Let's talk about this familiar yet unfamiliar GPIO.
What is GPIO?
Literally, GPIO=General Purpose Input Output. Sometimes it is referred to as "IO port". General, that is, it is a panacea, it can do anything. Input and output, that is, it can be used as both an input port and an output port. A port is a pin on a component. How to use it? Write software control.
In summary: GPIO is a pin on a chip that can do anything.
After talking so much, I believe that people who don't understand are still confused. Let's look at the case to see how GPIO is used. As for concepts such as pull-up, pull-down, floating, high impedance, open drain, push-pull, etc., you can slowly figure it out later.
Simple use of GPIO
Output control signal
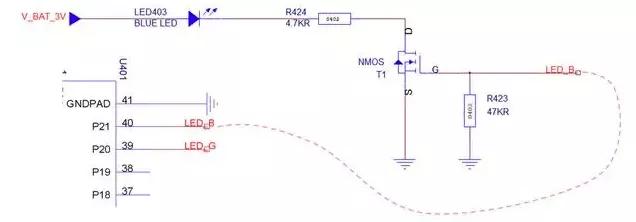
GPIO controls the switch of LED lights.
GPIO is used for switch control, which is the most common application scenario.
As shown in the figure above, when the GPIO port P21 outputs 1, LED403 lights up, and when it outputs 0 or no output, LED403 goes out.
How is the GPIO port controlled? Through software code. When you need to turn on the light, call the function of pulling the GPIO port high, and when you need to turn off the light, call the function of pulling the GPIO low to achieve control. The operation of the function eventually becomes writing data to the hardware register of this GPIO, and the state of the hardware will change with the change of the register data.
The hardware register here can be understood as an electronic switch, just like you tell the nanny at home to say "Go and turn off the lights in the living room", and he goes over and presses the light switch, and then the lights go out. The action of the instruction you issued is equivalent to calling the function of GPIO operation, and the action of the nanny pressing the switch is equivalent to the function configuring the register.
Of course, you can also press the switch directly (directly operate the register). Although this approach works, it does not meet the specifications in code design. It is easy to cause misoperation in subsequent modifications. In actual operation, it is necessary to pre-initialize, configure GPIO parameters, establish register interfaces for other processes to call, and other software operations, which will not be described in detail here.
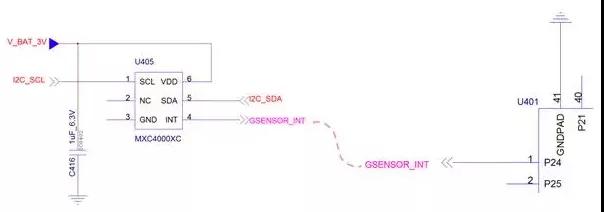
Input interrupt signal
The gravity sensor outputs an interrupt signal to the MCU's GPIO port
G-sensor, also called a gravity sensor/acceleration sensor/motion sensor, to detect whether the device is moving. The pedometer of the Bluetooth bracelet we usually use is mainly calculated based on the motion data sampled by the G-sensor.
When the device is not moving, the G-sensor and the MCU are both in sleep mode to save power. When
the device moves, the G-sensor senses it and wakes up, and sends a high-level signal to the interrupt port (GSENSOR_INT). When the MCU senses that the level of this interrupt port changes from low to high, it exits sleep and starts normal operation.
Then the MCU reads the data in the G-sensor through the I2C data interface.
How to understand interrupts? You are sleeping, and suddenly someone comes to you, and he has to shake you awake first. This is to interrupt your sleep and wake you up from sleep (as in the above example).
Similarly, if you are watching a movie and suddenly your phone rings, you see that it is your girlfriend calling, you have to pause the movie, keep the current playback position of the movie, and then answer your girlfriend's call. After answering the phone, continue playing from the previous playback position.
This phone call is the interrupt signal, saving the movie position is the state before the interrupt response is pushed into the stack, the process of answering the phone is the interrupt service program, and continuing to play after hanging up the phone is the interrupt state popped out of the stack.
Some people may say, why is it redundant, can't the G-sensor send the data directly to the MCU? This is because I2C can only be initiated by the master device to actively initiate a data transmission request, and the slave device cannot actively send data (it can only allow the master device to come and read the data). For the content of the I2C protocol, please see the relevant article.
Any device with an I2C interface and continuous operation needs to have an interrupt output to tell the host "I have prepared the data, please come and take it away quickly." When
using GPIO as an interrupt, you also need to pay special attention to one thing: if you choose this interrupt port to wake up the system, you must check the chip specification to see clearly whether the selected interrupt port can wake up the system?
For most microcontrollers, almost every interrupt port can wake up the system, but for high-frequency processors, such as mobile phones and tablets, not all GPIOs can be configured as interrupts, and not all interrupts can wake up the system.
If you select an interrupt port that cannot wake up the system for the above example, once the MCU enters sleep mode, the peripheral will become ineffective
.
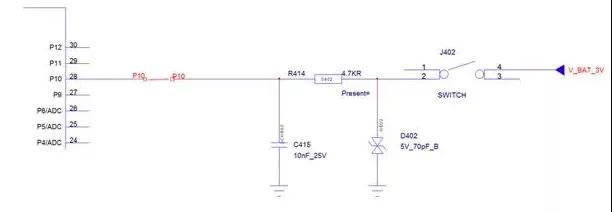
GPIO for key detection
Strictly speaking, a key is also an interrupt. The default state of the GPIO port is low level. After the key is pressed, it is pulled to a high level. At this time, the system can detect the interrupt and determine that the key is pressed.
When the key is released, the GPIO port detects that the voltage returns to a low level, and it is determined that the key is released. This practice is a common practice on microcontrollers. On smarter hardware platforms, there is often an independent hardware key interface (non-GPIO port). A key controller is added inside the chip to implement key debounce, double-click and long-press judgment through hardware.
For a microcontroller, once it is triggered by a key, the program starts running inside, reading the key status every few milliseconds to determine whether the key is released. The functions of debounce, double-click and long-press are implemented through software.
The capacitor in the figure is used to filter out external interference to avoid false triggering, and at the same time plays a certain role in key debounce. The TVS tube in the figure is to prevent static electricity from entering the CPU.
Some people may ask, if the key is pressed, it is pressed, why does it jitter?
Because the buttons are mechanical, there will be a slight jitter of contact-disconnection-re-contact in the microsecond time period when the two metal sheets touch. The jitter will not disappear until the two metal sheets are firmly in contact. The so-called button de-jitter is to eliminate the abnormal state of contact and disconnection through delay.
If there are not enough GPIO ports, but multiple button detection is required, the buttons can also be configured as ADC, and different voltages are generated by different buttons to detect different key values using one ADC port. This method is usually used to detect the three buttons on the 3.5mm wired headset of the mobile phone.
Advanced applications of GPIO
In addition to simple input and output, GPIO can also perform some relatively complex operations, such as simulating I2C or SPI data lines, ADC voltage detection, and outputting PWM waveforms.
Some of these functions can be directly configured as hardware interfaces, or waveforms can be simulated by software.
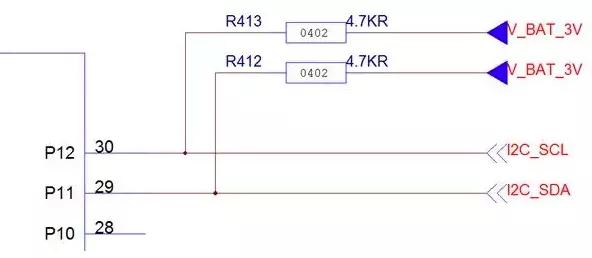
Used as I2C interface
GPIO used as I2C data bus
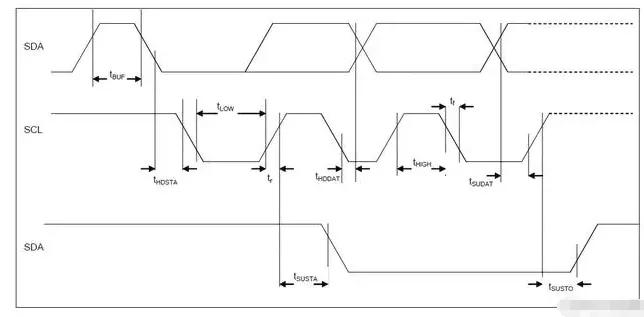
I2C timing diagram
I2C is the most commonly used data transmission bus in intelligent hardware circuits. It only needs 2 wires to mount multiple slave devices. It can transmit in both directions with a maximum speed of 400Kbps, which is very suitable for transmitting control instructions and small amounts of data.
The G-sensor sensors, light distance sensors, capacitive touch screens, LED light controllers, camera control commands, etc. that people usually use are almost all I2C interfaces.
Using the GPIO port as I2C is the most common way for GPIO to transmit data. If the chip has its own I2C controller, you can directly configure the GPIO to switch to the hardware I2C. For example, almost all single-chip microcomputers can do this.
If the I2C interface inside the chip is not enough, you can also use the software to control the GPIO port to pull high and low to simulate the waveform and timing of I2C, and it can still be used as I2C.
The same method of simulating data lines can also be used to simulate SPI with GPIO. As long as it is a low-speed synchronous data line with a clock, it can be simulated with the GPIO port.
However, the GPIO port cannot be used to simulate the UART serial port. Because UART has no clock line, it needs to output the waveform very accurately at the agreed time interval. The software timer is inaccurate and the hardware timer takes up a lot of system resources, so it is difficult to achieve.
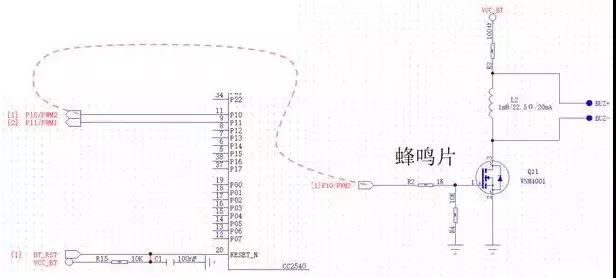
PWM output
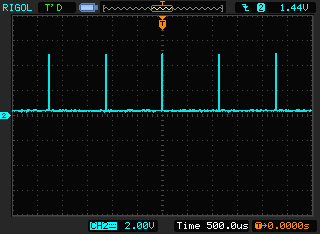
GPIO outputs PWM wave to control buzzer The PWM wave output by GPIO port
with different duty cycles
is the same as that used as I2C. By controlling the GPIO port to pull up and down regularly, the PWM waveform can be output.
As shown in the figure above, the external boost circuit is controlled by PWM to drive the buzzer to make a sound. PWM can also be used to control the dimming of LED lights, change the duty cycle of PWM output, and adjust the brightness of the light.
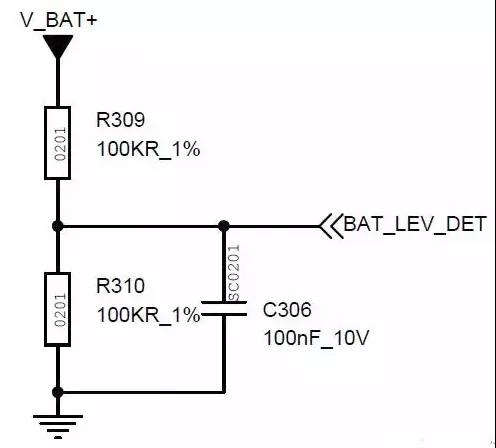
ADC sampling
GPIO is used for ADC sampling and collecting battery voltage
After the battery is divided, it is sampled by
ADC, Analog-to-Digital Converter, which converts analog signals into digital signals. ADC has a wide range of applications, such as sampling of microphone audio data, sampling of voltage and current signals, and quantization of data output by analog sensors.
Limited by accuracy, range, sampling speed, etc., GPIO ADC is generally not used for too complex applications, and most of the time it only collects voltage.
As shown in the figure above, the GPIO port is configured to ADC mode to collect battery voltage for battery power display. This method is only suitable for simple battery voltage display. If you want to do battery power management with 1% accuracy like a smartphone, you need to add a higher-precision ADC and battery compensation algorithm. The
most common problems encountered when GPIO is used as ADC are:
1. Not all GPIO ports can be used as ADC, so be sure to read the specification carefully!
2. ADC has voltage domain restrictions, and 3V-powered ADC cannot measure voltages exceeding 3V. For example, in the first figure above, the MCU is powered by a 3V battery. At this time, the power supply voltage of GPIO/ADC is 3V, and the maximum range is also 3V, which can measure the battery voltage. In the second picture, the lithium-ion battery voltage is 4.2V, the MCU power supply is 3V, and the GPIO/ADC working voltage is also 3V, so it cannot measure such a high voltage. The voltages measured beyond the range are the same. Therefore, using resistor voltage divider, the 4.2V battery voltage is halved to 2.1V for use with the 3V range ADC.
|