the button on the left joystick corresponds to PB9, as shown in the figure below.
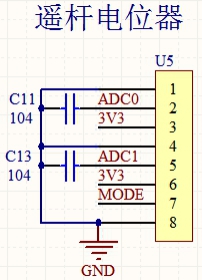
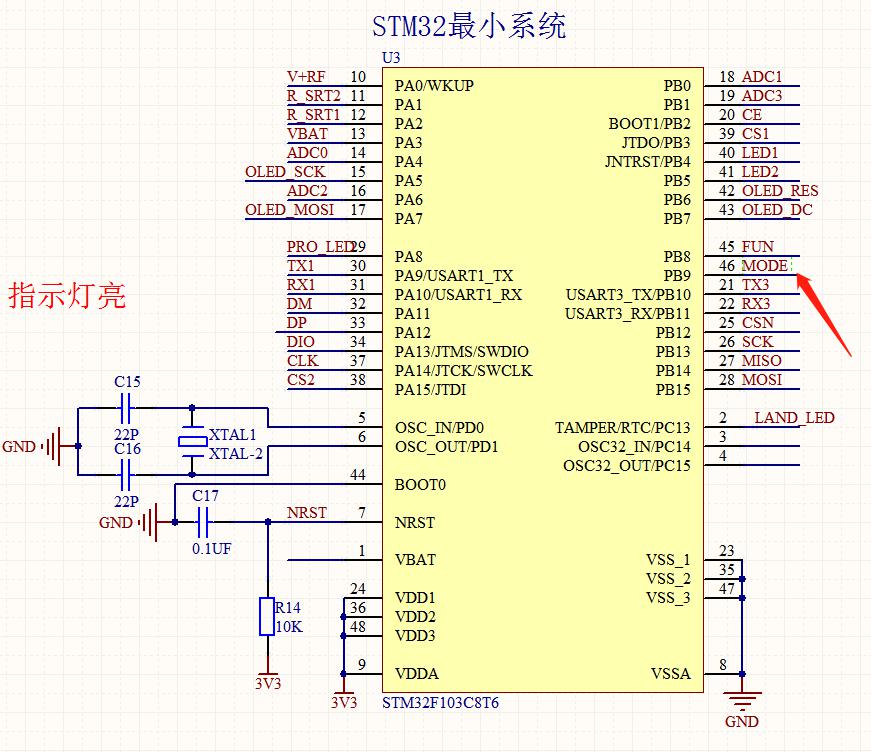
For LED configuration, please refer to the "GPIO" section of the remote control development basic tutorial. The overall idea of writing code is shown in the following table:
Code ideas
1
|
Pin Configuration
|
1. Define the structure;
2. Enable the clock;
3. Filling structure;
4. Load the structure.
|
2
|
External interruption placement
|
1. Define the structure;
2. Enable the clock;
3. Configure the interrupt line;
4. Filling structure;
5. Load the structure.
|
3
|
Interrupt Management Configuration
|
1. Define the structure;
2. Filling structure;
3. Load the structure.
|
4
|
Logical Processing
|
1. Interrupt function logic.
|
According to the code idea, write the code (by calling the official library), and the code for configuring the external interrupt for the left button of the joystick is as shown in the figure below:
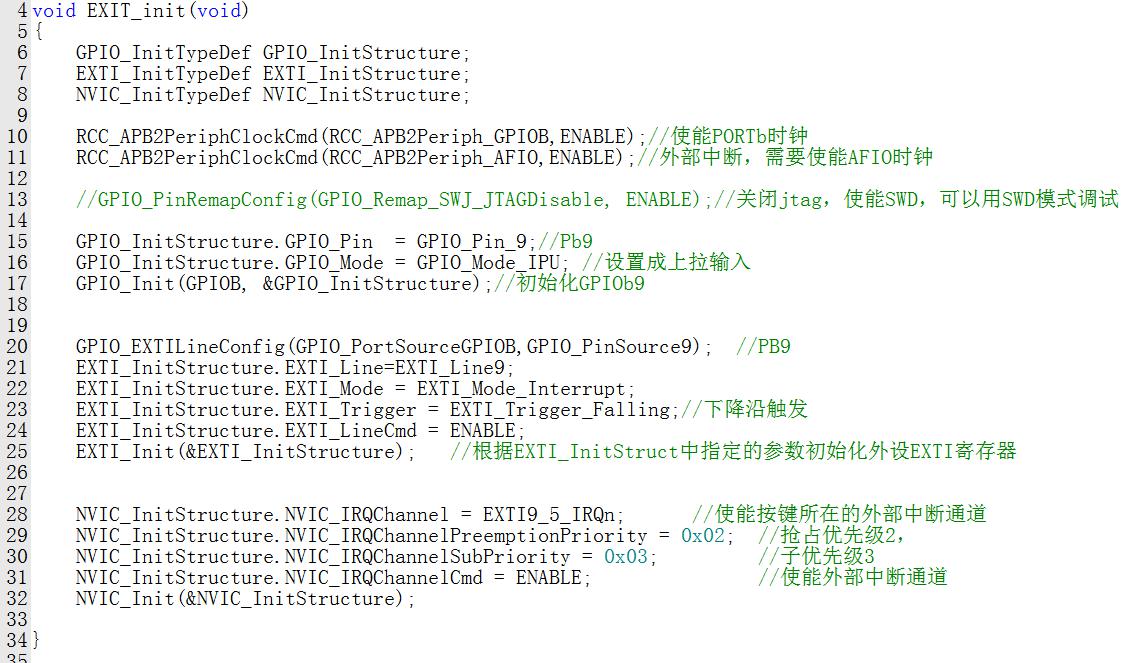
The logical processing of the interrupt service function is shown in the figure below.
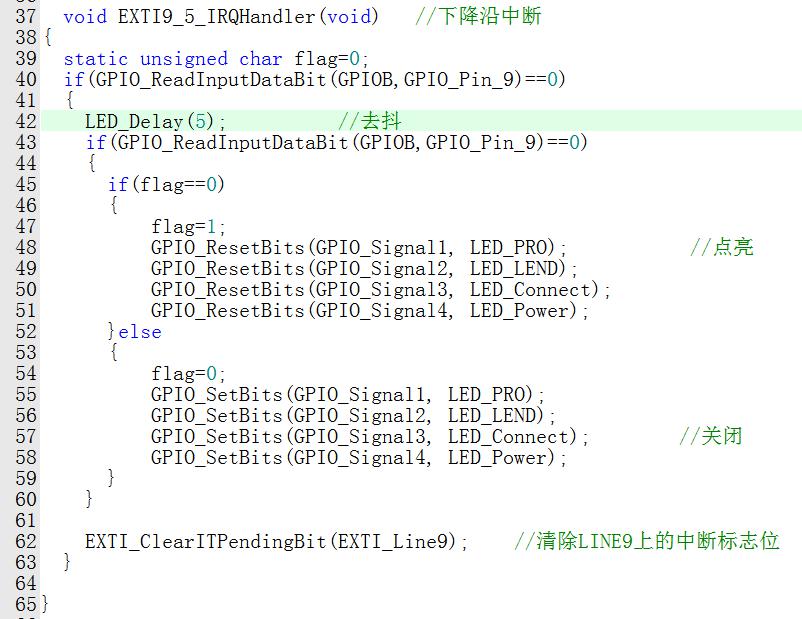
Save, compile, and download. Press the left button continuously and you can see the four LED indicators on the remote controller turn off and on, as shown in the figure below.
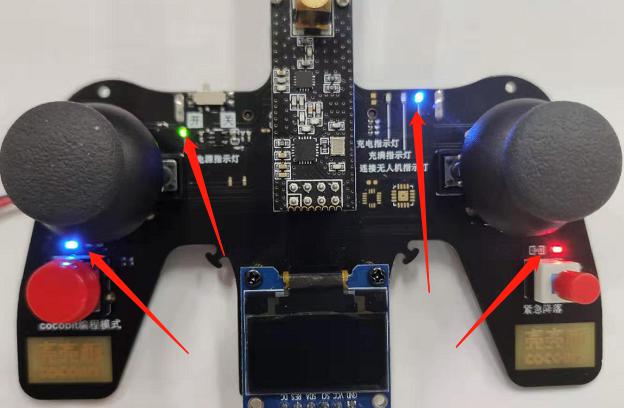